In my previous article we learned about helper classes that BRIDGEs the way to access HTML DOM. Each class has their own methods to access HTML elements.
So here we continue hunting for a few more such helper classes to try, and to do some practical exercises to access HTML in Silverlight.
Let us try to manipulate the HTML element from Silverlight code behind.
What we are trying to do here is to access HTNL element and manipulate them, define property similar to CSS way we do in HTML.
We will re-work with project we created in last article.
Step 1: Add a Silverlight user control and name it "PlayWithHTMLElement.xaml"
Step 2: Create 4 buttons to perform respective manipulations.
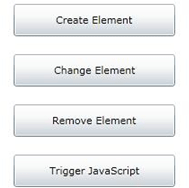
Step 3: Now let's start writing a handler for each button.
Create Element: On the click of a button we will create a HTML element.
private void create_Click(object sender, RoutedEventArgs e)
{
HtmlElement element = HtmlPage.Document.CreateElement("p");
element.Id = "paragraph";
element.SetProperty("innerHTML", "This is a new element.Click to change its backgroud color.");
HtmlPage.Document.Body.AppendChild(element);
element.SetStyleAttribute("border", "solid 1px black");
element.SetStyleAttribute("margin", "10px");
element.SetStyleAttribute("padding", "10px");
element.AttachEvent("onclick", paragraph_Click);
}
CreateElement actually takes a tag name of browser element to create, so you can see "p" is a html tag for creating paragraphs. Here you can create any other valid HTML tag
HtmlElement element = HtmlPage.Document.CreateElement("p");
element.Id = "paragraph" == define element ID
element.SetProperty == You give name to element and its value
HtmlPage.Document.Body.AppendChild(element)= This adds the created element to HTML page.
To create CSS like look, we can use
element.SetStyleAttribute("border", "solid 1px black");
element.SetStyleAttribute("margin", "10px");
element.SetStyleAttribute("padding", "10px");
What if we already have a css class defined in HTML and we want to access it from code behind? In that case we will use the property called CssClass.
<style type="text/css">
.highlightedParagraph
{
color: White;
border: solid 1px black;
background-color: Lime;
}
element.CssClass = "highlightedParagraph"
You can also trigger an event for HTML element:
element.AttachEvent("onclick", paragraph_Click);
private void paragraph_Click(object sender, HtmlEventArgs e)
{
HtmlElement element = (HtmlElement)sender;
element.SetProperty("innerHTML", "You clicked this HTML element, and Silverlight handled it.");
element.SetStyleAttribute("background", "#00ff00");
}
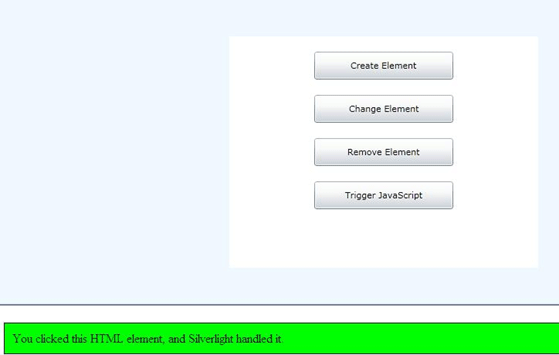
Similarty to Update the element
private void change_Click(object sender, RoutedEventArgs e)
{
HtmlElement element = HtmlPage.Document.GetElementById("paragraph");
if (element == null) return;
element.SetProperty("innerHTML",
"This HTML paragraph has been updated by Silverlight.");
element.SetStyleAttribute("background", "white");
}
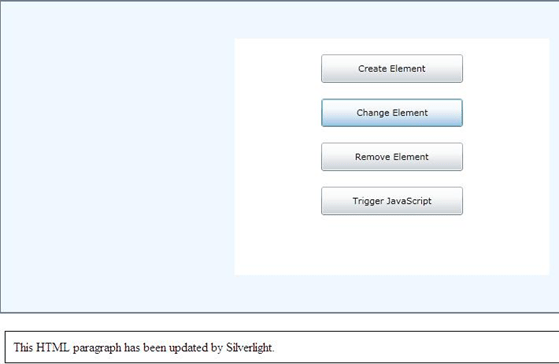
Here we are finding the element by its ID
HtmlPage.Document.GetElementById("paragraph");
To remove any element from HTML:
private void remove_Click(object sender, RoutedEventArgs e)
{
HtmlElement element = HtmlPage.Document.GetElementById("paragraph");
if (element == null) return;
element.Parent.RemoveChild(element);
}
Ok, so now it's time for something big, which is calling a Javascript function from Silverlight.
Say we have the following javascript and calling any js file is a common thing in ASP.Net.
<script type="text/javascript">
function changeParagraph(newText) {
var element = document.getElementById("paragraph");
element.innerHTML = newText;
}
</script>
Now where can you write all your javascipt code? Well you can write them in the HTML or ASPX file which hosts the Silverlight application.
private void trigger_Click(object sender, RoutedEventArgs e)
{
HtmlElement element = HtmlPage.Document.GetElementById("paragraph");
if (element == null) return;
ScriptObject script = (ScriptObject)HtmlPage.Window.GetProperty("changeParagraph");
script.InvokeSelf("Changed through JavaScript.");
}
Let us recall about ScriptObject and what does it do; it represents a JavaScript function that's defined in the page, and allows you to invoke the function from your Silverlight application. So here we are invoking a javascript function from Silverlight.
InvokeSelf : To invoke Javascript function.
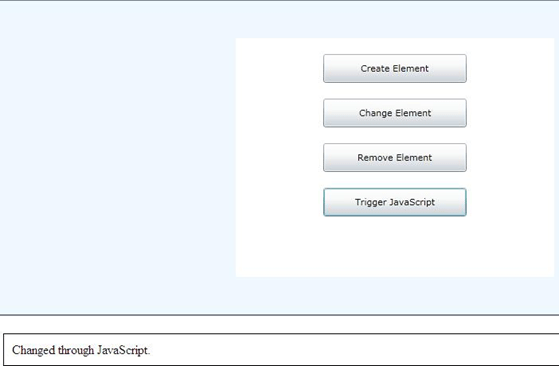
What about when we wish to get a return value from Javascript, such as below.
function sendText() {
return "Hi from HTML Javascript!";
}
It's pretty simple.
private void return_Click(object sender, RoutedEventArgs e)
{
string obj = HtmlPage.Window.Invoke("sendText", null) as string;
MessageBox.Show(obj);
}
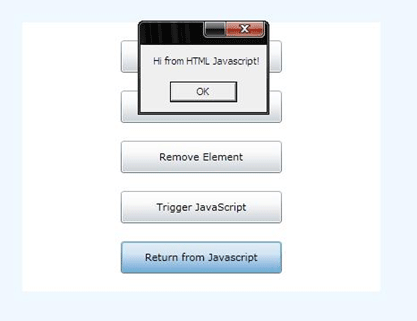
That's all in this article, hope you enjoyed
Cheers...