This article has been excerpted from book "Graphics Programming with GDI+".
The PathGradientBrush object is used to fill a graphics path with a gradient. We can specify the center and boundary colors of a path.
The CenterColor and SurroundColors properties are used to specify the center and boundary colors. Listing 9.28 uses the CenterColor and SurroundColors properties; it sets the center color of the path to red and the surrounding color to green.
LISTING 9.28: Blending using PathGradientBrush
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace BlendingUsingPathGradientBrush
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Paint(object sender, PaintEventArgs e)
{
Graphics g = this.CreateGraphics();
g.Clear(this.BackColor);
// Create Blend object
Blend blend = new Blend();
// Create point and positions arrays
float[] factArray = { 0.0f, 0.3f, 0.5f, 1.0f };
float[] posArray = { 0.0f, 0.2f, 0.6f, 1.0f };
// Set Factors and Positions properties of Blend
blend.Factors = factArray;
blend.Positions = posArray;
// Set smoothing mode of Graphics object
g.SmoothingMode = SmoothingMode.AntiAlias;
// Create path and add a rectangle
GraphicsPath path = new GraphicsPath();
Rectangle rect = new Rectangle(10, 20, 200, 200);
path.AddRectangle(rect);
// Create path gradient brush
PathGradientBrush rgBrush =
new PathGradientBrush(path);
// Set Blend and FocusScales properties
rgBrush.Blend = blend;
rgBrush.FocusScales = new PointF(0.6f, 0.2f);
Color[] colors = { Color.Green };
// Set CenterColor and SurroundColors properties
rgBrush.CenterColor = Color.Red;
rgBrush.SurroundColors = colors;
g.FillEllipse(rgBrush, rect);
// Dispose of object
g.Dispose();
}
}
}
If you run the code from Listing 9.28, you will see that the focus is the center of the ellipse and there is scattering in a faded color toward the boundary of the ellipse. The center is red, and the border is green (see Figure 9.37).
The FocusScales property changes the focus point for the gradient falloff. The following code snipped sets the FocusScales property.
rgBrush.FocusScales = new PointF(0.6f, 0.2f);
After FocusScales is set the color of the ellipse changes from the center of the ellipse to a rectangle. Figure 9.38 shows the new output.
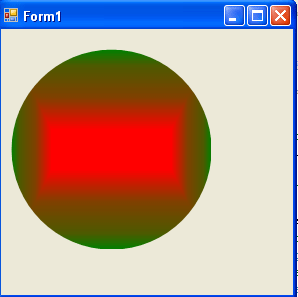
FIGURE 9.37: Blending using PathGradientBrush
We can even specify multiple surrounding colors. For example, we can create an array of different colors and use them for the SurroundedColors property of the brush. To do so, we replace the following line of Listing 9.28.
Color[] colors = { Color.Green };
with the following code snipped:
Color[] colors =
{ Color.Green, Color.Blue,
Color.Red, Color.Yellow};
rgBrush.SurroundColors = colors;
If you add this code to the application, you will see a totally different output. As Figure 9.39 shows, the new ellipse has four different boundary colors.
Like LinearGradientBrush, the PathGradientBrush class provides Blend and InterpolationColors properties. Listing 9.29 shows the InterpolationColors property in use.
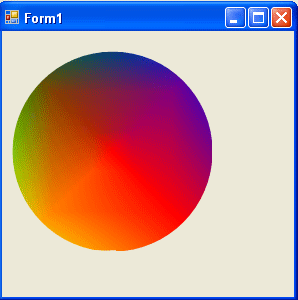
FIGURE 9.39: Blending multiple colors
LISTING 9.29: Using the InterpolationColors property of PathGradientBrush
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace BlendingUsingPathGradientBrush
{
public partial class Form1 : Form
{
public Form1()
{ InitializeComponent();
}
private void Form1_Paint(object sender, PaintEventArgs e)
{
Graphics g = this.CreateGraphics();
g.Clear(this.BackColor);
// Create color and points arrays
Color[] clrArray =
{
Color.Red, Color.Blue, Color.Green,
Color.Pink, Color.Yellow,
Color.DarkTurquoise};
float[] posArray =
{
0.0f, 0.2f, 0.4f, 0.6f, 0.8f, 1.0f};
// Create a ColorBlend object and set its Colors and Positions properties
ColorBlend colorBlend = new ColorBlend();
colorBlend.Colors = clrArray;
colorBlend.Positions = posArray;
// Set smoothing mode of Graphics object
g.SmoothingMode = SmoothingMode.AntiAlias;
// Create a graphics path and add a rectangle
GraphicsPath path = new GraphicsPath();
Rectangle rect = new Rectangle(10, 20, 200, 200);
path.AddRectangle(rect);
// Create a path gradient brush
PathGradientBrush rgBrush =
new PathGradientBrush(path);
// Set Interpolation colors and focus scales
rgBrush.InterpolationColors = colorBlend;
rgBrush.FocusScales = new PointF(0.6f, 0.2f);
Color[] colors = { Color.Green };
// Set center and surrounding colors
rgBrush.CenterColor = Color.Red;
rgBrush.SurroundColors = colors;
// Draw ellipse
g.FillEllipse(rgBrush, rect);
// Dispose of object
g.Dispose();
}
}
}
Figure 9.40 shows the output from Listing 9.29.
You can even apply blending on a path gradient brush using the Blend property. Listing 9.30 creates a Blend object and sets the Blend property of the brush.
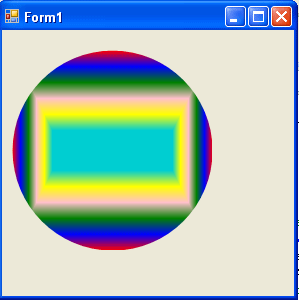
FIGURE 9.40: Using the InterpolationColors property of PathGradeintBrush
LISTING 9.30: Using the Blend property of PathGradientBrush
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace BlendingUsingPathGradientBrush
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Paint(object sender, PaintEventArgs e)
{
Graphics g = this.CreateGraphics();
g.Clear(this.BackColor);
// Create Blend object
Blend blend = new Blend();
// Create point and position arrays
float[] factArray = { 0.0f, 0.3f, 0.5f, 1.0f };
float[] posArray = { 0.0f, 0.2f, 0.6f, 1.0f };
// Set Factors and Positions properties of Blend
blend.Factors = factArray;
blend.Positions = posArray;
// Set smoothing mode of Graphics object
g.SmoothingMode = SmoothingMode.AntiAlias;
// Create path and add a rectangle
GraphicsPath path = new GraphicsPath();
Rectangle rect = new Rectangle(10, 20, 200, 200);
path.AddRectangle(rect);
// Create path gradient brush
PathGradientBrush rgBrush =
new PathGradientBrush(path);
// Set Blend and FocusScales properties
rgBrush.Blend = blend;
rgBrush.FocusScales = new PointF(0.6f, 0.2f);
Color[] colors =
{
Color.Green, Color.Blue,
Color.Red, Color.Yellow
};
// Set CenterColor and SurroundColors
rgBrush.CenterColor = Color.Red;
rgBrush.SurroundColors = colors;
g.FillEllipse(rgBrush, rect);
// Dispose of object
g.Dispose();
}
}
}
Figure 9.41 shows the output from Listing 9.30. Blending is done with four different colors.
Just as with LinearGradientBrush, you can use the SetBlendTriangularShape methods with PathGradientBrush.
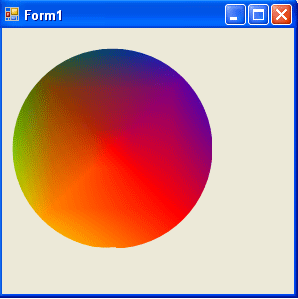
FIGURE 9.41: Multicolor blending using PathGradientBrush
Conclusion
Hope the article would have helped you in understanding Blending Using PathGradientBrush Objects in GDI+. Read other articles on GDI+ on the website.
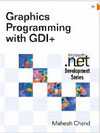 |
This book teaches .NET developers how to work with GDI+ as they develop applications that include graphics, or that interact with monitors or printers. It begins by explaining the difference between GDI and GDI+, and covering the basic concepts of graphics programming in Windows. |