ASP.Net Silverlight control allows passing startup parameters through InitParamaters property. You can specify the parameters as commas separated key-value pair string as follows in aspx page
<asp:Silverlight ID="Xaml1" runat="server" InitParameters="key1=value1,key2=value2" Source="~/ClientBin/InitParamSilverlight.xap" MinimumVersion="2.0.31005.0" Width="100%" Height="100%" />
OR
Declare the initParams param on the Silverlight plug-in and pass in a comma-delimited set of key-value pair tokens as follows in html
<object data="data:application/x-silverlight-2," type="application/x-silverlight-2" width="100%" height="100%">
<param name="source" value="ClientBin/InitParamSilverlight.xap"/>
<param name="onerror" value="onSilverlightError" />
<param name="background" value="white" />
<param name="minRuntimeVersion" value="2.0.31005.0" />
<param name="autoUpgrade" value="true" />
<param name="initParams" value="key1=value1,key2=value2" />
<a href="http://go.microsoft.com/fwlink/?LinkID=124807" style="text-decoration: none;">
<img src="http://go.microsoft.com/fwlink/?LinkId=108181" alt="Get Microsoft Silverlight" style="border-style: none"/>
</a>
</object>
Extract Parameters
Extract the initialization parameters using StartupEventArgs.InitParams property in the Application's Startup
event handler.
private void Application_Startup(object sender, StartupEventArgs e)
{
if (e.InitParams != null)
{
foreach (var item in e.InitParams)
{
this.Resources.Add(item.Key, item.Value);
}
}
this.RootVisual = new Page();
}
Access and read the values on page.xaml.cs
private void UserControl_Loaded(object sender, RoutedEventArgs e)
{
if (App.Current.Resources.Contains("key1"))
TextBlock1.Text += " key1="+App.Current.Resources["key1"].ToString();
if (App.Current.Resources.Contains("key2"))
TextBlock1.Text += " key2="+App.Current.Resources["key2"].ToString();
}
Things work fine if the initParams
string is restricted to alphanumeric values. But if you want to use any special character then replace the special character with its URL encoded value and then in application use Uri.UnescapeDataString to decode that value as below
Aspx page (%2B is encoding of +)
<asp:Silverlight ID="Xaml1" runat="server" InitParameters="key1=value1,key2=value21 %2Bvalue22" Source="~/ClientBin/InitParamSilverlight.xap" MinimumVersion="2.0.31005.0" Width="100%" Height="100%" />
App.xaml.cs
private void Application_Startup(object sender, StartupEventArgs e)
{
if (e.InitParams != null)
{
foreach (var item in e.InitParams)
{
this.Resources.Add(item.Key, Uri.UnescapeDataString(item.Value));
}
}
this.RootVisual = new Page();
}
The resulting output is
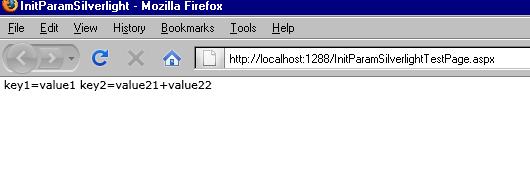