Silverlight is a new cross-browser, cross-platform implementation of the .NET Framework for building and delivering the next generation of media experiences and Rich Interactive Applications(RIA) for the web. It runs in all popular browsers, including Microsoft Internet Explorer, Mozilla Firefox, Apple Safari, Opera. The plugin required to run Silverlight is very small in size hence gets installed very quickly.
It is combination of different technolgoies into a single development platform that allows you to select tools and the programming language you want to use. Silverlight integrates seamlessly with your existing Javascript and ASP.NET AJAX code to complement functionality which you have already created.
Silverlight aims to compete with Adobe Flash and the presentation components of Ajax. It also competes with Sun Microsystems' JavaFX, which was launched a few days after Silverlight.
In this article I m going to discuss how to do database connectivity and show data in DataGrid using Silverlight Enables Web Service.
As you all know just create a new project using Silverlight Application.
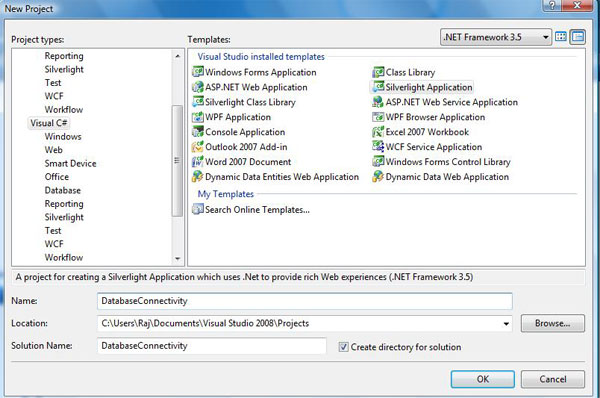
And by default "Add a new Web to the solution for hosting the control" radio button is checked. And click OK.
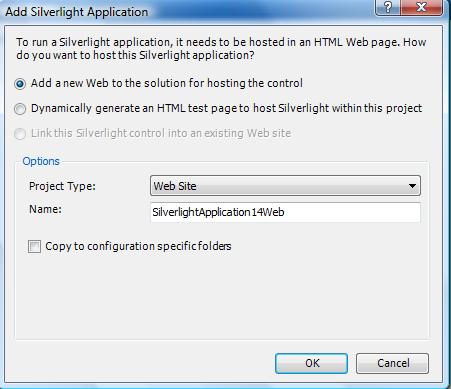
Add a new LINQ to SQL class in App_Code folder.
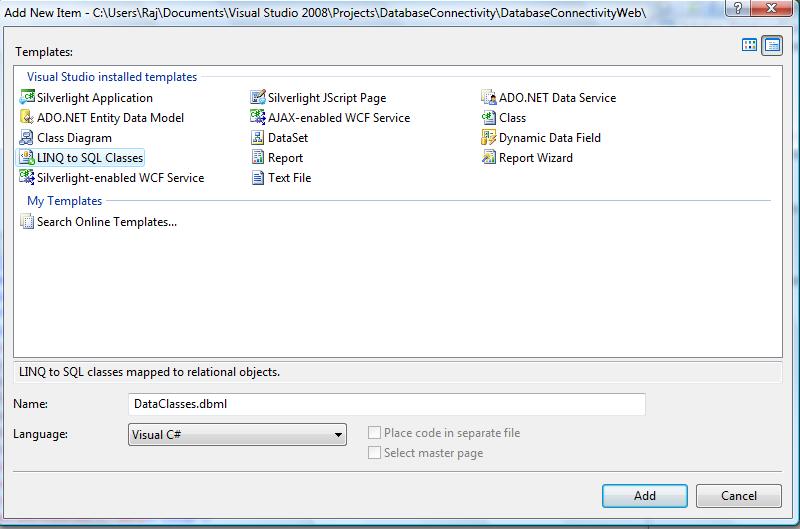
And using server explorer drag and drop your table in DataClass. And you will see like this.
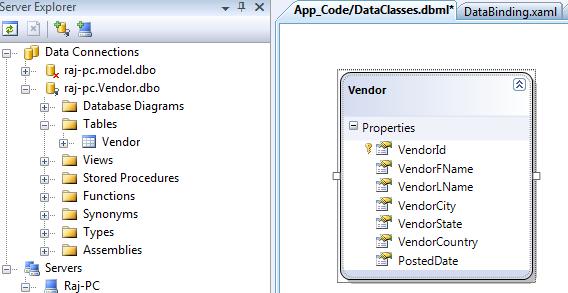
Now add a new Silverlight-enabled WCF Service in Web Project.
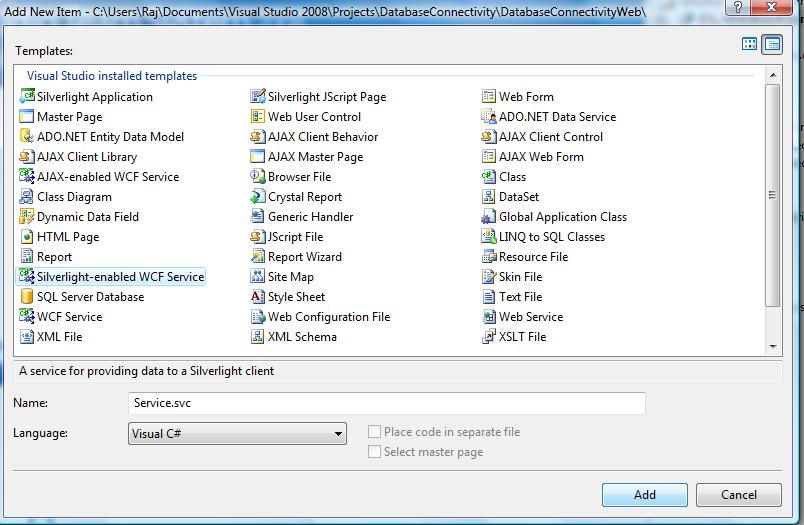
Now let's write some code on Service.cs. I am wriring all code here
using System;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.ServiceModel.Activation;
using System.Collections.Generic;
using System.Text;
[ServiceContract(Namespace = "")]
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]
public class Service
{
[OperationContract]
public List<Vendor> GetVendors()
{
DataClassesDataContext db = new DataClassesDataContext();
var mVendors = from vendor in db.Vendors
select vendor;
return mVendors.ToList();
}
}
I am fetching all data from my Vendor table. You can put your condition according to your requirment.
NOTE - I am putting my database file in APP_Data folder. If you want use my Vendot data.
Now add service reference in References folder.
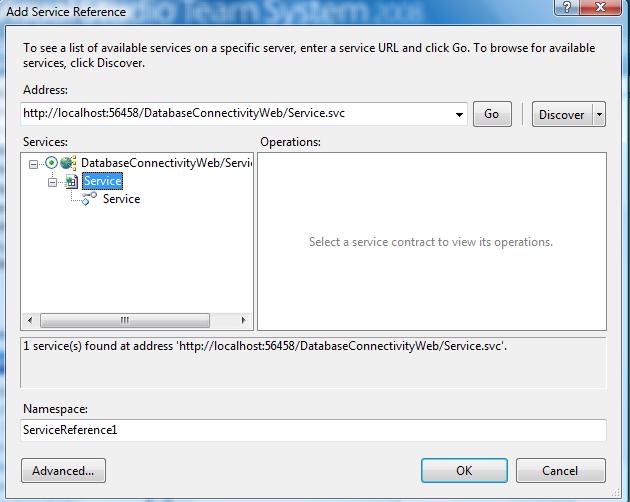
Now let's drag and drop DataGrid on Page.xaml, and bind data using DisplayMemberBinding property.
<UserControl xmlns:my="clr-namespace:System.Windows.Controls;assembly=System.Windows.Controls.Data" x:Class="DatabaseConnectivity.Page"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Width="800" Height="300" Loaded="UserControl_Loaded">
<Grid x:Name="LayoutRoot" Background="White" ShowGridLines="True">
<Grid.RowDefinitions>
<RowDefinition Height="*" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<my:DataGrid x:Name="VendorDataGrid" AlternatingRowBackground="Beige"
AutoGenerateColumns="False"
Width="800" Height="300"
Grid.Row="1" Grid.Column="1"
CanUserResizeColumns="True">
<my:DataGrid.Columns>
<my:DataGridTextColumn Header="VendorId" Width="50" DisplayMemberBinding="{Binding VendorId}" FontSize="11" />
<my:DataGridTextColumn Header="First Name" Width="120" DisplayMemberBinding="{Binding VendorFName}" FontSize="11" />
<my:DataGridTextColumn Header="Last Name" Width="120" DisplayMemberBinding="{Binding VendorLName}" FontSize="11" />
<my:DataGridTextColumn Header="City" Width="120" DisplayMemberBinding="{Binding VendorCity}" FontSize="11" />
<my:DataGridTextColumn Header="State" Width="120" DisplayMemberBinding="{Binding VendorState}" FontSize="11" />
<my:DataGridTextColumn Header="Country" Width="120" DisplayMemberBinding="{Binding VendorCountry}" FontSize="11" />
<my:DataGridTextColumn Header="Join Date" Width="120" DisplayMemberBinding="{Binding PostedDate}" FontSize="11" />
</my:DataGrid.Columns>
</my:DataGrid>
</Grid>
</UserControl>
And this code on Page.xaml.cs
private void UserControl_Loaded(object sender, RoutedEventArgs e)
{
ServiceReference1.ServiceClient webService = new ServiceReference1.ServiceClient();
webService.GetVendorsCompleted += new EventHandler<DatabaseConnectivity.ServiceReference1.GetVendorsCompletedEventArgs>(webService_GetVendorsCompleted);
webService.GetVendorsAsync();
}
void webService_GetVendorsCompleted(Object sender, ServiceReference1.GetVendorsCompletedEventArgs e)
{
VendorDataGrid.ItemsSource = e.Result;
}
Now debug your project and you will get this error message.
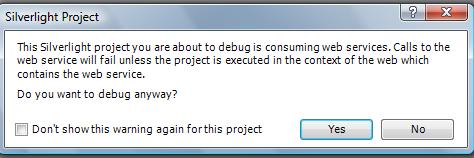
For remove this error message you need to go in propery of Web Project and Do "Use Dyanamic Ports = False".
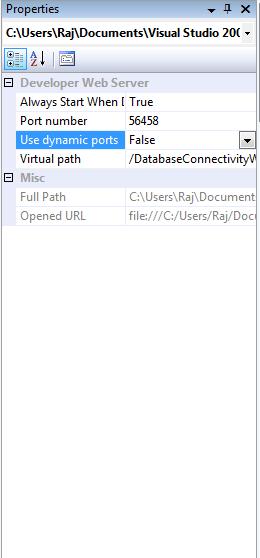
And now set Web Project as startup project and TestPage as set as start page. And debug now. Result will look like this.
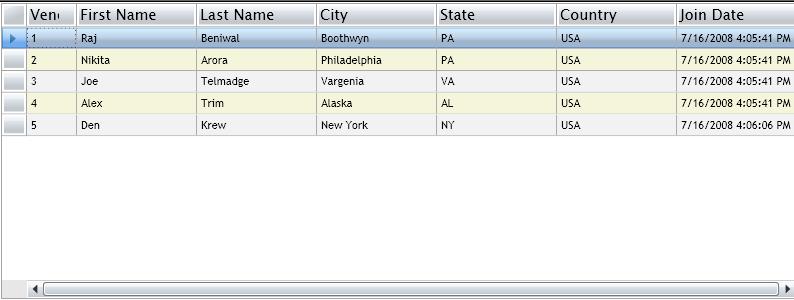
For more information I am attaching my project with database. Database is in App_Data folder. If you have any question or queries then mail me or comment me using c-sharcorner comment section.