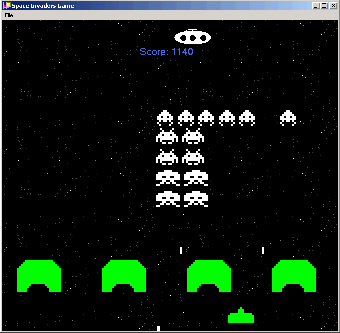
Figure 1 - The Space Invader Game
Yes, the classic arcade game has returned and is appearing in C# Corner complete with sound and authentic aliens and source code. It's not quite as good as the real game, but with a few adjustments it can get there. I actually got many of the sounds and images from a web site that is bent on celebrating this traditional ground breaking game: http://spaceinvaders.retrogames.com. This made writing the game a little bit easier since they supplied many of the files in which you can capture the images and sounds.
Playing the Game
This version is played using the left and right arrow keys to move the man and the space bar to fire. If you hit an alien, you get 10, 20, or 30 points, depending on which one you hit. If a bomb hits you, you die and you only have 3 lives. The shields will protect you from a bomb, so you can hide behind them while you wait for the bombs to pass. Occasionally, a saucer flies across the screen. If you hit it, you get a suprise score (up to 150 points).
Design of the Game
The design for this game is shown below:
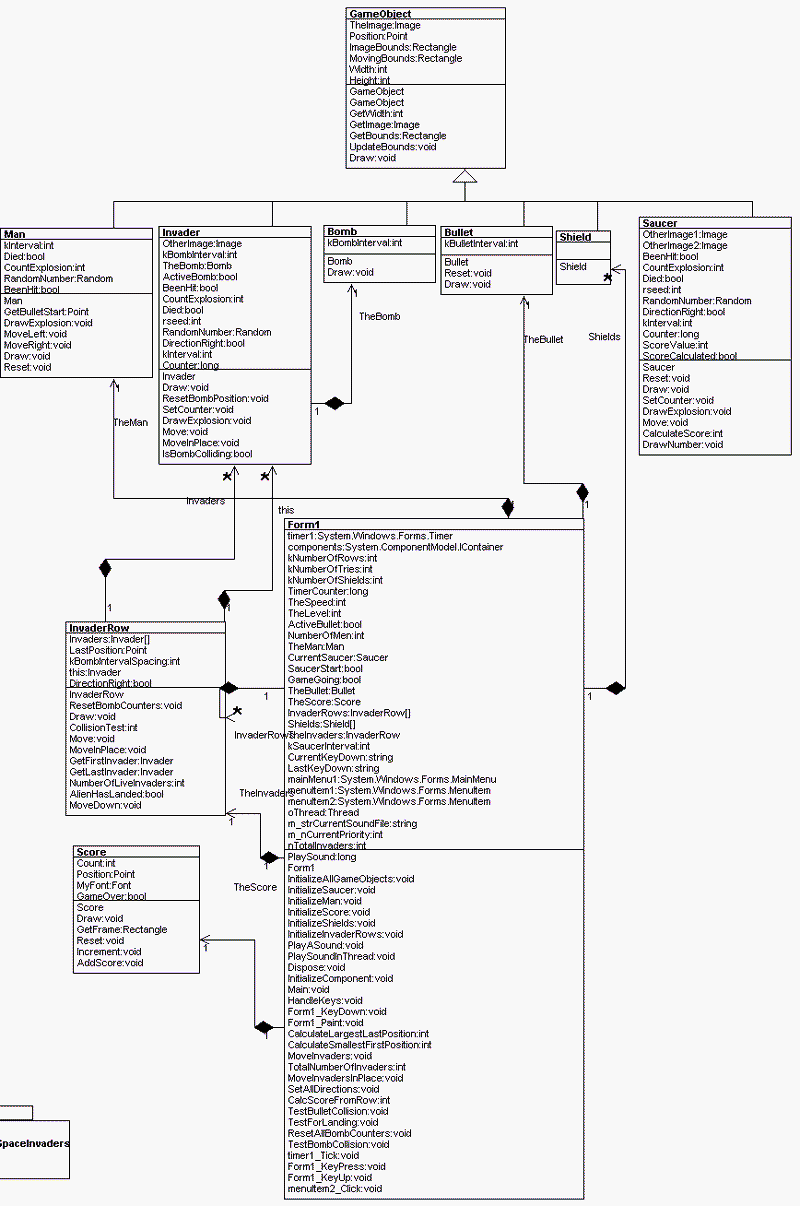
Figure 2 - Space Invader UML Design Reverse engineered using WithClass 2000
The Design basically has a base class GameObject which knows how to draw the game pieces bitmap. Subclassed under this object is all the component classes of the game: Man, Invader, Bullet, Bomb and Shield. A row of Invader classes is controlled through the InvaderRow class. The form contains an array of InvaderRows, an array of Shields, and a Man.
The Timer Event Handler
The entire game is played off of the timer component. After each tick of the timer, the game checks to see if their are bomb collisions, bullet collisions, and whether or not to move the invaders. It also handles the key presses from the user here so as not to change the timing of the game. If a key is pressed, it is remembered by the key press event, but nothing more happens in the key press event handler. Below is the code executed with each entry into the timer event handler:
Listing 1 - The Timer Event Handler in the Form
private void timer1_Tick(object sender, System.EventArgs e)
{
// move the man according to keypresses
HandleKeys();
// Count each timer interval to use it as a reference for timing the game
TimerCounter++;
// if the game is over, just invalidate and return
if (GameGoing == false)
{
// Move the Invaders in place to make them look like they are clapping
if (TimerCounter % 6 == 0)
MoveInvadersInPlace();
Invalidate();
return;
}
// if the bullet shot from the man is off the screen, turn it off
if (TheBullet.Position.Y < 0)
{
ActiveBullet = false;
}
// Fly the saucer every 200 intervals
if (TimerCounter % kSaucerInterval == 0)
{
InitializeSaucer();
PlaySoundInThread("8.wav", 1);
SaucerStart = true;
}
// Move the saucer across the screen
if (SaucerStart == true)
{
CurrentSaucer.Move();
if (CurrentSaucer.GetBounds().Left > ClientRectangle.Right)
{
SaucerStart = false;
}
}
// move invaders every timer interval according to the speed factor
if (TimerCounter % TheSpeed == 0)
{
MoveInvaders();
nTotalInvaders = TotalNumberOfInvaders();
// Change the speed of the Invader according to how many are left
if (nTotalInvaders <= 20)
{
TheSpeed = 5;
}
if (nTotalInvaders <= 10)
{
TheSpeed = 4;
}
if (nTotalInvaders <= 5)
{
TheSpeed = 3;
}
if (nTotalInvaders <= 3)
{
TheSpeed = 2;
}
if (nTotalInvaders <= 1 )
{
TheSpeed = 1;
}
if (nTotalInvaders == 0)
{
// All the invaders were killed
// Start the game again at the next level (move the invader starting position down)
InitializeAllGameObjects(false); // don't initialize score
TheLevel++;
}
}
// Test for bullet and bomb collisions and act appropriately
TestBulletCollision();
TestBombCollision();
// Paint all the game objects
Invalidate();
}
Painting the Game Components
The drawing of all the components is triggered by the Invalidate. Below is the paint event handler that paints all of the game elements:
Listing 2 - The Paint Event Handler in the Form
private void Form1_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
// get the graphics surface
Graphics g = e.Graphics;
// Draw the entire graphics surface black
g.FillRectangle(Brushes.Black, 0, 0, ClientRectangle.Width, ClientRectangle.Height);
// Draw the man
TheMan.Draw(g);
// Draw the score
TheScore.Draw(g);
// Draw the Bullet shot
if (ActiveBullet)
{
TheBullet.Draw(g);
}
// Draw the Saucer
if (SaucerStart)
{
CurrentSaucer.Draw(g);
}
// Draw the Space Invaders
for (int i = 0; i < kNumberOfRows; i++)
{
TheInvaders = InvaderRows[i];
TheInvaders.Draw(g);
}
// Draw the Shields
for (int i = 0; i < kNumberOfShields; i++)
{
Shields[i].Draw(g);
}
}
Improvements:
This game does not erode the shields like the original. This can be done by storing "bomb holes" every time the shield is hit and letting the bombs pass through the holes. Also the bombs coming down could be a bit smarter. For example more bombs could be dropped near the location of the defending ship. Also the design could be improved a bit. A few of the game objects repeat members, so a base class could be used to consolidate these variables (e.g. the BeenHit attribute). Anyway have fun! Don't let those invaders get the best of you!