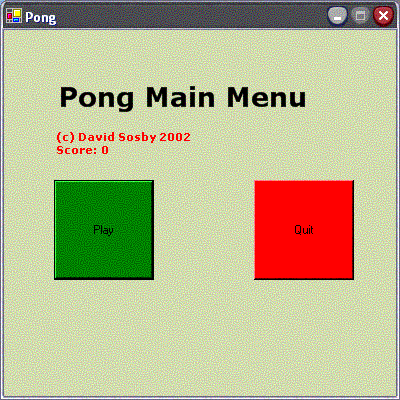
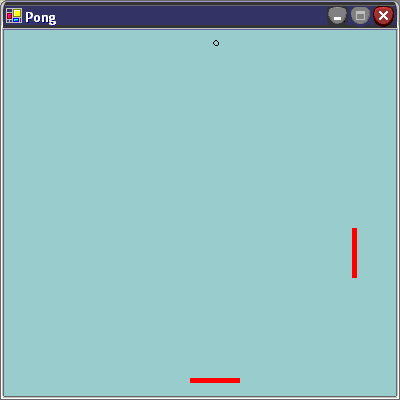
[Purpose]
The purpose of making this classic game was to show simple game structures in C#. Concepts include the "Game Loop", "Input" and "Paint. If you are just beginning in game programming, C# is turning out to be a great place to start.
Also shown are the basics of Forms, along with useful properties. Timers are used to control game speed and morphing color effects. Controls and GDI+ are also demonstrated in this beginner's source code.
Another neat effect that I have demonstrated is a morphing background color. This feature was fairly easy to implement (OK, it was REALLY easy) but adds a degree of design into it and shows another use of the Timer class.
[Game Loop]
In order to control the game speed on all computers, fast or slow, we have to implement a Timer class to run at a specified interval. When the gameTimer ticks, it calls the main game loop (gameLoop()). During this loop, we update all our variables, such as ball_x and ball_y. Once we are done updating, it's time to redraw the game screen. To do this, we call the method Invalidate(). This tells the form that the current window is invalid and in turn sends a Paint event to the form.
[Game ReDraw]
When it is time to redraw the screen, we call the paint1() method with the form's Paint EventHandler. The paint1() method stores the Graphics class that was sent from the EventHandler and calls our game redraw method, gameDraw(). During gameDraw, we test to see if we want to draw the game graphics this frame or not. If we do, we draw out our graphics. If not, we draw nothing.
[Game Input]
All games need input. To implement the input part of our game structure, we put EventHandler's in our Windows form. For mouse input, we use MouseEventHandler. For keyboard input, we use KeyEventHandler.
----Mouse
We call the mouse1() method to handle the mouse input in this program. We receive the current mouse state and store it in e. Now we can access different properties of the current mouse state. In this simple program, we are only using the MouseEventArgs.X and MouseEventArgs.Y properties. These properties hold an integer value of the current X,Y position of the cursor.
//Follow the mouse and move your paddles
private void mouse1(Object sender, MouseEventArgs e)
{
p_x = e.X-25;
p_y = e.Y-25;
}
----Keyboard
The KeyEventArgs class works much like the MouseEventArgs class. In this program, I have decided to use the KeyEventArgs.KeyCode property to figure out which key was hit. Different key values are stored in the Keys class. Here, we compare the KeyEventArgs.KeyCode to the Escape key. If true, we invoke the gameOver() method.
//This tests to see if the Escape key has been hit. If so, gameOver() is called
private void keyHandler(Object sender, KeyEventArgs e)
{
if ( e.KeyCode == Keys.Escape )
gameOver();
}
If you would like to learn all of the available game codes, use your .NET Framework SDK documentation.
[Conclusion]
Now that you know the basic structure of most games, you can set out and make your own games. The key to great game programming and design is to think outside the box. Do something different. Put a great twist on an old game. But most importantly, KEEP IT FUN. Have a nice time!