Introduction
MOSS 2007 or WSS 3.0 based portal has the unique permission levels on any site, list or library.
Permissions on sites, lists, and library are managed nearly 100% by the Business. So many times, when the business needs permissions to a site, list or document, they open a Service Support Request to request permissions. Corporate IT closes this cases by looking-up the permissions on the site, list or document in question, and telling the end user who has permissions on the object in question to grant other permissions. I would like to come up with a way so the end-user can self service, and find out who has permissions to any given site, list or library.
Solution approach
Development of the Site and List Permission Lookup Web part using Microsoft .NET Technologies. And deploy it on WSS3.0/MOSS -2007 Intranet portal.
- Web part will deploy globally and available to all web application within farm
- Web part will be accessible to all authorized Intranet users
- If end-user is having restricted access to any web, list or library. Still able to find out who has the permission
- Separate SharePoint site page will be created for web part
- GUI of Web Part contains Label, Textbox, Search button and Grid View Controls
- Web part will shows list of user having permission level 'Full Control' , 'Manage hierarchy' at site collection, web, list, Document library
- End-user will paste any Intranet site URL inside textbox and click on Go button
- And relevant list of users (Login Name, Username & Email ID) will get display
- And with this end-user can self service and easily find permission to any given site, list, and document library
The Code
MOSS - 2007 URL is normally as below format,
E.g.
Department site: http://intranet.companyname.com/sites/department/department1/pages/default.aspx
Project site: http://intranet.companyname.com/sites/project/P000245/pages/default.aspx
List: http://intranet.companyname.com/sites/project/P000741/list/Calendar/allitem.aspx
Library: http://intranet.companyname.com/sites/project/P000741/library/shareddocument/allitem.aspx
Now based on URL entered in the web part textbox, below is code to identify it's a site, list or library.
if (!(textboxurl.EndsWith(".aspx") || textboxurl.EndsWith("/")))
{
int urllength = textboxurl.Length;
webURL = textboxurl.Insert(urllength, "/");
}
else
{
webURL = textboxurl;
}
using (SPSite site = new SPSite(webURL))
{
using (SPWeb web = site.OpenWeb())
{
if (web.Exists)
{
iLen = web.Url.EndsWith("/") ? web.Url.Length : web.Url.Length + 1;
string sUrlEnd = webURL.Substring(iLen);
string replacespace = sUrlEnd;
replacespace = replacespace.Replace("%20", " ");
After verifying URL three SharePoint Objects created SPWeb, SPList and SPDocumentLibrary and inside each object Role definition sets like "Full Control, Manage hierarchy"
Based on Role Definition users will filtered and the relevant user or SharePoint group users will display in data grid table.
public void gobutton_click(object sender, EventArgs e)
{
try
{
Error.Visible = false;
SPSecurity.RunWithElevatedPrivileges(delegate()
{
string textboxurl = urltextbox.Text;
if (!(textboxurl.EndsWith(".aspx") || textboxurl.EndsWith("/")))
{
int urllength = textboxurl.Length;
webURL = textboxurl.Insert(urllength, "/");
}
else
{
webURL = textboxurl;
}
using (SPSite site = new SPSite(webURL))
{
using (SPWeb web = site.OpenWeb())
{
if (web.Exists)
{
iLen = web.Url.EndsWith("/") ? web.Url.Length : web.Url.Length + 1;
string sUrlEnd = webURL.Substring(iLen);
string replacespace = sUrlEnd;
replacespace = replacespace.Replace("%20", " ");
SPList ActualList = null;
SPList ActualLibrary = null;
foreach (SPList List in web.Lists)
{
if (replacespace.StartsWith(List.RootFolder.Url))
{
if (List is SPDocumentLibrary) // checks if list is document library
{
SPList docLib = (SPDocumentLibrary)List;
{
listtest = true;
ActualLibrary = docLib;
SPRoleDefinitionCollection roleDefinitions = web.RoleDefinitions;
SPRoleDefinition FCROLE = roleDefinitions["Full Control"];
SPRoleDefinition MHROLE = roleDefinitions["Manage Hierarchy"];
SPRoleDefinition ECMROLE = roleDefinitions["Enterprise Content Manager"];
foreach (SPRoleAssignment roleAssigment in ActualLibrary.RoleAssignments)
{
if (roleAssigment.RoleDefinitionBindings.Contains(FCROLE) ||
roleAssigment.RoleDefinitionBindings.Contains(MHROLE) || roleAssigment.RoleDefinitionBindings.Contains(ECMROLE))
{
SPPrincipal oPrincipal = roleAssigment.Member;
try
{
SPUser oRoleUser = (SPUser)oPrincipal;
if (oRoleUser.IsDomainGroup)
{
String ADgrp = oRoleUser.Name.ToString();
if( ADgrp.Contains("\\"))
{
String ADgrp1 = ADgrp.Substring(ADgrp.LastIndexOf('\\') + 1);
GetGroupMember(ADgrp1);
}
else
{
GetGroupMember(ADgrp);
}
}
else
{
if (!( (oRoleUser.IsSiteAdmin)))
{
row = table.NewRow();
row[" User Name "] = oRoleUser.Name.ToString();
row[" Login Name "] = oRoleUser.LoginName.ToString();
row[" Email Address "] = oRoleUser.Email.ToString();
table.Rows.Add(row);
}
}
}
catch (Exception ex)
{
string msg = ex.Message;
}
try
{
SPGroup oRoleGroup = (SPGroup)oPrincipal;
if (oRoleGroup.Users.Count > 0)
{
foreach (SPUser actualUser in oRoleGroup.Users)
{
if (!( (actualUser.IsSiteAdmin) ))
{
row = table.NewRow();
row[" User Name "] = actualUser.Name.ToString();
row[" Login Name "] = actualUser.LoginName.ToString();
row[" Email Address "] = actualUser.Email.ToString();
table.Rows.Add(row);
}
}
}
}
catch (Exception ex)
{
string msg = ex.Message;
}
}
}
}
}
else
{
ActualList = List;
listtest = true;
SPRoleDefinitionCollection roleDefinitions = web.RoleDefinitions;
SPRoleDefinition FCROLE = roleDefinitions["Full Control"];
SPRoleDefinition MHROLE = roleDefinitions["Manage Hierarchy"];
SPRoleDefinition ECMROLE = roleDefinitions["Enterprise Content Manager"];
foreach (SPRoleAssignment roleAssigment in ActualList.RoleAssignments)
{
if (roleAssigment.RoleDefinitionBindings.Contains(FCROLE) ||
roleAssigment.RoleDefinitionBindings.Contains(MHROLE) || roleAssigment.RoleDefinitionBindings.Contains(ECMROLE))
{
SPPrincipal oPrincipal = roleAssigment.Member;
try
{
SPUser oRoleUser = (SPUser)oPrincipal;
if (oRoleUser.IsDomainGroup)
{
String ADgrp = oRoleUser.Name.ToString();
if (ADgrp.Contains("\\"))
{
String ADgrp1 = ADgrp.Substring(ADgrp.LastIndexOf('\\') + 1);
GetGroupMember(ADgrp1);
}
else
{
GetGroupMember(ADgrp);
}
}
else
{
if (!( (oRoleUser.IsSiteAdmin)))
{
row = table.NewRow();
row[" User Name "] = oRoleUser.Name.ToString();
row[" Login Name "] = oRoleUser.LoginName.ToString();
row[" Email Address "] = oRoleUser.Email.ToString();
table.Rows.Add(row);
}
}
}
catch (Exception ex)
{
string msg = ex.Message;
}
try
{
SPGroup oRoleGroup = (SPGroup)oPrincipal;
if (oRoleGroup.Users.Count > 0)
{
foreach (SPUser actualUser in oRoleGroup.Users)
{
if (!( (actualUser.IsSiteAdmin) ))
{
row = table.NewRow();
row[" User Name "] = actualUser.Name.ToString();
row[" Login Name "] = actualUser.LoginName.ToString();
row[" Email Address "] = actualUser.Email.ToString();
table.Rows.Add(row);
}
}
}
}
catch (Exception ex)
{
string msg = ex.Message;
}
}
}
}
if (table.Rows.Count > 0)
{
datagrid1.DataSource = table;
datagrid1.DataBind();
datagrid1.Visible = true;
}
else
{
table.Clear();
datagrid1.Columns.Clear();
datagrid1.DataSource = table;
datagrid1.DataBind();
datagrid1.Visible = false;
Error.Text = "No end users are setup with permissions to this URL";
Error.Visible = true;
}
break;
}
}
if (listtest == false)
{
SPRoleDefinitionCollection roleDefinitions = web.RoleDefinitions;
SPRoleDefinition FCROLE = roleDefinitions["Full Control"];
SPRoleDefinition MHROLE = roleDefinitions["Manage Hierarchy"];
SPRoleDefinition ECMROLE = roleDefinitions["Enterprise Content Manager"];
foreach (SPRoleAssignment roleAssigment in web.RoleAssignments)
{
if (roleAssigment.RoleDefinitionBindings.Contains(FCROLE) || roleAssigment.RoleDefinitionBindings.Contain
(MHROLE) || roleAssigment.RoleDefinitionBindings.Contains(ECMROLE))
{
SPPrincipal oPrincipal = roleAssigment.Member;
try
{
SPUser oRoleUser = (SPUser)oPrincipal;
if (oRoleUser.IsDomainGroup)
{
String ADgrp = oRoleUser.Name.ToString();
if (ADgrp.Contains("\\"))
{
String ADgrp1 = ADgrp.Substring(ADgrp.LastIndexOf('\\') + 1);
GetGroupMember(ADgrp1);
}
else
{
GetGroupMember(ADgrp);
}
}
else
{
if (!( (oRoleUser.IsSiteAdmin)))
{
row = table.NewRow();
row[" User Name "] = oRoleUser.Name.ToString();
row[" Login Name "] = oRoleUser.LoginName.ToString();
row[" Email Address "] = oRoleUser.Email.ToString();
table.Rows.Add(row);
}
}
}
catch (Exception ex)
{
string msg = ex.Message;
}
try
{
SPGroup oRoleGroup = (SPGroup)oPrincipal;
if (oRoleGroup.Users.Count > 0)
{
foreach (SPUser actualUser in oRoleGroup.Users)
{
if (!( (actualUser.IsSiteAdmin) ))
{
row = table.NewRow();
row[" User Name "] = actualUser.Name.ToString();
row[" Login Name "] = actualUser.LoginName.ToString();
row[" Email Address "] = actualUser.Email.ToString();
table.Rows.Add(row);
}
}
}
}
catch (Exception ex)
{
string msg = ex.Message;
}
}
}
if (table.Rows.Count > 0)
{
datagrid1.DataSource = table;
datagrid1.DataBind();
datagrid1.Visible = true;
}
else
{
table.Clear();
datagrid1.Columns.Clear();
datagrid1.DataSource = table;
datagrid1.DataBind();
datagrid1.Visible = false;
Error.Text = "No end users are setup with permissions to this URL.";
Error.Visible = true;
}
}
}
else
{
Error.Text = "Kindly Enter Correct Intranet URL";
}
}
}
}
);
}
catch (System.UriFormatException)
{
table.Clear();
datagrid1.Columns.Clear();
datagrid1.DataSource = table;
datagrid1.DataBind();
datagrid1.Visible = false;
Error.Text = "Invalid URL - Ensure the format is "Your Intranet site URL";
Error.Visible = true;
}
catch (Exception ex)
{
table.Clear();
datagrid1.Columns.Clear();
datagrid1.DataSource = table;
datagrid1.DataBind();
datagrid1.Visible = false;
Error.Visible = true;
string msg = ex.Message;
Error.Text = "The URL "+ urltextbox.Text + " is not a part of "Your Intranet site URL" Only content on "Your Intranet site URL" can be queried for permissions.";
}
}
If suppose Active directory group is being directly added to the list or library then kindly use below code to find relevant users of that AD group.
public void GetGroupMember(string strGroup)
{
try
{
DirectorySearcher srch = new DirectorySearcher("(CN=" + strGroup + ")");
SearchResultCollection coll = srch.FindAll();
foreach (SearchResult rs in coll)
{
ResultPropertyCollection resultPropColl = rs.Properties;
foreach (Object memberColl in resultPropColl["member"])
{
DirectoryEntry gpMemberEntry = new DirectoryEntry("LDAP://" + memberColl.ToString().Replace("/", "\\/"));
System.DirectoryServices.PropertyCollection userProps = gpMemberEntry.Properties;
object obVal = userProps.Contains("displayName") ? userProps["displayName"].Value : "Unknown"; //Avoids an
exception if property is null.
object obAcc = userProps.Contains("sAMAccountName") ? userProps["sAMAccountName"].Value : "Unknown"; //Avoids an exception
if property is null.
Object obemail = userProps.Contains("mail") ? userProps["mail"].Value : "Unknown"; //Avoids an exception if
property is null.
row = table.NewRow();
row[" User Name "] = obVal.ToString();
row[" Login Name "] = obAcc.ToString();
row[" Email Address "] = obemail.ToString();
table.Rows.Add(row);
}
}
coll.Dispose(); // It is important to dispose of SearchResultCollection objects, as garbage collection won't release them.
}
catch (Exception ex)
{
string msg = ex.ToString();
Error.Text = ex.Message + " Detail: " + msg;
Error.Visible = true;
}
}
Technical design & solution
Microsoft Visual Studio 2005 is used for development of web part. Important detail technical design is as below.
- Create Class library project from visual studio 2005
- As the web part is developed using SharePoint object model, first we need to add reference of below listed assemblies Microsoft.SharepointMicrosoft.office.Sharepoint
a. Microsoft.Sharepoint
b. Microsoft.office.Sharepoint
c. System. Data
d. System. Drawing
- Under "CreateChildControl()"method add below listed controls
a. Label for title ("Enter Site or List URL :")
b. Textbox for entering URL link
c. Button with On click event handler
d. Grid view control for displaying return results
e. Add columns in grid view and set CSS properties
f. Label for error message
- on click method of "GO button"
a. Web part application is going to fetch permission information of any given site, List and library, which may be restricted to some group of users. So we need to write our functionality inside "RunWithElevatedPrivileges" method
b. Now based on URL enter inside textbox, we need to identify its a web, list or library
c. Also need to replace "%20" character with " " (space)
d. Once the URL corrected then using SharePoint object model create object of site, web, list or library
e. Using SPListDefinitionCollection set all required permission level
In our case we are using below permission level
i. Full Control
ii. Manage Hierarchy
Note: In future if new permission level needs to add then write inside SPlistDefinitionCollection
f. Now based on above permission filter users/groups associated to given web, list or library using roleAssigment.RoleDefinitionBindings
g. If results contains SharePoint group then find all users inside that group
h. Also filter Farm administration account from return result by using "IsSiteAdmin" condition.
User accounts excluded from return results having below condition,
I. System admin Users will get filtered
ii. If site, list or library contains domain group then it will be treat under SPUser object and for fetching members of that group we need to setup a new connection with LDAP server and supplies the group name to it
j. Now adding all return result users information row inside table object, and at the end bind the table to data grid
k. Exception handling done using "Try catch and finally ", all exception is display in a label (error)
Application Design Diagram
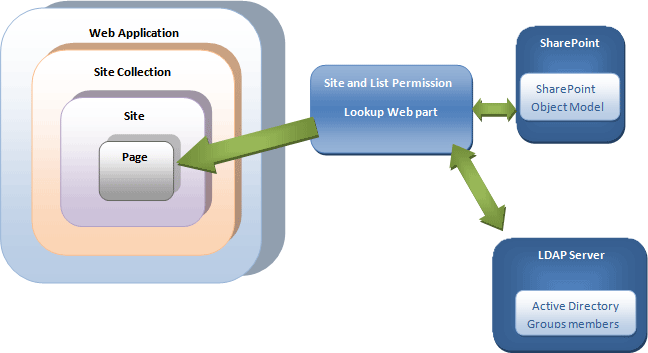
Screenshots of the web part
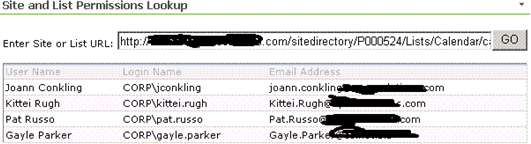
Deployment Details
Installation steps for SharePoint Environment
- From web part project file, open folders 'Package >> bin '
- Copy QueryPermission.wsp file and save it on MOSS CA application server
- Open command prompt on application server go to below directory

- Run the command : stsadm –o addsolution –filename "wsp file path"
e.g if Querypermission.wsp saved at D:\QPwebpart\Querypermission.wsp then command will be,
stsadm -o addsolution -filename "D:\QPwebpart\QueryPermission.wsp"

- Now open Central Administration and go to ' operations >> solution management '
- QueryPermission.wsp will be visible , double click on it and deploy the solution globally
- Safe Control Entry
<SafeControl Assembly="QueryPermission, Version=1.0.3800.24661, Culture=neutral, PublicKeyToken=359764589431b540" Namespace="QueryPermissionWebpart" TypeName="*" Safe="True" />
- Populate intranet webpart gallery
a. Now open web application
b. Go to ' site actions >> site settings >> modify all site settings '
c. Click on ' web parts' under galleries group
d. Now web part gallery list will open , click on 'New' link from list header
e. Now from available list of new webpart gallery search for 'Querypermissionwebpart.querypermission'
f. Click the checkbox and from top of the page click populate gallery button
g. Now the web part will be available to use on Intranet Portal
- Add web part to page
a. Create new blank web part page on intranet portal
b. Open the newly created page and from site action click 'Edit page' option
c. From left webpart zone click ' add web part ' link
d. Now "Add web part - Web part Dialog " window opens and go to Miscellaneous category
e. Find "querypermission", tick mark the checkbox and click OK
f. Now Query permission webpart available on that page
Thanks
I faced a problem in fetching users from active directory group. My team leader Scott Dirlam and Jayson Tautic helped me to figure out the solution. So I personally thank him. I have taken for granted that someone will face the same problem and they may get help from this article.