Session State Management: a storage mechanism that is accessible from all pages requested by a single Web browser session. Therefore, you can use session state to store user-specific information. A session is defined as the period of time that a unique user interacts with a Web application. Active Server Pages (ASP) developers who wish to retain data for unique user sessions can use an intrinsic feature known as session state.
Advantages:
- Uniquely identify browser or client-device requests and map them to individual session instances on the server. This allows you to track which pages a user saw on your site during a specific visit.
- Store session-specific data on the server for use across multiple browsers or client-device requests during the same session. This is perfect for storing shopping cart information.
- Raise appropriate session management events. In addition, you can write application code leveraging these events.
How and where ASP.NET session state stores data: We know how ASP.NET handles an HTTP request; actually it flows through a HTTP pipeline of different modules that can react to application events. One of the modules in this chain is the SessionStateModule (in the System.Web.SessionState namespace). The job of this module SessionStateModule is to generate the session ID, retrieves the session data from external state providers, and binds the data to the call context of the request. But actually SessionStateModule doesn't actually store the session data. Instead, the session state is persisted in external components, which are named state providers.
These state providers are listed below:
- In-Memory objects.
- Windows Service (aspnet_state.exe)
- Tables in ASPState database.
What is the role of session id: Session state is maintained by Session ID which is the only piece of information that is transmitted between the web server and the client. When the client presents the session ID, ASP.NET looks up the corresponding session, retrieves the serialized data from the state server, converts it to live objects, and places these objects into a special collection so they can be accessed in code. This process takes place automatically.
How session state works: For session state to work, the client needs to present the appropriate session ID with each
request. The final ingredient in the puzzle is how the session ID is tracked from one request to the next. You can accomplish this in two ways:
- Using cookies: In this case, the session ID is transmitted in a special cookie (named ASP.NET_SessionId), which ASP.NET creates automatically when the session collection is used. This is the default.
- Using modified URLs: In this case, the session ID is transmitted in a specially modified (or "munged") URL.
How to use Session State:
Class Name: System.Web.SessionState.HttpSessionState
Storing dataset in session variable,
Session["ProductsDataSet"] = dsProducts;
Retrieving value from session variable;
dsProducts = (DataSet)Session["ProductsDataSet"];
When the session variable value will be lost:
- If browser is closed and restarted by user.
- If same page is accessed or browsed via a different browser window
- If the user accesses the same page through a different browser window, although the session
will still exist if a web page is accessed through the original browser window. Browsers differ on how they handle this situation.
- If the session times out because of inactivity. By default, a session times out after 20 idle minutes.
- If the programmer ends the session by calling Session.Abandon().
- When application domain will be recreated.
How to configure Session State in web.config
Below is a sample config.web file used to configure the session state settings for an ASP.NET application:
<sessionState
mode="InProc"
stateConnectionString="tcpip=127.0.0.1:42424"
stateNetworkTimeout="10" sqlConnectionString="data source=127.0.0.1;Integrated Security=SSPI"
sqlCommandTimeout="30"
allowCustomSqlDatabase="false"
useHostingIdentity="true"
cookieless="UseCookies"
cookieName="ASP.NET_SessionId"
regenerateExpiredSessionId="false"
timeout="20"
customProvider="">
</sessionState>
What are Session Modes:
1. InProc:
- ASP.NET to store information in the current application domain.
- Once the server is restarted, the state information will be lost. i.e. session state is managed in process and if the process is re-cycled, state is lost.
- It's default option
- High performance because the time it takes to read from and write to the session state dictionary, will be much faster when the memory read to and from is in process
2. Off:
- This setting disables session state management for every page in the application.
- it can provide a slight performance improvement for websites that are not using session state.
3. StateSever:
- the objects you store in a session state must be serializable.
- ASP.NET will use a separate Windows service for state management
- the problem is that increased time is delay imposed when state information is transferred between two processes. If you frequently access and change state information, this can make for a fairly unwelcome slowdown.
To use this in your application, you need to start it.
Browse this path:
Select Start -> Programs -> Administrative Tools-> Computer Management-> Services and Applications->service node; find the service called ASP.NET State Service in the list.
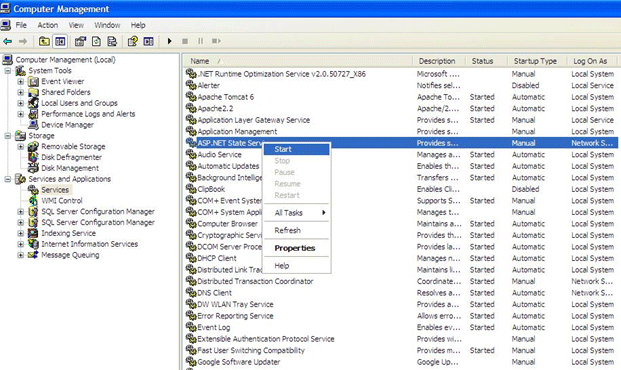
From here you can manually start and stop this service.
4. Custom:
- while using custom mode, we need to indicate what session state store provider to use by supplying the customProvider attribute.
- we use custom mode when we need to store session information in a database other than SQL Server or to use an existing table in a database that has a specific schema.
- the customProvider attribute directs to the name of a class that's part of web application in the App_Code directory, or in a compiled assembly in the Bin directory or the GAC.
What is CookieLess attribute in session state configuration setting.
cookieless="UseCookies"
This feature with which we can configure for ASP.NET session state is cookieless session state. Essentially this feature allows sites whose clients choose not to use cookies to take advantage of ASP.NET session state.
How it works: ASP.NET will modify relative links found within the page and embed this ID. Thus, as long as the user follows the path of links the site provides, session state can be maintained. This is done by modifying the URL with an ID that uniquely identifies the session:
http://localhost/(lit3py55t21z5v55vlm25s55)/Application/SessionState.aspx
Let us understand these attributes in detail
- useCookie: Cookies are always used, even if the browser or device doesn't support cookies or they are disabled. This is the default
- useURI: Cookies are never used, regardless of the capabilities of the browser or device. Instead, the session ID is stored in the URL.
- UseDeviceProfile: ASP.NET chooses whether to use cookieless sessions by examining the BrowserCapabilities object. The drawback is that this object indicates what the device should support
- AutoDetect: ASP.NET attempts to determine whether the browser supports cookies by attempting to set and retrieve a cookie
How to control timeout for session state: Timeout specifies the number of minutes that ASP.NET will wait, without receiving a request, before it abandons the session,
timeout="20"
You can also programmatically change the session time-out in code.
Session.Timeout = 10;
Reference read from msdn here:
http://msdn.microsoft.com/en-us/library/ms972429.aspx
Session Example: The idea here is to create product detail class and save the items in session and bind it to listbox. On selecting a product from the listbox we provide full detail for that product. Here we also show and use session attribute to show session id , mode, cookieless etc.
public class Product
{
public string name;
public string description;
public double cost;
public Product(string sname, string sdescription, double scost)
{
name = sname;
description = sdescription;
cost = scost;
}
}
Creating a product object, storing them in session variable and adding it in listbox
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
Product product1 = new Product("laptop", "Apple", 1234);
Product product2 = new Product("camera", "Sony", 5433);
Product product3 = new Product("watch", "Omega", 6789);
Session["product1"] = product1;
Session["product2"] = product2;
Session["product3"] = product3;
lstItems.Items.Clear();
lstItems.Items.Add(product1.name);
lstItems.Items.Add(product2.name);
lstItems.Items.Add(product3.name);
}
// Display some basic information about the session.
// This is useful for testing configuration settings.
lblSession.Text = "Session ID: " + Session.SessionID;
lblSession.Text += "<br>Number of Objects: ";
lblSession.Text += Session.Count.ToString();
lblSession.Text += "<br>Mode: " + Session.Mode.ToString();
lblSession.Text += "<br>Is Cookieless: ";
lblSession.Text += Session.IsCookieless.ToString();
lblSession.Text += "<br>Is New: ";
lblSession.Text += Session.IsNewSession.ToString();
lblSession.Text += "<br>Timeout (minutes): ";
lblSession.Text += Session.Timeout.ToString();
}
On More information click, we are retrieving more details from the session variable and displaying them.
protected void cmdMoreInfo_Click(object sender, EventArgs e)
{
if (lstItems.SelectedIndex == -1)
{
lblRecord.Text = "You have not seleected any product";
}
else
{
string key = "product" + (lstItems.SelectedIndex + 1).ToString();
Product p = (Product)Session[key];
if (p != null)
{
lblRecord.Text = "Name: " + p.name;
lblRecord.Text += "<br>Description: ";
lblRecord.Text += p.description;
lblRecord.Text += "<br>Cost: $" + p.cost.ToString();
}
}
}
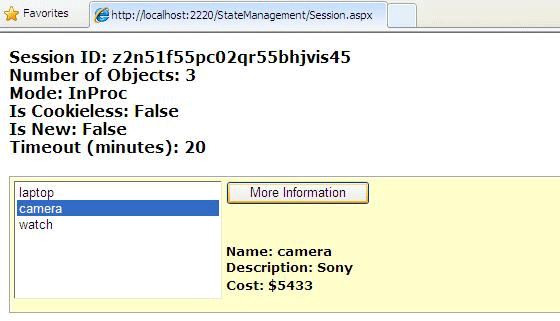
This is the end of your fourth hour of reading. Hope you enjoyed it.
Click below to continue to the fifth hour of reading.
Cheers.........