Files:
-
Form File(Form1)
-
Class File (DataSourceDatagrid.cs)
File: Form1.cs[Design]
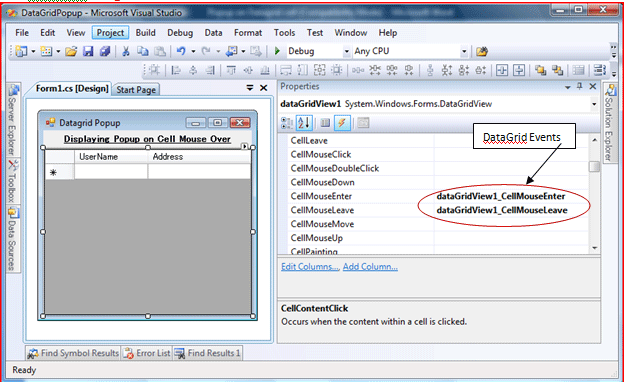
File: Form1.cs ->
using System;
using System.Collections;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Windows.Controls.Primitives;
using System.Windows.Controls;
namespace DataGridPopup
{
public partial class Form1 : Form
{
//Members for Popup declared here so that only one instance is managed for the popup //at a time.
Popup codePopup = new Popup();
TextBlock popupText = new TextBlock();
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
ArrayList arrSrc = new ArrayList();
try
{
//Binding Datagrid with data:
arrSrc.Add(new DataSourceDatagrid("SAN", "Pune"));
arrSrc.Add(new DataSourceDatagrid("Dipak", "Mumbai"));
arrSrc.Add(new DataSourceDatagrid("Kiran", "Delhi"));
arrSrc.Add(new DataSourceDatagrid("SAN1", "Pune1"));
arrSrc.Add(new DataSourceDatagrid("Dipak1", "Mumbai1"));
arrSrc.Add(new DataSourceDatagrid("Kiran1", "Delhi1"));
arrSrc.Add(new DataSourceDatagrid("SAN2", "Pune2"));
arrSrc.Add(new DataSourceDatagrid("Dipak2", "Mumbai2"));
arrSrc.Add(new DataSourceDatagrid("Kiran2", "Delhi2"));
dataGridView1.DataSource = arrSrc;
}
catch (Exception ex)
{
ex = new Exception("Error occurred while binding the datasource to datagrid.
" + ex.Message);
throw ex;
}
}
private void dataGridView1_CellMouseEnter(object sender, DataGridViewCellEventArgs e)
{
try
{
//Additional Check: to see if there is a valid row-column cell for the datagrid on each mouse over event:
if (e.RowIndex >= 0 && e.RowIndex < dataGridView1.RowCount
&& e.ColumnIndex >= 0 && e.ColumnIndex < dataGridView1.ColumnCount)
{
codePopup.Visibility = System.Windows.Visibility.Hidden;
codePopup.IsOpen = false;
popupText.Text = "Information: "
+ System.Environment.NewLine
+ "Name: " + dataGridView1[0, e.RowIndex].Value.ToString()
+ System.Environment.NewLine
+ "Address: " + dataGridView1[1, e.RowIndex].Value.ToString();
popupText.Background = System.Windows.Media.Brushes.LightBlue;
popupText.Foreground = System.Windows.Media.Brushes.Blue;
codePopup.Child = popupText;
codePopup.Placement = System.Windows.Controls.Primitives.PlacementMode.MousePoint;
codePopup.Height = 50;
codePopup.Width = 100;
codePopup.IsOpen = true;
}
}
catch (Exception ex)
{
ex = new Exception("Error occurred while displaying the popup for datagrid cell. " +
ex.Message);
throw ex;
}
}
private void dataGridView1_CellMouseLeave(object sender, DataGridViewCellEventArgs e)
{
try
{
codePopup.IsOpen = false;
}
catch (Exception ex)
{
ex = new Exception("Error occurred while hiding the popup for datagrid cell. " +
ex.Message);
throw ex;
}
}
}
}
File: DataSourceDatagrid.cs ->
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace DataGridPopup
{
public class DataSourceDatagrid
{
private string userName = string.Empty;
private string address = string.Empty;
public string UserName
{
get
{
return this.userName;
}
set
{
this.userName = value;
}
}
public string Address
{
get
{
return this.address;
}
set
{
this.address = value;
}
}
public DataSourceDatagrid(string usrName, string addr)
{
userName = usrName;
address = addr;
}
}
}
>> And, there are so many possiblities with the Popup Class that can be played with for better and attractive GUI etc.