Description: In this article I will describe how to do a filter search by comparing two given attributes in another window.
Content:
Now first we have to grid the JQ grid and bind the data from an array source.
After that we have to write the search Jquery for the JQ grid.
For downloading the JQ Grid js file go to the following site:
http://www.trirand.com/blog/?page_id=6
Now I will create an ASP.Net application where I will display the record from an array through the JQ Grid.
Step 1:
In VS 2010 first create a simple ASP.Net web project named "JQgridapplication".
Step 2:
After that add the required JS file and folder for the JQ grid. The folder and file structure will look like the following figure1 marked with red.
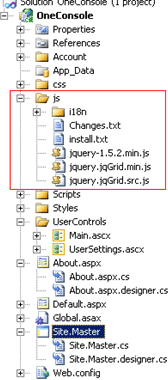
Figure 1
Step 3:
Now create a folder named "UserControls" under the main project. After that create a user control named "Main.ascx" under the "UserControls" folder.
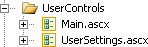
Figure 2
Step 4:
Now in the Main.ascx file first call all the related JQ Grid related Js files:
<script src="../js/jquery-1.5.2.min.js" type="text/javascript"></script>
<script src="../js/i18n/grid.locale-en.js" type="text/javascript"></script>
<script src="../js/jquery.jqGrid.min.js" type="text/javascript"></script>
<script src="../Scripts/json2-min.js" type="text/javascript"></script>
After that for JQ Grid paste the following code:
<script type="text/javascript">
$(document).ready(function () {
jQuery("#list1").jqGrid({
datatype: "local",
colNames: ['Inv No', 'Date', 'Client', 'Amount', 'Tax', 'Total', 'Notes'],
colModel: [
{ name: 'id', index: 'id', width: 60, sorttype: "int" },
{ name: 'invdate', index: 'invdate', width: 90, sorttype: "date" },
{ name: 'name', index: 'name', width: 100 },
{ name: 'amount', index: 'amount', width: 80, align: "right", sorttype: "float" },
{ name: 'tax', index: 'tax', width: 80, align: "right", sorttype: "float" },
{ name: 'total', index: 'total', width: 80, align: "right", sorttype: "float" },
{ name: 'note', index: 'note', width: 150, sortable: false }
],
multiselect: true,
rowNum: 10,
rowList: [5, 10, 20, 50, 100],
pager: jQuery('#pager1'),
sortorder: "desc",
viewrecords: true,
caption: "Manipulating Array Data"
});
var mydata = [
{ id: "1", invdate: "2007-10-01", name: "test", note: "note", amount: "200.00", tax: "10.00", total: "210.00" },
{ id: "2", invdate: "2007-10-02", name: "test2", note: "note2", amount: "300.00", tax: "20.00", total: "320.00" },
{ id: "3", invdate: "2007-09-01", name: "test3", note: "note3", amount: "400.00", tax: "30.00", total: "430.00" },
{ id: "4", invdate: "2007-10-04", name: "test", note: "note", amount: "200.00", tax: "10.00", total: "210.00" },
{ id: "5", invdate: "2007-10-05", name: "test2", note: "note2", amount: "300.00", tax: "20.00", total: "320.00" },
{ id: "6", invdate: "2007-09-06", name: "test3", note: "note3", amount: "400.00", tax: "30.00", total: "430.00" },
{ id: "7", invdate: "2007-10-04", name: "test", note: "note", amount: "200.00", tax: "10.00", total: "210.00" },
{ id: "8", invdate: "2007-10-03", name: "test2", note: "note2", amount: "300.00", tax: "20.00", total: "320.00" },
{ id: "9", invdate: "2007-09-01", name: "test3", note: "note3", amount: "400.00", tax: "30.00", total: "430.00" }
];
for (var i = 0; i <= mydata.length; i++)
jQuery("#list1").jqGrid('addRowData', i + 1, mydata[i]);
});
// });
</script>
Here I am defining the JQ Grid and binding it through an array.
Now I have to write the Jquery for the Jq Grid for searching.
The code we have to write just after the JQ grid definition:
jQuery("#list1").jqGrid('navGrid', '#pager1', { del: false, add: false, edit: false }, {}, {}, {}, { multipleSearch: true });
Like figure 3:
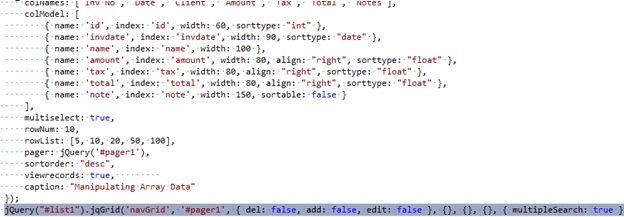
Figure 3:
Step 5:
Now in the "Default.aspx" we have to call the "Main.ascx" UserControl.
So the code is:
<%@ Page Title="Home Page" Language="C#" MasterPageFile="~/Site.master" AutoEventWireup="true"
CodeBehind="Default.aspx.cs" Inherits="OneConsole._Default" %>
<%@ Register TagPrefix="OneConsole" TagName="MainControl" Src="~/UserControls/Main.ascx" %>
<asp:Content ID="HeaderContent" runat="server" ContentPlaceHolderID="HeadContent">
</asp:Content>
<asp:Content ID="BodyContent" runat="server" ContentPlaceHolderID="MainContent">
<h2>
OneConsole
</h2>
<OneConsole:MainControl ID="mainControl" runat="server" />
</asp:Content>
Step 6:
Now run the Application. It will look like the following Figure 4.
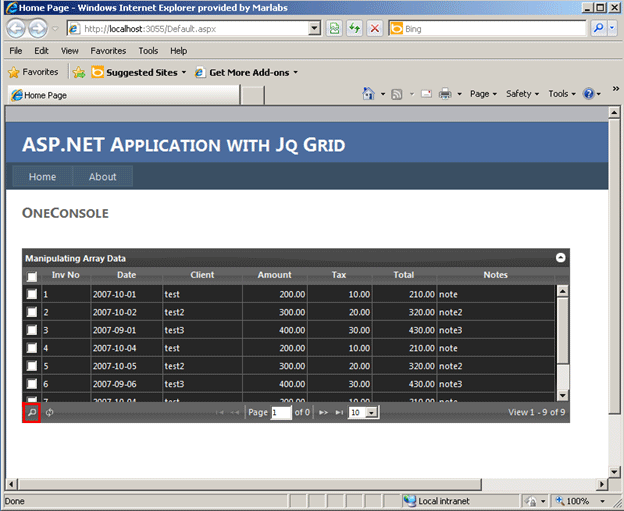
Figure 4:
See in the above picture you have seen a marked red version. This is actually the filter button. When you click this it will look like the following Figure 5.
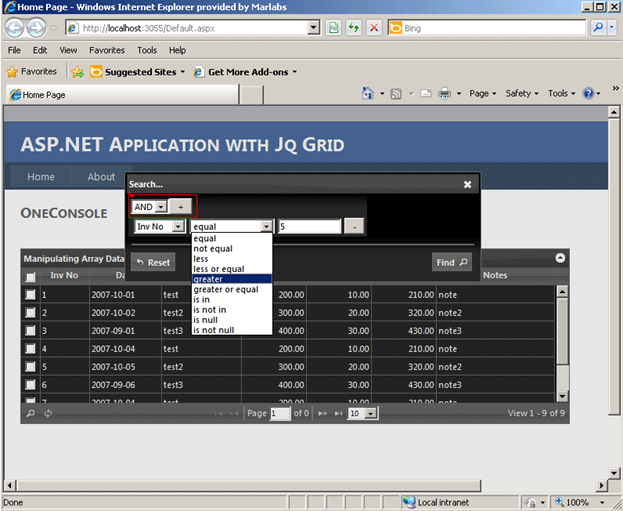
Figure 5:
In the above picture you have seen that another window opens. Where you can filter your required query through AND or OR condition which I marked with red.
Here in the above picture I am filtering all my Inv No which are greater than 5.
After giving the value when I press the Find button it will display the result in the grid like in Figure 6.
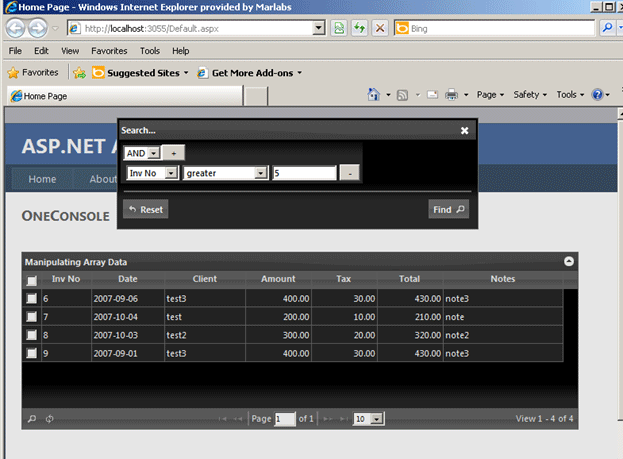
Figure 6:
See the Inv No which is greater than 5 is showing here.
Now when I press the reset button the grid will load the original data.
Conclusion: So in this article I have described how to achieve the filter search in JQ grid.