Introduction
An Object Expression is an F# expression that creates a new type "on the fly". The new type created is Anonymous, meaning it has no accessibility and must be based on an existing base type interface or set of interfaces. Object Expressions are at the heart of object oriented programming in F#. They provide a concise syntax to create an object that inherits from an existing type.
The Object Expression feature provdes us the ability to create anonymous inner classes that implement a certain interface. This is useful when you are only making use of an implementation of an interface in one place in the code and do not want to expose this type in other code. This is a cool feature of F#. This allows us to easily instantiate classes.
Object Expressions implement abstract types. This allows us to implement an abstract type without creating a concrete type and it is particularly useful when you need to build an implementation on the fly using functions already defined somewhere else in the program.
Object Expressions are not limited to interfaces; you can use them whenever you want to inject a bit of implementation without creating a whole new type. An Object Expression allows you to provide an implementation of the class while at the same time creating a new instance of it.
Each object expression defines a different way to sort a list of names. Without the use of an Object Expression, this would take two separate type definitions, each implementing IComparer<'a>. But, from the use of object expressions, no new explicit types were required.
We generally use an object expression to create types that are used locally and that participate in a different situation. The canonical example used for Object Expression is that of object comparison with an eye towards sorting; I think it's a useful example as it captures the essence of Object Expression and demonstrates their uses in a few lines of code.
Here I am discussing an example in which I will create a record Empl using Object Expression to generate classes that can sort employees based on first name and salary.
Example
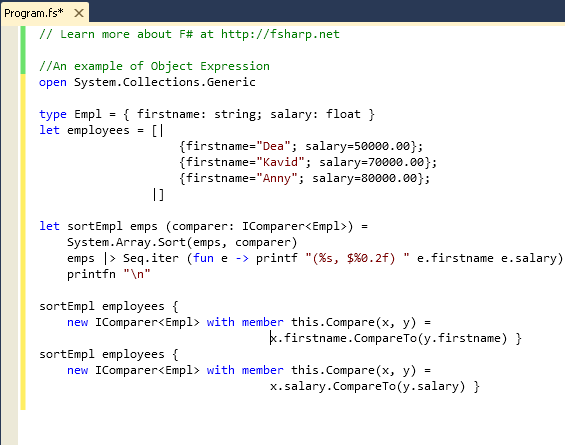
//An example of Object Expression
open System.Collections.Generic
type Empl = { firstname: string; salary: float }
let employees = [|
{firstname="Dea"; salary=50000.00};
{firstname="Kavid"; salary=70000.00};
{firstname="Anny"; salary=80000.00};
|]
let sortEmpl emps (comparer: IComparer<Empl>) =
System.Array.Sort(emps, comparer)
emps |> Seq.iter (fun e -> printf "(%s, $%0.2f) " e.firstname e.salary)
printfn "\n"
sortEmpl employees {
new IComparer<Empl> with member this.Compare(x, y) =
x.firstname.CompareTo(y.firstname) }
sortEmpl employees {
new IComparer<Empl> with member this.Compare(x, y) =
x.salary.CompareTo(y.salary) }
Output
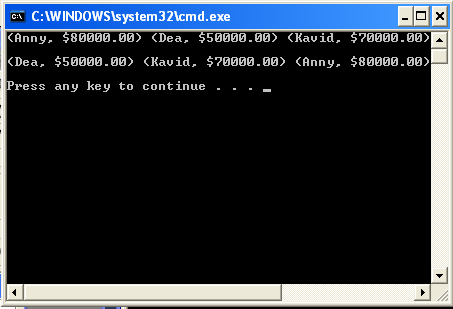
Example
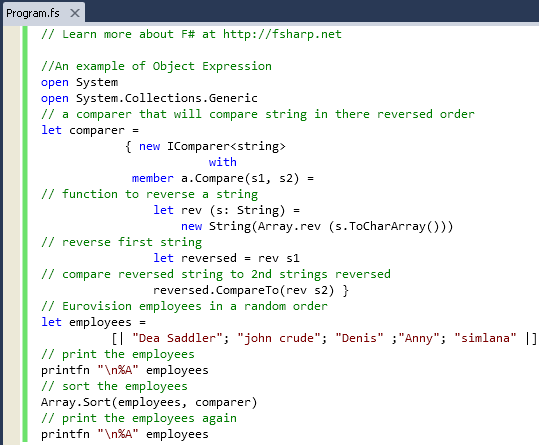
//An example of Object Expression
open System
open System.Collections.Generic
// a comparer that will compare string in there reversed order
let comparer =
{ new IComparer<string>
with
member a.Compare(s1, s2) =
// function to reverse a string
let rev (s: String) =
new String(Array.rev (s.ToCharArray()))
// reverse first string
let reversed = rev s1
// compare reversed string to 2nd strings reversed
reversed.CompareTo(rev s2) }
// Eurovision employees in a random order
let employees =
[| "Dea Saddler"; "john crude"; "Denis" ;"Anny"; "simlana" |]
// print the employees
printfn "\n%A" employees
// sort the employees
Array.Sort(employees, comparer)
// print the employees again
printfn "\n%A" employees
Output
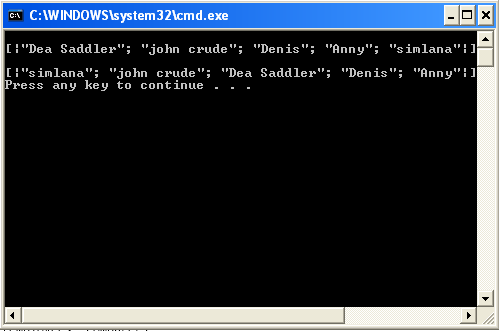
Object Expressions are a powerful mechanism to quickly and concisely introduce object oriented functionality from objects, non F# libraries into your F# code. The main disadvantage of the Object Expression is that they do not allow you to add extra methods or properties to these Objects.
Summary
In this article I have discussed about Object Expressions in F#.