Introduction
LINQ provides many operators that support making various types of queries with any type of object.
An aggregate operator's main purpose is to return a single value; aggregate operators include Aggregate, Average, Count, LongCount, Max, Min and Sum. In this article we are going to see how to implement the three aggregate operators Max, Min and Sum in order to analyze ProductInventoryAmount class to extract some values as a notification about the Inventory level to notify people to take action when needed.
First, we made ProductInventoryAmount class to host basic properties related to the class activity, then we created an object of type List<> to host ProductInventoryAmount class. After that we created a variety of objects that represent different types of products with various states. Up to the point, using LINQ aggregate functions to analyse inventory level and show the results in dataGridView1.
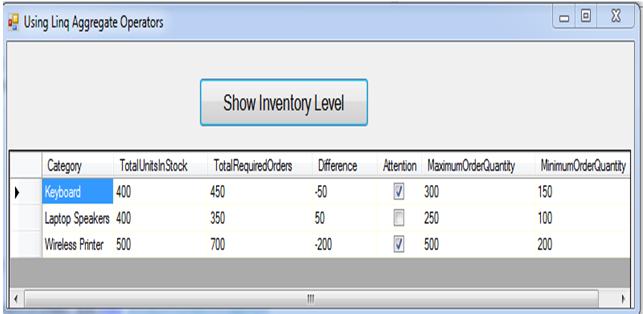
using System;
using System.Collections.Generic;
using System.Linq;
using System.Windows.Forms;
namespace UsingLinqAggregateOperators
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
List <ProductInventoryAmount> InventoryDemo = new List<ProductInventoryAmount>();
InventoryDemo.Add(new ProductInventoryAmount
{
CategoryName = "Desktop Computer Accessories",
ProductName = "Keyboard",
ProductQuantity = 200,
ProductOrder = 300
});
InventoryDemo.Add(new ProductInventoryAmount
{
CategoryName = "Laptop Accessories",
ProductName = "Laptop Speakers",
ProductQuantity = 200,
ProductOrder = 100
});
InventoryDemo.Add(new ProductInventoryAmount
{
CategoryName = "Laptop Accessories",
ProductName = "Laptop Speakers",
ProductQuantity = 200,
ProductOrder = 100
});
InventoryDemo.Add(new ProductInventoryAmount
{
CategoryName = "Printers",
ProductName = "Wireless Printer",
ProductQuantity = 200,
ProductOrder = 200
});
InventoryDemo.Add(new ProductInventoryAmount
{
CategoryName = "Printers",
ProductName = "Wireless Printer",
ProductQuantity = 300,
ProductOrder = 500
});
// Using Linq aggregate functions to analyse inventory level
var InventoryStockProcessing =
from p in InventoryDemo
group p by p.ProductName into g
let maxProductOrder = g.Max(p => p.ProductOrder)
select new {
// Name of category
Category = g.Key,
// Quantity of product in inventory
TotalUnitsInStock = g.Sum(p => p.ProductQuantity),
// Quantity of orders related to product
TotalRequiredOrders = g.Sum(p => p.ProductOrder),
// Calculate the difference between product quantity in inventory and required quantity
Difference = g.Sum(p => p.ProductQuantity) - g.Sum(p => p.ProductOrder),
//Paying attention if we need to increase quantity to meet order
Attention = g.Sum(p => p.ProductQuantity) < g.Sum(p => p.ProductOrder) ? true : false,
//Show the maximum and the minimum product order
MaximumOrderQuantity = g.Max(p => p.ProductOrder),
MinimumOrderQuantity = g.Min(p => p.ProductOrder) };
// Show data in dataGridView1
dataGridView1.DataSource = InventoryStockProcessing.ToList();
}
}
public class ProductInventoryAmount
{
public ProductInventoryAmount()
{
}
// Automatic properties of ProductInventoryAmount
public string CategoryName { get; set; }
public string ProductName { get; set; }
public int ProductQuantity { get; set; }
public int ProductOrder { get; set; }
}
}