Introduction
This article explains about how to minimize performance degradation caused by frequently used complex regex using compiled Regular Expressions.
Prerequisites:
C# language,RegEx
Technologies:
.Net 2.0/3.5
Implementation
First of all what is Compiled RegEx???
Well when you create normal RegEx object of complex regex object and want to compare that pattern with some text every time its interpreted by MSIL and then pattern is matched now if we have complex regular expression to match and if it's used frequently it will obvious that it will affect the performance of application.
So to overcome this problem we can use compiled Regular expressions that will be compiled at compile time to MSIL then it will not interpreted again and again in MSIL . That will result in faster execution of regex object.
However several disadvantages also of this compiled time Regex like JIT need to do more work .once application is compiled we cannot unload the Regex .from memory to reclaim it.
Now back to the Point how to create Compiled Regex.
As Example let's make regex Compiled regex object that will match small case word in beginning of string.
Here for making Compiled Regex we need to Set RegexOptions.Compiled option while creating Compiled Regex.
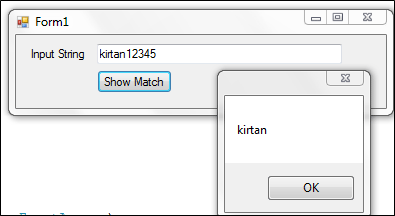
using System.Text.RegularExpressions;
private void btnShowMatch_Click(object sender, EventArgs e)
{
string InputString = txtInputString.Text;
Regex rex = new Regex("^[a-z]*", RegexOptions.Compiled);
Match m = rex.Match(InputString);
//Show Match in Message Box
MessageBox.Show(m.Value.ToString());
}
Conclusion
This article shows how to make use of compiled Regular Expressions to gain performance over non compiled Regular Expressions.