This article has been excerpted from book "Graphics Programming with GDI+".
Typography Namespace
In the .NET framework library, two namespaces define the font-related functionality: System.Drawing and System.Drawing.Text .The System.Drawing namespace contains general typography functionality, and System.Drawing.Text contains advanced typography functionality. Before using any of the typography-related classes in your application, you must include the appropriate namespace. We will discuss advanced typography in section 5.6.
The font class provides functionality for fonts, including methods and properties to define functionalities such as font style, size, name, and conversions. Before we discuss the Font class, we will introduce the FontStyle enumeration and the FontFamily class, which we will use to create Font objects.
The FontStyle Enumeration
The FontStyle enumeration defines the common style of a font. The member of FontStyle are described in Table 5.4
The FontFamily Class
The FontFamily class provides methods and properties to work with font families. Table 5.5 describes the properties of the FontFamily class.
TABLE 5.4 FontStyle members
Member |
Description |
Bold |
Bold text |
Italic |
Italic text |
Regular |
Normal text |
Strikeout |
Text with a line through the middle |
Underline |
Underlined text |
TABLE 5.5: FontFamily Properties
Property |
Description |
Families |
Returns an array of all the font families associated with the current graphics context. |
GenericMonospace |
Returns a monospace font family |
GenericSansSerif |
Returns a sans serif font family |
GenericSerif |
Returns a serif font family |
Name |
Returns the name of a font family |
Table 5.6 describes the methods of the FontFamily class.
Table 5.6 introduces some new terms, including base line, ascent, and descent. Let's see what they mean. Figure 5.10 shows a typical font in windows. As you can see, although the letters b and q are the same size, their starting points and ending points (top and bottom locations) are different. The total height of a font-including ascent, descent, and extra space-is called the line spacing. Ascent is the height above the base line, and decent is the height below the base line. As figure 5.10 shows, two characters may have different positions along the base line. For some fonts, the extra value is 0, but others it is not.
For some fonts, line spacing is some of the ascent and descent. Listing 5.6 create a new fonts uses get the values of line spacing, ascent, and descent and, calculates the extra space by subtracting ascent and descent from the line space. The following list identifies the get methods of a FontFamily object:
- GetCellAscent returns the cell ascent, in font design units.
- GetCellDescent returns the cell descent, in font design units.
- GetCelEmHeight returns the em height, in font design units.
- GetLineSpacing returns the line spacing for font family
Figure 5.10: Font metrics
In addition to these get methods, the Font object class provides GetHeight, which returns the height of a Font object.
As Listing 5.6, shows, we use GetLineSpacing, GetLineAscent, GetLineDescent, and GetEmHeight to get line spacing, ascent, descent and font height, respectively, and than we display the output in massage box.
TABLE 5.6 FontFamily methods
Method |
Description |
GetCellAscent |
Returns the cell ascent, in font design units of a font family. |
GetCellDescent |
Returns the cell descent, in font design units of a font family. |
GetEmHeight |
Returns the height, in font design units of the em square for the specified style. |
GetFamilies |
Returns an array that contains all font families available for a graphics object. This method takes an argument of Graphics type. |
GetLineSpacing |
Returns the amount of space between two consecutive lines of text for a font family. |
GetName |
Return the name, in the specified language, of a font family. |
IsStyleAvialable |
Before applying a style to a font, you may want to know whether the font family in question supports that style. This method returns true if a font style is available. For example, the following code snippet checks whether or not the Arial font family supports italics:
FontFamily ff =new FontFamily ('Arial");
if (ff.IsStyleAvailable(FontStyle.Italic)) //do something |
LISTING 5.6 Getting line spacing, ascending, descending and fontheight
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Paint(object sender, PaintEventArgs e)
{
//Create a Graphocs object
Graphics g = this.CreateGraphics();
//Create a font object
Font fnt = new Font("Verdana", 10);
//Get height
float lnSpace = fnt.GetHeight(g);
//Get line spacing
int cellSpace = fnt.FontFamily.GetLineSpacing(fnt.Style);
//get cell ascent
int cellAscent = fnt.FontFamily.GetCellAscent(fnt.Style);
//Get cell descent
int cellDescent = fnt.FontFamily.GetCellDescent(fnt.Style);
//Get font height
int emHeight = fnt.FontFamily.GetEmHeight(fnt.Style);
//Get free space
float free = cellSpace - (cellAscent + cellDescent);
//Display values
string str = " Cell Height :" + lnSpace.ToString() + ", Line Spacing: " + cellSpace.ToString() + " Ascent : " +
cellAscent.ToString() + " Decent :" +
cellDescent.ToString() + ",Free:" + free.ToString() +
" EM Height:" + emHeight.ToString();
MessageBox.Show(str.ToString());
//Dispose of objects
fnt.Dispose();
g.Dispose();
}
}
}
Figure 5.11 shows the output from Listing 5.6 we get cell height, line spacing, ascent, descent, free (extra) space, and em height.
The GraphicsUnit Enumeration
You can define the unit of measure of a font when you construct a Font object. The Font class constructor takes an argument of type GraphicsUnit enumeration, which specifies the unit of measure of a font. The default unit of measure for fonts is the point (1/72 inch) .you can get the current unit of a font by using the unit property of the font class .The following code snippet returns the current unit of the font:
Font fnt = new Font(" Verdana", 10);
MessageBox.Show(fnt.Unit.ToString());
The members of the GraphicsUnit enumeration are described in Table 5.7
FIGURE 5.11 Getting line spacing, ascent, descent free (extra) space, and height of a font
TABLE 5.7 GraphicsUnit members
Member |
Unit of Measure |
Display |
1/75 inch |
Document |
1/300 inch |
Inch |
1 inch |
Millimeter |
1 Millimeter |
Pixel |
1 pixel |
Point |
1/72 inch |
World |
The world unit |
Conclusion
Hope the article would have helped you in understanding Font in .NET in context of GDI classes. Read other articles on GDI+ on the website.
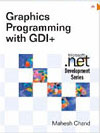
|
This book teaches .NET developers how to work with GDI+ as they develop applications that include graphics, or that interact with monitors or printers. It begins by explaining the difference between GDI and GDI+, and covering the basic concepts of graphics programming in Windows. |