Introduction
Caching is a technique of storing a copy of data in memory. For example, you may cache the a page or the results of a query. The advantage of caching is to build better performance into your application. Accessing cached data in memory is much faster than re-building a page or re-querying the database.
There are two types of caching in asp.net 2.0:
- Output Caching
- Data Caching
1. Output Caching
Output caching stores an HTML page in a cache that will not refresh until your specified duration time passes.
<%@ OutputCache Duration="30" VaryByParam="None" %>.
Duration -> Allows you to specify time in seconds.
VaryByParam -> Output based on query string.
<%@ OutputCache Duration="30" VaryByParam="UserID" %>.
Result
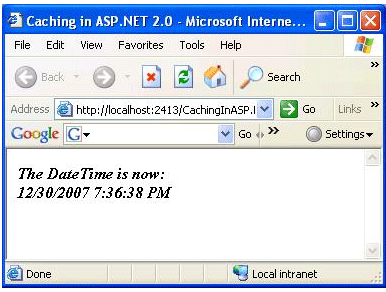
The date/time will refresh automatically after 30 seconds if you put the following code on a page load event.
protected void Page_Load(object sender, EventArgs e)
{
LabelDate.Font.Bold = true;
LabelDate.Font.Italic = true;
LabelDate.Text = "The DateTime is now:<br/>";
LabelDate.Text += DateTime.Now.ToString();
}
Put this line on top of your .aspx page and set your duration time as desired.
<%@ OutputCache Duration="30" VaryByParam="None" %>.
2. Data Caching
Data caching is the most important type of caching. The basic principle of data caching is that you can add items in your collection object called Cache.
Example
protected void Page_Load(object sender, EventArgs e)
{
LabelData.Font.Bold = true;
LabelData.Font.Italic = true;
if (Cache["RajCacheItems"] == null)
{
LabelData.Text += "Raj creating items.....<br />";
DateTime dateTime = DateTime.Now;
LabelData.Text += "Storing items in cache ";
LabelData.Text += "for 50 seconds.<br />";
Cache.Insert("RajCacheItems", dateTime, null, DateTime.Now.AddSeconds(50), TimeSpan.Zero);
}
else
{
LabelData.Text += "Accessing RajCacheItems.....<br />";
DateTime dateTime = (DateTime)Cache["RajCacheItems"];
LabelData.Text += "RajCacheItems is '" + dateTime.ToString();
}
}
Result
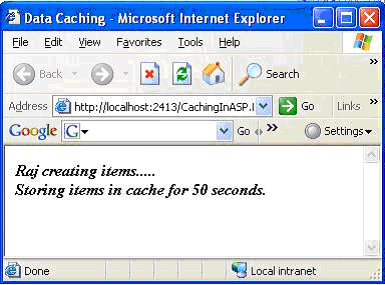
Data Source Control Caching
This is the caching that is built into the data source controls like SqlDataSource, ObjectDataSource and XmlDataSource.
There are four properties of caching in SqlDataSource:
-
Enable Caching: You need to set the enable caching property to true; the default value is false.
-
Cache Expiration Policy: Absolute and sliding.
-
Cache Duration: Duration time in seconds.
-
CacheKeyDependency: Makes a cached item dependent on another item in the data cache or on a table in your database.
Example
<asp:SqlDataSource ID="SqlDataSource1" runat="server" ConnectionString="<%$ ConnectionStrings:TestConnectionString %>"
SelectCommand="SELECT [Id], [FirstName], [LastName], [Address], [City], [State], [Country] FROM [Records] WHERE ([City] = @City)"
EnableCaching="True" CacheExpirationPolicy="Sliding" CacheDuration="20">
<SelectParameters>
<asp:ControlParameter ControlID="DropDownList1" Name="City" PropertyName="SelectedValue" Type="String" />
</SelectParameters>
</asp:SqlDataSource>
Result
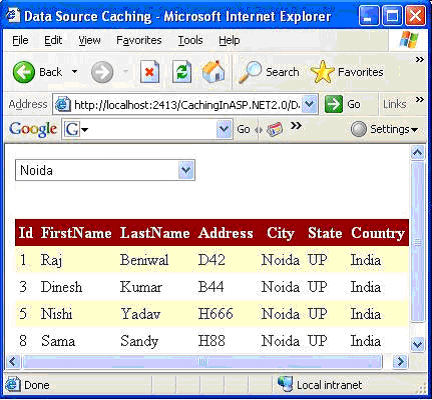