This article demonstrates how to use ChildWindow in Windows Phone 7. ChildWindow is like a popup window or message box which is used to display information or content. A ChildWindow always displays in a model popup that blocks user interaction with the underlying user interface.
A ChildWindow has three distinct areas inside its Root part:
Overlay - the part of the child window that covers the parent window.
Chrome - the part of the child window which hosts the Title and CloseButton parts.
ContentRoot - the part of the child window which hosts application-specific content in its ContentPresenter part.
Getting Started
Creating a Windows Phone Application:
- Open Visual Studio 2010.
- Go to File => New => Project
- Select Silverlight for Windows Phone from the Installed templates and choose Windows Phone Application
- Enter the Name and choose the location.
Click OK.
First of all add a new assembly reference (System.Windows.Controls) then add a new Windows Phone User Control using add new item. Looks like this.
<UserControl x:Class="WPChildWindow.WindowsPhoneControl1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
FontFamily="{StaticResource PhoneFontFamilyNormal}"
FontSize="{StaticResource PhoneFontSizeNormal}"
Foreground="{StaticResource PhoneForegroundBrush}"
d:DesignHeight="480" d:DesignWidth="480">
<Grid x:Name="LayoutRoot" Background="{StaticResource PhoneChromeBrush}">
</Grid>
</UserControl>
Now add System.Windows.Controls namespace on .xaml control and rename user control name to child window like this.
<tk:ChildWindow x:Class="WPChildWindow.LoginChildWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:tk="clr-namespace:System.Windows.Controls;assembly=System.Windows.Controls"
mc:Ignorable="d"
Title="Login" Closing="ChildWindow_Closing"
OverlayBrush="#7F000000"
BorderBrush="Black"
BorderThickness="2"
FontFamily="{StaticResource PhoneFontFamilyNormal}"
FontSize="{StaticResource PhoneFontSizeNormal}"
Foreground="Orange"
d:DesignHeight="256" d:DesignWidth="480">
<Grid x:Name="LayoutRoot" Background="#FF3B6261" Height="202">
<TextBlock HorizontalAlignment="Left" TextWrapping="Wrap" Text="User Id: " VerticalAlignment="Top" Margin="20,43,0,0"/>
<TextBlock HorizontalAlignment="Left" TextWrapping="Wrap" Text="Password: " VerticalAlignment="Top" Margin="20,104,0,0"/>
<TextBox x:Name="txtUserId" VerticalAlignment="Top" Margin="117,23,8,0" Height="62" FontSize="18.667"/>
<PasswordBox x:Name="txtPassword" VerticalAlignment="Top" Margin="117,85,8,0" Height="62" FontSize="18.667"/>
<Button x:Name="btnLogin" Content="Login" Margin="117,132,214,0" d:LayoutOverrides="Width" Click="btnLogin_Click" FontSize="18.667" BorderBrush="#FFC06161" BorderThickness="1" Foreground="#FFE46767" Background="#FF562D29" Height="58" VerticalAlignment="Top" FontFamily="Tahoma"/>
<Button x:Name="btnCancel" Content="Cancel" HorizontalAlignment="Left" Margin="232,132,0,0" VerticalAlignment="Top" FontSize="18.667" BorderBrush="#FFC06161" BorderThickness="1" Foreground="#FFE46767" Background="#FF562D29" Height="58" FontFamily="Tahoma" Click="btnCancel_Click" />
</Grid>
</tk:ChildWindow>
Change ChildWindow base class too on cs page.
public partial class LoginChildWindow : ChildWindow
{
public LoginChildWindow()
{
InitializeComponent();
}
private void btnLogin_Click(object sender, RoutedEventArgs e)
{
this.DialogResult = true;
}
private void ChildWindow_Closing(object sender, System.ComponentModel.CancelEventArgs e)
{
if (this.DialogResult == true &&
(this.txtUserId.Text == string.Empty || this.txtPassword.Password == string.Empty))
{
e.Cancel = true;
ChildWindow cw = new ChildWindow();
cw.Height = 100;
cw.Width = 300;
cw.Content = "Please enter userid and password or click Cancel.";
cw.Show();
}
}
private void btnCancel_Click(object sender, RoutedEventArgs e)
{
this.DialogResult = false;
}
}
Now add a new button control on main page and fire click event and put this code.
private void button1_Click(object sender, RoutedEventArgs e)
{
LoginChildWindow loginWindow = new LoginChildWindow();
loginWindow.Show();
}
Result should be like this.
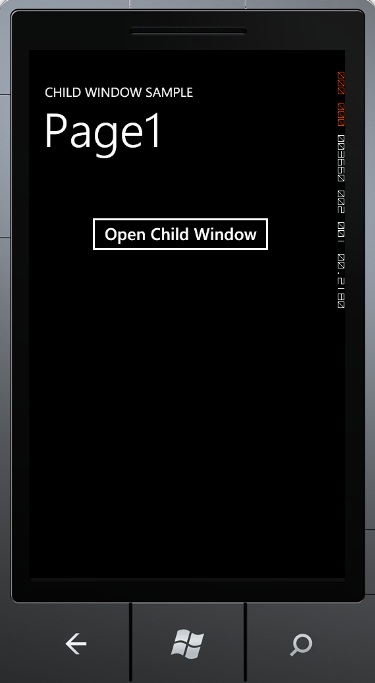
Image 1.
Image 2.
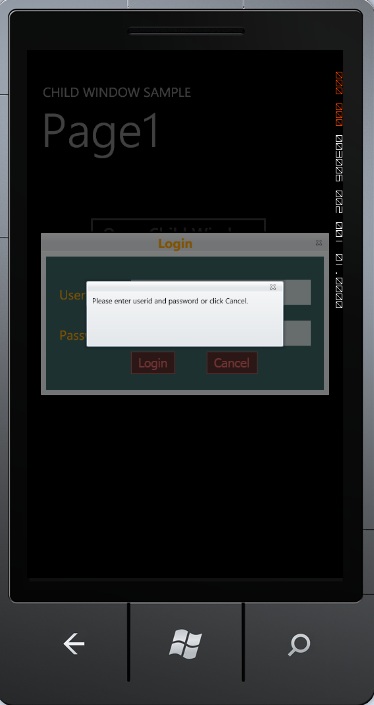
Image 3.