Introduction:
We will be learning how to develop a Web Part that loads in a SharePoint site. To begin creating a web part:
- VS.NET 2005 installed.
- "Web Part Project Library" installed on your system.
- Start VS.NET 2005 and create a new project.
- Select Project Type as Visual C#-->SharePoint.
- Visual Studio Installed templates as Web Part.
- Change Name to Web Part Basics.
- Change Location to e:\Webpartbasics (or appropriate drive letter).
- Change Solution name to FileUploadWebPart.
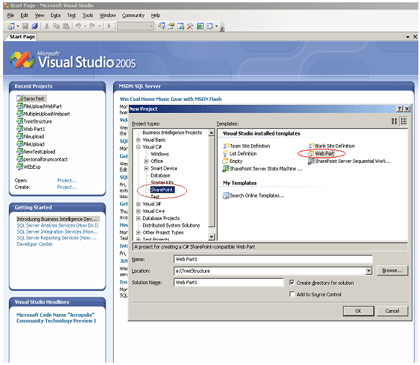
The Render Method
The Web Part base class overrides the Render method of System.Web.UI.Control because the Web Part infrastructure needs to control the rendering of the Web Part contents. For this reason custom Web Parts must override the Render method of the Web part base class.
The Complete Web Part Code
/*Creation Log**********************************************************<?xml:namespace prefix = o ns = "urn:schemas-microsoft-com:office:office" />
* Author : Saravanan Gajendran
* Creation Date : 12-01-2008
* FileName : Webpartbasics.cs
* Class : Webpartbasics
* Description : Tracking the web part life cycle.
A Web Part is an add-on ASP.NET technology to Windows SharePoint Services
***********************************************************************/
using System;
using System.Web.UI;
using System.Xml.Serialization;
using System.Runtime.InteropServices;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.WebControls;
using Microsoft.SharePoint;
using Microsoft.SharePoint.WebControls;
using Microsoft.SharePoint.WebPartPages;
namespace Webpartbasics
{
[Guid("c07e5146-b025-4f9b-b0a4-a0fc5cb2cfd6")]
public class Webpartbasics : System.Web.UI.WebControls.WebParts.WebPart
{
//varible declaration
private string _strResult;
private TextBox _tbxText;
private Button _btnShow;
private Label _lblErrMsg;
/// <summary>
/// 1 st EVENT IN WEB PART MANAGER
/// During the initialization, configuration values that were marked as webbrowsable
/// and set through the webpart task pane are loaded to the webpart
/// </summary>
/// <param name="e"></param>
protected override void OnInit(EventArgs e)
{
try
{
this._strResult += "onInit Method <br>";
base.OnInit(e);
}
catch (Exception ex)
{
this._lblErrMsg.Text = ex.Message.ToString();
}
}
/// <summary>
/// 2 nd EVENT IN WEB PART MANAGER
///
/// Viewstate is a property inherited from System.Web.UI.Control .
/// The viewstate is filled from the state information that was previously serialized
/// would like to persist your own data within webpart
/// </summary>
/// <param name="savedState"></param>
protected override void LoadViewState(object savedState)
{
try
{
_strResult += "LoadViewState<br>";
object[] viewstate = null;
if (savedState != null)
{
viewstate = (object[])savedState;
base.LoadViewState(viewstate[0]);
_strResult += (string)viewstate[1] + "<br>";
}
}
catch (Exception ex)
{
this._lblErrMsg.Text = ex.Message.ToString();
}
} /// <summary>
/// 3 rd EVENT IN WEB PART MANAGER
/// All of the constituent controls are created and added to the controls collection
/// </summary>
protected override void CreateChildControls()
{
try
{
_strResult += "CreateChildControls<br>"; //creating error label controls
this._lblErrMsg = new Label();
this._lblErrMsg.ID = "lblErrMsg";
this._lblErrMsg.Text = string.Empty;
this.Controls.Add(_lblErrMsg); //creating text controls
this._tbxText = new TextBox();
this._tbxText.ID = "tbxText";
this._tbxText.Text = string.Empty;
this.Controls.Add(_tbxText); //creating button controls
this._btnShow = new Button();
this._btnShow.ID = "btnShow";
this._btnShow.Text = "Check Text Value";
this._btnShow.Click += new EventHandler(_btnShow_Click);
this.Controls.Add(_btnShow);
}
catch (Exception ex)
{
this._lblErrMsg.Text = ex.Message.ToString();
}
}
/// <summary>
/// butoon click event
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void _btnShow_Click(object sender, EventArgs e)
{
try
{
this._strResult += "button click event has fired<br>";
}
catch (Exception ex)
{
this._lblErrMsg.Text = ex.Message.ToString();
}
}
/// <summary>
/// 4 th EVENT IN WEB PART MANAGER
/// Perform actions common to all requests, such as setting up a database query
/// At this point, server controls in the tree are created and initialized, the state is
restored,
/// and form controls reflect client-side data.
/// </summary>
/// <param name="e"></param>
protected override void OnLoad(EventArgs e)
{
try
{
this._strResult += "Onload<br>";
base.OnLoad(e);
}
catch (Exception ex)
{
this._lblErrMsg.Text = ex.Message.ToString();
}
}
/// <summary>
/// 5 th EVENT IN WEB PART MANAGER
/// Perform any updates before the output is rendered.
/// Any changes made to the state of the control in the pre-render phase can be
saved
/// while changes made in the rendering phase are lost.
/// </summary>
/// <param name="e"></param>
protected override void OnPreRender(EventArgs e)
{
try
{
this._strResult += "OnPreRender<br>";
base.OnPreRender(e);
}
catch (Exception ex)
{
this._lblErrMsg.Text = ex.Message.ToString();
}
}
/// <summary>
/// 6 th EVENT IN WEB PART MANAGER
/// store cutom data within a web part's viewstate
/// The ViewState property of a control is automatically persisted to a string
object after this stage.
/// This string object is sent to the client and back as a hidden variable.
/// For improving efficiency, a control can override
/// the SaveViewState method to modify the ViewState property.
/// once viewstate is saved,the control webpart can be removed from the memory
of the server.
/// webpart receives notification that they are about removed from memory
through dispose event
/// </summary>
/// <returns></returns>
protected override object SaveViewState()
{
this._strResult += "SaveViewState<br>";
object[] viewstate = new object[2]; viewstate[0] = base.SaveViewState();
viewstate[1] = "MyTestData";
return viewstate;
} /// <summary>
/// 7 th EVENT IN WEB PART MANAGER
/// Generate output to be rendered to the client.
/// you can create user interface of your webpart using html table.
/// You can apply your css classes here itself
/// </summary>
/// <param name="writer"></param>
public override void RenderControl(HtmlTextWriter writer)
{
try
{
//this method ensure all created child controls
EnsureChildControls();
this._strResult += "RenderControl<br>";
//table start
writer.Write("<table id='tblTest'align='center' cellpadding='0' cellspacing='0'
border='1' width='100%'>");
writer.Write("<tr>");
writer.Write("<td>");
writer.Write(_strResult);
writer.Write("</td>");
writer.Write("</tr>"); //row 2
writer.Write("<tr>");
writer.Write("<td>");
this._tbxText.RenderControl(writer);
writer.Write("</td>");
writer.Write("</tr>"); //row 3
writer.Write("<tr>");
writer.Write("<td>");
this._btnShow.RenderControl(writer);
writer.Write("</td>");
writer.Write("</tr>"); //row 4
writer.Write("<tr>");
writer.Write("<td>");
this._lblErrMsg.RenderControl(writer);
writer.Write("</td>");
writer.Write("</tr>");
writer.Write("</table>");
//table end
}
catch (Exception ex)
{
this._lblErrMsg.Text = ex.Message.ToString();
}
}
/// <summary>
/// 8 th EVENT IN WEB PART MANAGER
///
/// Perform any final cleanup before the control is torn down.
/// References to expensive resources such as database connections must be
/// released in this phase.
/// </summary>
public override void Dispose()
{
base.Dispose();
}
/// <summary>
/// 9 th EVENT IN WEB PART MANAGER
///
/// Perform any final cleanup before the control is torn down.
/// Control authors generally perform cleanup in Dispose and do not handle this
event
/// The webpart removed from memory of the server
/// Generally webpart developer do not need access to this event because all
/// the clean up should have been accomplish in dispose event
/// </summary>
/// <param name="e"></param>
protected override void OnUnload(EventArgs e)
{
base.OnUnload(e);
}
}
}
Deploy File Upload Web Part in the SharePoint Server
- Right click solution file then select properties.
- Select Debug --> Start browser with URL selects your respective SharePoint Site.
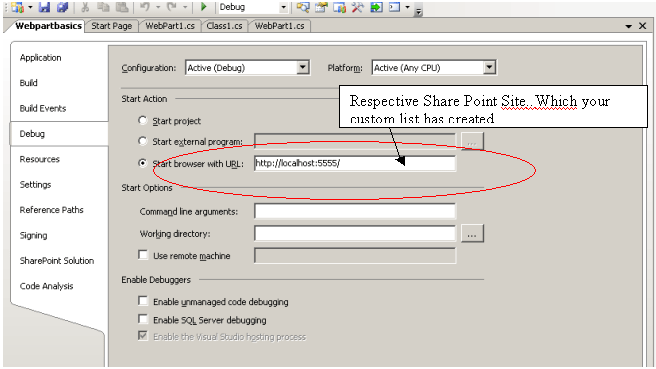
Select Signing Option
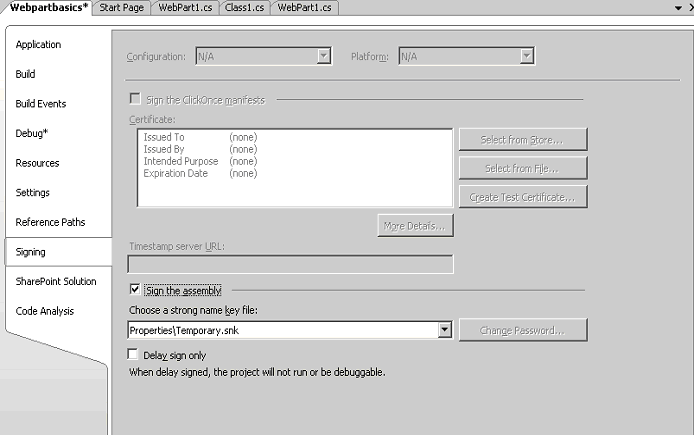
- Ensure the strong name key and sign the assembly check box.
- Right Click properties and select Deploy.
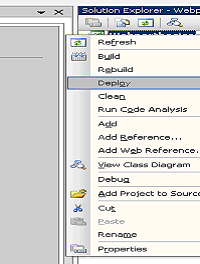
- The Web Part is automatically deployed to the respective site.
- Deploy will take care of .Stop IIS, buliding solution, restarting solution, creating GAC, restarting IIS like that.
- Now you should have this web part in your SharePoint page.
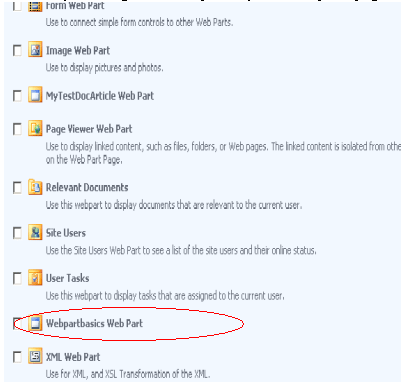
The final web part is added to the site. All events displayed in web part have order.
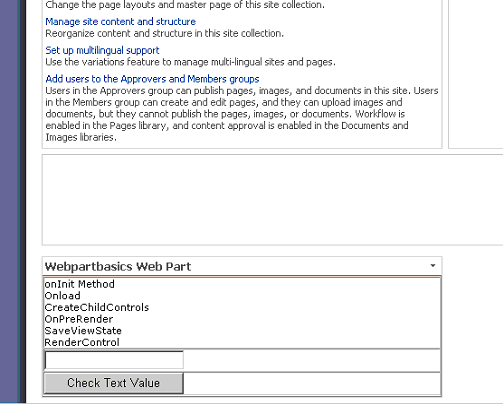