In most of our machines, there are few things running in background called as services. We can view the services running on our machine by typing services.msc in Run of Start command. Windows provides a built-in explorer for viewing the services of our machine. But, it won't provide easy navigation. So, I thought of doing an application that makes our services exploration easy and fast. First, I will explain the features of this application followed by its design and coding.
Features provided by this Explorer:
-
Easy to navigate among the services.
-
Easy to filter services based on search criteria.
-
Start/Stop services.
-
Uninstall a service.
-
Remote Machine services Viewing.
-
Show services dependent on a particular service
-
Show Dependencies.
-
Better User Experience and lot more.
I designed this application in VS 2008 SP1. Create a new Windows application in C# and name it as EnhancedServiceExplorer. Add the controls to ServiceExplorer form as shown below:
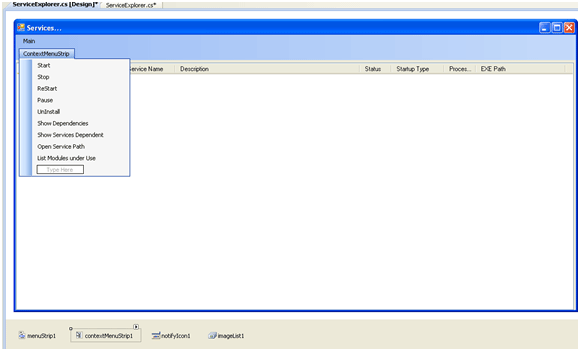
Purpose of above controls:
-
ListView (lstServices) -> To Display list of services.
-
Context Menu(contextMenuStrip1) ->To change status of a service and see service dependencies.
-
Image List (imageList1) ->To display icon for services in List View.
-
Main Menu (menuStrip1) -> To connect a remote machine, refresh services. and exit application.
-
Notify Icon (notifyIcon1) ->To show/hide the Explorer.
We are done with the design. Now, let's dig into the functionality part of this application. I will outline the steps performed by it.
-
When we run the application, it will load all the services of our machine.
-
We can start/stop/uninstall any service.
-
We can view list of dependencies on a service.
-
We can connect to a remote machine and get their services list by entering machine name or IP Address in "Connect To" text box followed by an Enter. We need permissions on a machine, to get services list from it.
-
We can filter services by entering search criteria.
Let's go into the coding part. I will outline the important methods.
-
LoadServiceItems -> This method loads all the services of a machine on a separate thread to improve the performance.
-
UpdateOrUninstallService -> This method is used start/stop/uninstall a service.
-
ShowDependencies -> Highlights the service's dependencies.
-
ShowServicesDependent -> Highlights the services dependent on a service.
I will explain the core method (LoadServiceItems) for loading services. In order to make application respond to user interactions while loading services, I had implemented loading on a separate thread using ThreadStart and Thread classes.
private void LoadServiceItems(string machinepath)
{
try
{
ManagementPath path = new ManagementPath();
ServicesList.Clear();
if (machinepath == "")
{
path.Server = System.Environment.MachineName;
MCName = "";
}
else
{
path.Server = machinepath;
MCName = machinepath;
}
path.ClassName = "Win32_Service";
path.NamespacePath = @"\root\cimv2";
ManagementClass serviceClass = new ManagementClass(path);
foreach (ManagementObject ob in serviceClass.GetInstances())
{
ListViewItem item1 = new ListViewItem();
Services objService = new Services();
item1.StateImageIndex = 0;
item1.Text = objService.DisplayName = ob.GetPropertyValue("DisplayName") == null ? "" : ob.GetPropertyValue("DisplayName").ToString();
objService.ServiceName = ob.GetPropertyValue("Name") == null ? "" : ob.GetPropertyValue("Name").ToString();
item1.SubItems.Add(objService.ServiceName);
objService.ServiceDesc = ob.GetPropertyValue("Description") == null ? "" : ob.GetPropertyValue("Description").ToString();
item1.SubItems.Add(objService.ServiceDesc);
objService.Status = ob.GetPropertyValue("State") == null ? "" : ob.GetPropertyValue("State").ToString();
item1.SubItems.Add(objService.Status);
objService.StartMode = ob.GetPropertyValue("StartMode") == null ? "" : ob.GetPropertyValue("StartMode").ToString();
item1.SubItems.Add(objService.StartMode);
objService.ProcessID = ob.GetPropertyValue("ProcessId") == null ? "" : ob.GetPropertyValue("ProcessId").ToString();
item1.SubItems.Add(objService.ProcessID);
objService.EXEPath = ob.GetPropertyValue("PathName") == null ? "" : ob.GetPropertyValue("PathName").ToString();
item1.SubItems.Add(objService.EXEPath);
item1.ToolTipText = objService.ServiceDesc;
m_addserviceItem = new addServiceItem(this.AddServiceItem);
this.Invoke(m_addserviceItem, new object[] { item1 });
ServicesList.Add(objService);
}
//Indication of services loaded completely.
m_addserviceItem = new addServiceItem(this.AddServiceItem);
this.Invoke(m_addserviceItem, new object[] { new ListViewItem("!!!!") });
}
catch { }
}
Here, we are getting list of services on a machine using ManagementClass object's GetInstances() by setting ManagementPath properties with machine name, path etc.
Than, we are looping through that services list and added to List view.
We are doing start/stop/uninstall of a service using ManagementObject's Invoke(). Finally, we are getting list of service's dependencies using ServiceController's DependentServices collection.
I added some more code to improve the UI and functionality. Finally, the application looks like below:
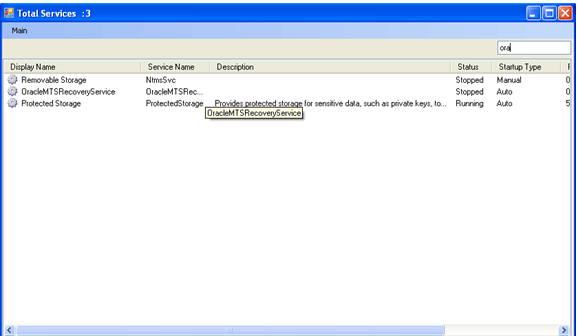
We can still enhance this application by adding UI and extra functionality like listing files used by a service, better performance etc. I am attaching source code for reference. I hope this article will be helpful for all.