Java bean: It is a development component for performing a task in an application. Any ordinary java class with a set of variables and methods can be called as javabean. The methods present in a java bean must follow a naming convention. A java bean can be used in a web application to store the state of the users.
Creation process of a java bean:
- Create a class in a package as the bean class.
- Provide the required variables as the properties.
- Provide setter and getter methods for each of the variables.
- Store the package folder inside a classes folder.
- Compile the file as ordinary java file.
Store the following bean file in (E:\Servlet\WEB-INF\classes\pack1)
Example:
package pack1;
public class mybean
{
private String name,pass;
public void setName(String n)
{
name=n;
}
public void setPass(String p)
{
pass=p;
}
public String getName()
{
return name;
}
public String getPass()
{
return pass;
}
}
Compile of bean:- javac mybean.java
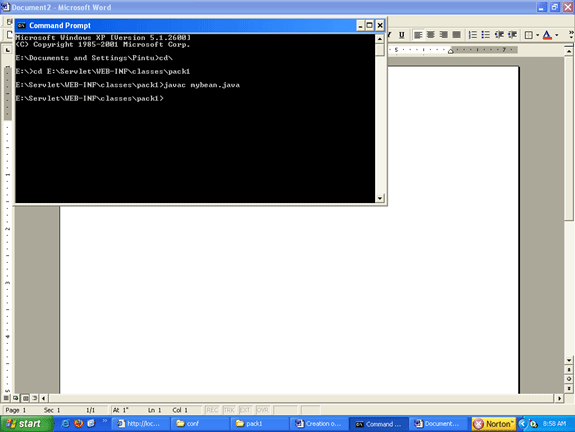
How to use this bean in JSP
- Use <jsp:useBean>; this tag creates an instance of the javabean in a JSP.
Attribute
Class- this contains name of the bean class with its package.
Id-this contains name of the bean object.
Scope-this is an optional attribute. This can contain a value such as page/request/session/application.
- Use <jsp:setProperty>; this tag calls setter methods of the bean.
Attribute
Name-this contains name of the bean object.
Property-this contains name of the property whose setter method required to be called.
Value-this contains value of the property to be assigned.
- Use <jsp:getProperty>; this tag can be used to call getter methods of the bean and to print the return value into the response.
Attribute
Name-this contains name of the bean object.
Property-this contains name of the property whose getter method required to be called.
Program beantest.jsp
Save the following beantest.jsp file in your context folder (E:\Servlet)
<html>
<body bgcolor="yellow">
<p> <h3><center>Please enter your user name and
password</center></h3></p>
<form>
<center>username</center>
<center><input type ='text' name ="t1"></center>
<center>Password</center>
<center><input type ='password' name ="p1"></center>
<center><input type ='submit' value="submit" ></center>
</form>
<jsp:useBean class="pack1.mybean" id="mb1" />
<%
String s1=request.getParameter("t1") ;
String s2=request.getParameter("p1") ;
if(s1!= null){ %>
<jsp:setProperty name="mb1" property="name" value="<%= s1 %>" />
<jsp:setProperty name="mb1" property="pass" value="<%= s2 %>" />
<h2>Name is :<jsp:getProperty name="mb1" property="name" /></h2>
<h2>Password is :<jsp:getProperty name="mb1" property="pass" /></h2>
<% } %>
</body>
</html>
Running the file
http://localhost:8082/javaservlet/beantest.jsp
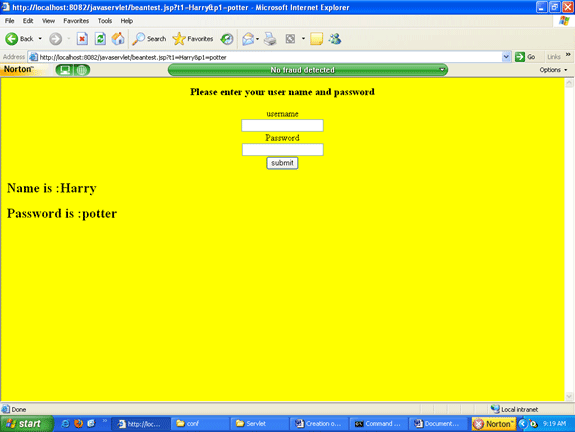