Introduction
In this tutorial we will try to understand how we can use interfaces and factory pattern to create a truly decoupled architecture framework. In this sample we will take up a simple three tier architecture and apply interfaces and factory pattern to see how we can transform the three tier in to a truly decoupled architecture.
Everyone's favorite the three tier architecture
Ok, everyone know what's a three tier architecture is. I will talk a bit about it and then we will get down to the issues related to three tier architecture. So basically as we all know in three tier architecture the UI code is in the client section, business object are nothing but business classes which has the business validation and the data access layer does all the database operations. Till here everything is good and nice.
If we analyze these three sections one of the important points to be noted is that business validations are highly volatile. Business rules change as the way of business changes with changing times.
The second volatile section is the client. People would like to have support for multiple clients depending on user interfaces. This is not so volatile as compared to business objects changes. Even if the customer wants to change its not on urgent basis. Business validations needs to be changed instantly.
Data access is the least volatile of all. At least in my whole project career I have still to come across project who wants to migrate from one database to other database frequently. There are times when there is a need but that's not urgent and is treated as a migration project.
So the conclusion business sections are the most volatile and when there is a change it needs to be changed instantly as compared to UI and back end databases.
Controlling change ripples
Below is a business class called as "clsInvoiceHeader". You can see in the code snippet the user interface is setting the value of the business object. So the user interface is directly consuming the class.
clsInvoiceDetail objInvoiceDetail = new clsInvoiceDetail();
objInvoiceDetail.CustomerName = txtCustomerName.Text; objInvoiceDetail.CustomerAddress = txtCustomerAddress.Text;
objInvoiceDetail.InvoiceComments = txtComments.Text;
objInvoiceDetail.InvoiceDate = Convert.ToDateTime(txtInvoiceDate.Text);
At the other end when the businesses object connects with the data access layer it's sending the values in a normal .NET data type. You can see how the "InsertInvoiceDetails" of the DAL component had normal .NET data types passed.
public class clsInvoiceDetail
{
public void Insert(int _InvoiceReference)
{
clsInvoiceDB objInvoiceDB = new clsInvoiceDB();
objInvoiceDB.InsertInvoiceDetails(_Id, _InvoiceReference, _ProductId, _Qty);
}
}
So what will happen if the business objects change?. It will just ripple changes across all the three layers. That means you need compile the whole project.
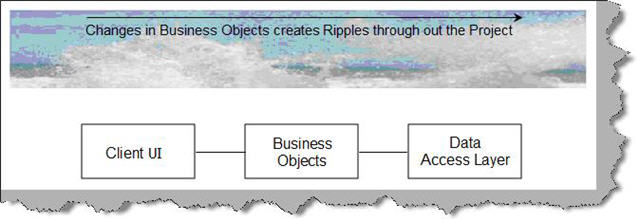
Magic of Interfaces and factory pattern
So how do we control those ripple effects across all the tiers? The answer to this is by interfaces and factory pattern. So let's first create an interface for "clsInvoiceDetail" class. Below is the Interface code for the same.
public interface IInvoiceDetail
{
string InvoiceReferenceNumber
{
get;
}
string CustomerName
{
set;
get;
}
string CustomerAddress
{
set;
get;
}
string InvoiceComments
{
set;
get;
}
DateTime InvoiceDate
{
set;
get;
}
}
Second we define a factory which creates the "clsInvoiceDetails" object. Below is the sample code for the same.
public class FactoryFinance
{
public static IInvoiceDetail getInvoiceDetail()
{
return new clsInvoiceDetail();
}
}
So the client only refers the interface which is the proxy and the factory creates the object of the concrete class "ClsInvoiceDetail". In this way the client never knows about the concrete class "ClsInvoiceDetail". The factory class is introduced to avoid the new keyword use in the client. If we have new keyword client in the UI then the UI needs to be direct contact with the concrete object which leads to heavy coupling.
IInvoiceDetails objInvoiceDetail = FactoryFinance.GetInvoiceDetails();
objInvoiceDetail.CustomerName = txtCustomerName.Text;
objInvoiceDetail.CustomerAddress = txtCustomerAddress.Text;
objInvoiceDetail.InvoiceComments = txtComments.Text;
objInvoiceDetail.InvoiceDate = Convert.ToDateTime(txtInvoiceDate.Text);
The other end i.e the database layer is also easy now. We need to pass the interface object in the database function rather than .NET primitive datatypes.
public class clsInvoiceDetail : IInvoiceDetail
{
public void UpDateToDatabase()
{
clsInvoiceDB objInvoiceDB = new clsInvoiceDB();
objInvoiceDB.InsertInvoiceDetails(this);
}
}
So here's what we have done the UI is isolated using factory and business object interface. The database layer is isolated using the interface. So in short when we change any logic we do not need to recompile the whole project and thus we have controlled the business logic ripple effect in the business logic project itself.
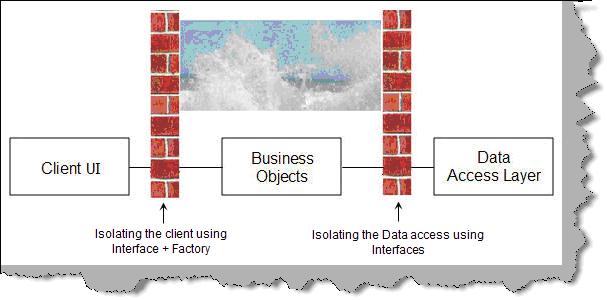
We have also uploaded the source code for an invoice project to demonstrate how the above decoupling works. One project is simple three tier architecture while the other shows how we have used the factory and interface to increase decoupling between the tiers.
Please find the Source code at the top of the article.