Overview
When you want to learn any new technology selecting right entry point is very important. In this article I have tried to give right starting point for Silverlight application development. You will learn how to start basic Silverlight application development using different development options available. I have started my first Silverlight application using notepad which is exiting and correct entry point for Silverlight application development, then you will learn what is happening behind the scene when you start using Visual Studio to create Silverlight application. You will also see how to develop Silverlight application using Visual Studio and detailed list of default files created by Silverlight application template. Finally you will see the life cycle of Silverlight application.
Creating Silverlight Application with Notepad
If you are new to Silverlight technology and want to learn how to start with Silverlight application development, I will encourage you to develop your first Silverlight application using notepad (not Visual studio or expression blend), so that you will completely understand what is visual studio 2008 or 2010 doing behind the scene when you create Silverlight application using Visual Studio 2008 project template. You might wondering how it is possible to start development with notepad without using any Microsoft development tools, yes it is possible, however you might not be writing your real life Silverlight application using notepad.
So you are ready for your first Silverlight applications, take a notepad and enter following code snippet and save it as .html extension. You might want to give meaning full name to your application; here I am call it as: SilverlightApplicationInNotePad.html
<html>
<head>
<title>This is my first Silverlight Application developed using Notepad</title>
</head>
<script type="text/javascript" >
// Create the MouseLeftButtonDownClick event handler for the root Canvas object.
function MouseLeftButtonDownClick(sender, args) {
var x = args.GetPosition(sender).x;
var y = args.GetPosition(sender).y;
// Retrieve a reference to the plug-in
var plugin = sender.getHost();
//Creates XAML content dynamically.
var textblockXaml = '<TextBlock Canvas.Left=" ' + x + '" Canvas.Top="' + y +
'" FontSize="18" Text="hello" Foreground="#CCCCCC"/>'
var textblock = plugin.content.CreateFromXaml(textblockXaml);
// Add the XAML fragment as a child of the root Canvas object.
sender.children.add(textblock);
}
</script>
<script type="text/xaml" id ="xamlsource">
<Canvas xmlns =http://schemas.microsoft.com/client/2007
Width="640"
Height="480"
Background="Black"
MouseLeftButtonDown="MouseLeftButtonDownClick">
<TextBlock Canvas.Left="4" FontSize="18" Text="My First Silverlight Application" Foreground="#CCCCCC"/>
<Ellipse Canvas.Left="20" Canvas.Top="50"
Fill="Orange" Width="200" Height="80" />
<Rectangle Canvas.Left="250" Canvas.Top="50" Width="80" Height="80" Fill="Red"/>
<Line Canvas.Left="20" Canvas.Top="100"
X1="20" Y1="20" X2="100" Y2="100" Stroke="Yellow" StrokeThickness="10"/>
<Polygon Canvas.Left="80" Canvas.Top="140"
Fill="blue" Stroke="Green" StrokeThickness="10"
Points="20,20 100,100 200,10"/>
<Path Canvas.Left="160" Canvas.Top="140"
Stroke="yellow" StrokeThickness="10">
<Path.Data>
<PathGeometry>
<PathFigure StartPoint="20,20">
<BezierSegment Point1="120,0" Point2="50,300" Point3="200,200"/>
</PathFigure>
</PathGeometry>
</Path.Data>
</Path>
</Canvas>
</script>
<body>
<object type="application/x-silverlight"
id="silverlightControl"
width="100%"
height="100%">
<param name="background" value="Yellow"/>
<param name="source" value="#xamlsource"/>
</object>
</body>
</html>
Output:
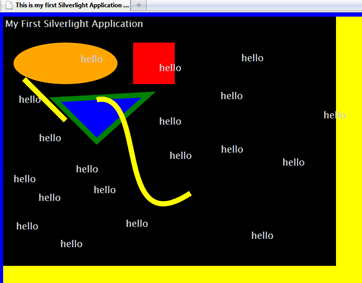
The output is so exiting isn't it? Silverlight is all about colorful animated media support rich internet application (RIA), ok Let's discuss step by step what is there inside the .html file, this walks through will help you to understand what is used for creating user interface (UI) and what is used for business logic (BL) and how to host a Silverlight application.
Step 1: - Hosting Silverlight application using HTML
There are different technologies available for hosting Silverlight application like HTML, ASP.NET, and PHP etc. In this application we will discuss how to host Silverlight application using HTML.
To host Silverlight application using HTML we need to use Object element which enables you to embed and configure the Silverlight plug-in in your HTML, this will help your browser to instantiate the plug in for Silverlight application.
There are two properties important to configure the plug in:
Type: you can specify the Silverlight version number using type attribute. Here I am not specifying any version so it will take available version installed in your PC.
Param: Parameter tag is required here to specify the XAML code reference. In this code I am using # (pound character) with ID, so that it will look xamlsource ID inside the same file.
<object type="application/x-silverlight"
id="silverlightControl"
width="100%"
height="100%">
<param name="background" value="Yellow"/>
<param name="source" value="#xamlsource"/>
</object>
Step 2: - Create User Interface using XAML code
XAML stands for Extensible Application Markup Language. It is a simple language based on XML to create and initialize .NET objects with hierarchical relations. Although it was originally invented for WPF, now we are using XAML for creating Silverlight UI too; here I am keeping everything in single html file just to make it simple. However you will be having separate file for xaml code and taking the xaml file reference to your html file using object element.
<Param> element name = Source in object element above is used to specific the ID called "xamlsource" for XAML code. Canvas is container tag holding some XAML elements for UI, I have specified an event called "MouseLeftButtonDown" when user clicks on left mouse button on Canvas it will call the JavaScript function to display some contents.
<script type="text/xaml" id ="xamlsource">
<Canvas xmlns =http://schemas.microsoft.com/client/2007
Width="640"
Height="480"
Background="Black"
MouseLeftButtonDown="MouseLeftButtonDownClick">
<TextBlock Canvas.Left="4" FontSize="18" Text="My First Silverlight Application" Foreground="#CCCCCC"/>
<Ellipse Canvas.Left="20" Canvas.Top="50"
Fill="Orange" Width="200" Height="80" />
<Rectangle Canvas.Left="250" Canvas.Top="50" Width="80" Height="80" Fill="Red"/>
<Line Canvas.Left="20" Canvas.Top="100"
X1="20" Y1="20" X2="100" Y2="100" Stroke="Yellow" StrokeThickness="10"/>
<Polygon Canvas.Left="80" Canvas.Top="140"
Fill="blue" Stroke="Green" StrokeThickness="10"
Points="20,20 100,100 200,10"/>
<Path Canvas.Left="160" Canvas.Top="140"
Stroke="yellow" StrokeThickness="10">
<Path.Data>
<PathGeometry>
<PathFigure StartPoint="20,20">
<BezierSegment Point1="120,0" Point2="50,300" Point3="200,200"/>
</PathFigure>
</PathGeometry>
</Path.Data>
</Path>
</Canvas>
</script>
Step 3: - Writing business logic using JavaScript
Again you will not be keeping java script code in the same file instead you can have .js file and take the reference of it into this .html file. This Browser supported interpreted unmanaged language is used to write some business logic. In real life application development you will be writing the code using managed programming languages like C# or VB.NET.
When the user clicks on his left mouse button, JavaScript code reads X, Y position of the Canvas surface and dynamically generates XAML code and render on that position.
The GetHost method retrieves the Silverlight plug-in instance that contains the object. This method is especially useful for retrieving the Silverlight plug-in in an input event-handling function in which the sender parameter is generally a DependencyObject.
Create XAML contents on the fly using CreateFromXaml method and add these elements to parent element of Canvas using sender.children.add method.
<script type="text/javascript" >
// Create the MouseLeftButtonDownClick event handler for the root Canvas object.
function MouseLeftButtonDownClick(sender, args) {
var x = args.GetPosition(sender).x;
var y = args.GetPosition(sender).y;
// Retrieve a reference to the plug-in
var plugin = sender.getHost();
//Creates XAML content dynamically.
var textblockXaml = '<TextBlock Canvas.Left=" ' + x + '" Canvas.Top="' + y +
'" FontSize="18" Text="hello" Foreground="#CCCCCC"/>'
var textblock = plugin.content.CreateFromXaml(textblockXaml);
// Add the XAML fragment as a child of the root Canvas object.
sender.children.add(textblock);
}
</script>
Creating Silverlight Application with Visual Studio
I am assuming that you already have Silverlight application development environment ready in your computer before we start Silverlight application using Visual Studio, if not please visit my another article on An Introduction to Silverlight for development tools.
This section will walk through step by step process of creating very simple Silverlight application using Visual Studio 2008.
Step -1: - From Visual Studio file menu select New Project dialog box --> select Silverlight Application template. You can select VB.NET or C# as programming language for coding and give meaning full name to your solution.
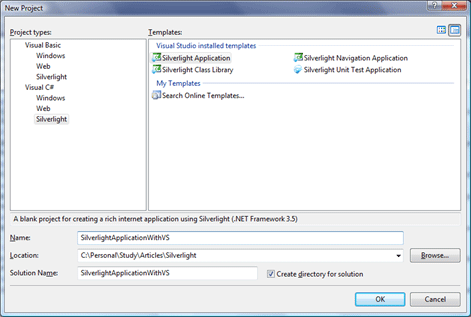
Step -2: - This is optional; you can select the hosting application template while creating Silverlight application itself or if you already have one website for hosting this Silverlight project you can uncheck the checkbox and click ok to continue.
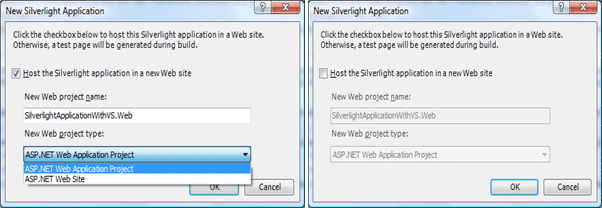
Step -3: - Before we start the actual development it is very important to understand solution explorer for Silverlight and hosting projects. Visual Studio template does lot of thing for developer behind the scene, however it is developer's responsibility to understand what is happening behind the scene. Here we will talk about each and every file created by Visual Studio for us.
Silverlight Application Project files
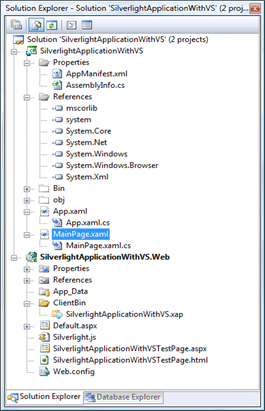
A Silverlight application project contains the following configuration, assembly references, and code files:
AppManifest.xml
This is the application manifest file that is required to generate the application package (.XAP file). You must not edit this file. This file will be updated automatically when we build the Silverlight application. This file identifies the packaged assemblies and the application entry point etc…
<Deployment xmlns="http://schemas.microsoft.com/client/2007/deployment"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<Deployment.Parts>
</Deployment.Parts>
</Deployment>
AssemblyInfo.cs or AssemblyInfo.vb
Nothing new in this file, if you are .NET developer, you will find this file in every .NET application which contains the name and version metadata that is embedded into the generated assembly.
Silverlight project references:
- mscorlib.dll
- System.dll
- System.Core.dll
- System.Net.dll
- System.Windows.dll
- System.Windows.Browser.dll
- System.Xml.dll
App.xaml and App.xaml.cs files
This is almost similar to ASP.NET application's Global.asax file. You can have any name to this file but it should be inherit from Application class. This is the entry point to your Silverlight application.
The App class is required by a Silverlight application to display the application user interface. The App class is implemented by using App.xaml and App.xaml.cs or App.xaml.vb. The App class is instantiated by the Silverlight plug-in after the application package (.xap file) is created.
App.xaml
<Application xmlns=http://schemas.microsoft.com/winfx/2006/xaml/presentation
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
x:Class="SilverlightApplicationWithVS.App">
<Application.Resources>
</Application.Resources>
</Application>
This file provides the following services:
- Application Entry Point
- Application Lifetime
- Application Management
- Application-Scoped Resources
- Unhandled Exception Detection
App.xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace SilverlightApplicationWithVS
{
public partial class App : Application
{
public App()
{
this.Startup += this.Application_Startup;
this.Exit += this.Application_Exit;
this.UnhandledException += this.Application_UnhandledException;
InitializeComponent();
}
private void Application_Startup(object sender, StartupEventArgs e)
{
this.RootVisual = new MainPage();
}
private void Application_Exit(object sender, EventArgs e)
{
}
private void Application_UnhandledException(object sender, ApplicationUnhandledExceptionEventArgs e)
{
// If the app is running outside of the debugger then report the exception using
// the browser's exception mechanism. On IE this will display it a yellow alert
// icon in the status bar and Firefox will display a script error.
if (!System.Diagnostics.Debugger.IsAttached)
{
// NOTE: This will allow the application to continue running after an exception has been thrown
// but not handled.
// For production applications this error handling should be replaced with something that will
// report the error to the website and stop the application.
e.Handled = true;
Deployment.Current.Dispatcher.BeginInvoke(delegate { ReportErrorToDOM(e); });
}
}
private void ReportErrorToDOM(ApplicationUnhandledExceptionEventArgs e)
{
try
{
string errorMsg = e.ExceptionObject.Message + e.ExceptionObject.StackTrace;
errorMsg = errorMsg.Replace('"', '\'').Replace("\r\n", @"\n");
System.Windows.Browser.HtmlPage.Window.Eval("throw new Error(\"Unhandled Error in Silverlight Application " + errorMsg + "\");");
}
catch (Exception)
{
}
}
}
}
MainPage files
This is the main getting started page to your application from which you can navigate to different pages of the application. You will be creating all these User Interface (UI) pages using XAML. This class should inherit from UserControl class.
MainPage.xaml
<UserControl x:Class="SilverlightApplicationWithVS.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x=http://schemas.microsoft.com/winfx/2006/xaml
xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d" d:DesignWidth="640" d:DesignHeight="480">
<Canvas xmlns =http://schemas.microsoft.com/client/2007
Width="640"
Height="480"
Background="Black"
MouseLeftButtonDown="Canvas_MouseLeftButtonDown">
<TextBlock Canvas.Left="4" FontSize="18" Text="My First Silverlight Application" Foreground="#CCCCCC"/>
<Ellipse Canvas.Left="20" Canvas.Top="50"
Fill="Orange" Width="200" Height="80" />
<Rectangle Canvas.Left="250" Canvas.Top="50" Width="80" Height="80" Fill="Red"/>
<Line Canvas.Left="20" Canvas.Top="100"
X1="20" Y1="20" X2="100" Y2="100" Stroke="Yellow" StrokeThickness="10"/>
<Polygon Canvas.Left="80" Canvas.Top="140"
Fill="blue" Stroke="Green" StrokeThickness="10"
Points="20,20 100,100 200,10"/>
<Path Canvas.Left="160" Canvas.Top="140"
Stroke="yellow" StrokeThickness="10">
<Path.Data>
<PathGeometry>
<PathFigure StartPoint="20,20">
<BezierSegment Point1="120,0" Point2="50,300" Point3="200,200"/>
</PathFigure>
</PathGeometry>
</Path.Data>
</Path>
</Canvas>
</UserControl>
You can also have code behind for writing event handler logic with C# or VB.NET.
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace SilverlightApplicationWithVS
{
public partial class MainPage : UserControl
{
public MainPage()
{
InitializeComponent();
}
private void Canvas_MouseLeftButtonDown(object sender, MouseButtonEventArgs args)
{
MessageBox.Show("Hello");
}
}
}
.xap file
Every hosting application should have folder called "ClientBin" to hold the XAP file which is created when we build Silverlight application project. This is also called the Silverlight application package. An application package is a compressed zip file that has a .xap file extension and contains all the files that you need to start your application.
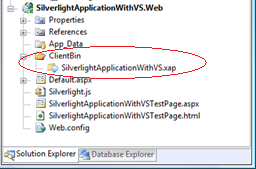
The managed API enables you to bundle managed assemblies and resource files into application package (.XAP) files. The Silverlight plug-in is responsible for loading an application package and extracting its contents.
To verify the compressed zip file, rename a XAP file like SilverlightApplicationWithVS.xap to SilverlightApplicationWithVS.xap.zip. You can then open the archive and view the files inside.
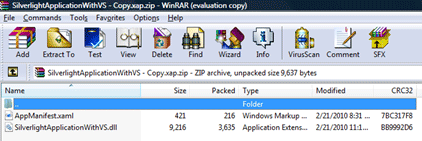
The XAP file system has two obvious benefits:
- It compresses your content: Because this content isn't decompressed until it reaches the client, it reduces the time required to download your application. This is particularly important if your application contains large static resources that can be easily compressed, like XML documents or blocks of text.
- It simplifies deployment: When you're ready to take your Silverlight application live, you simply need to copy the XAP file to the web server, along with SilverlightApplicationWithVSTestPage.html or a similar HTML file that includes a Silverlight content region. You don't need to worry about keeping track of the assemblies and resources.
Silverlight.js
The Silverlight.js file provides JavaScript helper functions for embedding the Silverlight plug-in in a Web page and for customizing the Silverlight installation experience.
You can use the createObject and createObjectEx functions to dynamically embed a Silverlight plug-in in a Web page. These functions generate HTML object elements from the specified parameters.
The Silverlight.js file is installed with the Silverlight SDK in the following location: %ProgramFiles%\Microsoft SDKs\Silverlight\v2.0\Tools.
SilverlightApplicationWithVSTestPage.aspx Test Page
There are two files created to host the application.
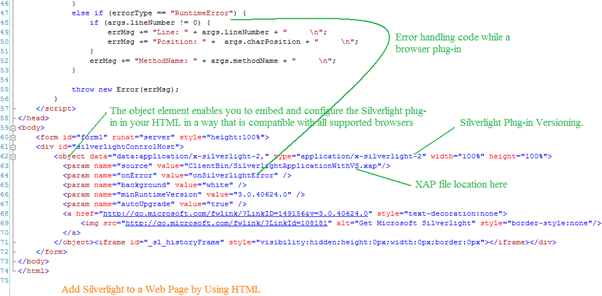
Step -4: - Build the solution. Once you build the solution you will find a xap file in the "ClientBin" folder and it will shows the following output.
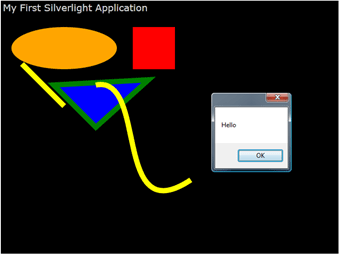
Life cycle of Silverlight Application
Silverlight application life cycle actually start when user request a page which is developed using Silverlight technology. Silverlight Plug-in happens when request come from web user which downloads all the reference files(XAP) including manifest which help for creating instance of application class for rendering Silverlight pages for users.
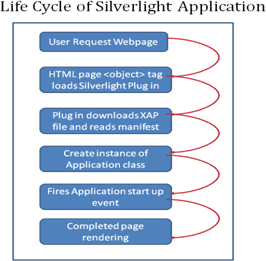
Conclusion
I hope you enjoyed my article; please write your comments on this article which is very important for me.