Introduction
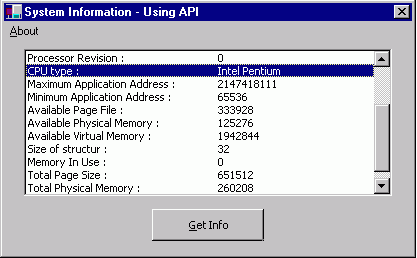
API (Application Programming Interface) is a set of commands, which interfaces the programs with the processors. The most commonly used set of external procedures are those that make up Microsoft Windows itself. The Windows API contains thousands of functions, structures, and constants that you can declare and use in your projects. Those functions are written in the C language, however, so they must be declared before you can use them. The declarations for DLL procedures can become fairly complex. Specifically to C# it is more complex than VB. You can use API viewer tool to get API function declaration but you have to keep in mind the type of parameter which is different in C#.
Most of the advanced languages support API programming. The Microsoft Foundation Class Library (MFC) framework encapsulates a large portion of the Win32 (API). ODBC API Functions are useful for performing fast operations on database. With API your application can request lower-level services to perform on computer's operating system. As API supports thousands of functionality from simple Message Box to Encryption or Remote computing, developers should know how to implement API in their program.
APIs has many types depending on OS, processor and functionality.
OS specific API:
Each operating system has common set of API's and some special e.g. Windows NT supports MS-DOS, Win16, Win32, POSIX (Portable Operating System Interface), OS/2 console API and Windows 95 supports MS-DOS, Win16 and Win32 APIs.
Win16 & Win32 API:
Win16 is an API created for 16-bit processor and relies on 16 bit values. It has platform independent nature e.g. you can tie Win16 programs to MS-DOS feature like TSR programs.
Win32 is an API created for 32-bit processor and relies on 32 bit values. It is portable to any operating system, wide range of processors and platform independent nature.
Win32 APIs has 32 prefix after the library name e.g. KERNEL32, USER32 etc
All APIs are implemented using 3 Libraries.
- KERNEL
It is the library named KERNEL32.DLL, which supports capabilities that are associated with OS such as
Process loading.
Context switching.
File I/O.
Memory management.
e.g. The GlobalMemoryStatus function obtains information about the system's current usage of both physical and virtual memory.
USER
This is the library named "USER32.DLL" in Win32.
This allows managing the entire user interfaces such as
Windows
Menus
Dialog Boxes
Icons etc.,
e.g. The DrawIcon function draws an icon or cursor into the specified device context.
GDI (Graphical Device Interface)
This is the library named "GDI32.dll" in Win32. It is Graphic output library. Using GDI Windows draws windows, menus and dialog boxes.
It can create Graphical Output.
It can also use for storing graphical images.
e.g. The CreateBitmap function creates a bitmap with the specified width, height, and color format (color planes and bits-per-pixel).
C# and API:
Implementing APIs in C# is tuff job for beginners. Before implementing API you should know how to implement structure in C#, type conversion, safe/unsafe code, managed/unmanaged code and lots more.
Before implementing complex APIs we will start with simple MessageBox API. To implement code for MessageBox API open new C# project and add one button. When button gets clicked the code will display Message Box.
Since we are using external library add namespace:
using System.Runtime.InteropServices;
Add following lines to declare API:
[DllImport("User32.dll")]
public static extern int MessageBox(int h, string m, string c, int type);
WhereDllImport attribute used for calling method from unmanaged code. "User32.dll" indicates library name. DllImport attribute specifies the dll location that contains the implementation of an extern method. The static modifier used to declare a static member, which belongs to the type itself rather than to a specific object, extern is used to indicate that the method is implemented externally. A method that is decorated with the DllImport attribute must have the extern modifier. MessageBox is function name, which returns int and takes 4 parameters as shown in declaration.
Many APIs uses structure to pass and retrieve values, as it is less expensive. It also uses constant data type for passing constant data and simple data type for passing Built-in data type as seen in previous declaration of MessageBox function.
Add following code for button click event:
protected void button1_Click (object sender, System.EventArgs e)
{
MessageBox (0,"API Message Box","API Demo",0);
}
Compile and run project, after clicking on button you will see MessageBox, which you called using API function!!!
Using structure
Working with APIs, which uses complex structure or structure in structure, is somewhat complex than using simple API. But once you understand the implementation then whole API world is yours.
In next example we will use GetSystemInfo API which returns information about the current system.
First step is open new C# form and add one button on it. Go to code window of form and add namespace:
using System.Runtime.InteropServices;
Declare structure, which is parameter of GetSystemInfo.
[StructLayout(LayoutKind.Sequential)]
public struct SYSTEM_INFO
{
public uint dwOemId;
public uint dwPageSize;
public uint lpMinimumApplicationAddress;
public uint lpMaximumApplicationAddress;
public uint dwActiveProcessorMask;
public uint dwNumberOfProcessors;
public uint dwProcessorType;
public uint dwAllocationGranularity;
public uint dwProcessorLevel;
public uint dwProcessorRevision;
}
Declare API function:
[DllImport("kernel32")]
static extern void GetSystemInfo(ref SYSTEM_INFO pSI);
Where ref is method parameter keyword causes a method to refer to the same variable that was passed into the method.
Add following code in button click event in which first create struct object and then pass it to function.
protected void button1_Click (object sender, System.EventArgs e)
{
try
{
SYSTEM_INFO pSI = new SYSTEM_INFO();
GetSystemInfo(ref pSI);
//
//
Once you retrieve the structure perform operations on required parameter
e.g.
listBox1.InsertItem (0,pSI.dwActiveProcessorMask.ToString());
//
//
//
}
catch(Exception er)
{
MessageBox.Show (er.Message);
}
}
In attached code I used two API's to retrieve various information from system.
NOTE: I used beta version 1.0 for creating sample application. In Beta2 declaration of API may be slightly different than 1.0.