'Nullable Enable' To Avoid Null Reference Exception
Introduction
We have seen unknown null reference in our production code sometimes. To avoid this this common problem, Microsoft has come up with a new C# 8.0 feature called 'nullable enable'. Enabling this nullable feature in your code will identify all areas where null reference exception can occur and force you to fix it by either adding null check or throwing known exception.
Implementation
Let's see the below code snippet and understand where the null reference exception can happen. if we miss one in development then it can create an issue in production later.
- class Program
- {
- static void Main(string[] args)
- {
- var trainings = TrainingService.GetTrainings();
- foreach (var item in GetTrainingData(trainings))
- {
- Console.WriteLine(item);
- }
- }
- static IEnumerable<string> GetTrainingData(IEnumerable<Training> trainings)
- {
- foreach (var item in trainings)
- {
- yield return $" Traning {item.Name} has {item.Cost} with first course {item.Courses[0]}";
- }
- }
- }
- public class Training
- {
- public string Name { get; set; }
- public string Courses { get; set; }
- public string Cost { get; set; }
- }
- public static class TrainingService
- {
- public static IEnumerable<Training> GetTrainings()
- {
- var trainings = new List<Training>();
- trainings.Add(new Training() { Name = "c# 8.0", Cost = "$20" });
- trainings.Add(new Training() { Name = "JS", Cost = "$40", Courses = "Basic, Data type, loops etc" });
- return trainings;
- }
- }
if you notice in GetTrainingData() to get the first element of Course, this could create an exception here if Course is null. But currently compiler does not detect this hidden null item and informs you to take care.
if you execute this code, you will get the null reference.
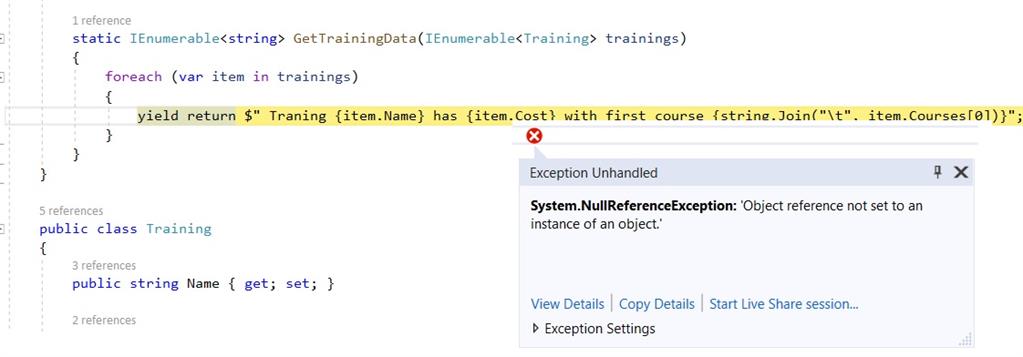
C# 8.0 Nullable enable
C# 8.0 has come with new feature i.e. 'nullable enable', enabling this feature will allow compiler to verify all properties and parameters in your code and track where null reference is possible and inform you to fix it. Let's enable nullable in your code.
- using System;
- using System.Collections.Generic;
- #nullable enable
- namespace TrainingModule
- {
- class Program
- {
- }
- }
Enabling this feature will show you all possible errors (non-nullable property is uninitialized) in your code to fix,
- Initializing all default properties in constructor
- If property is not initialized and is a candidate for null then it will ask to add null check wherever it used, similar to my code snippet where I have placed in GetTraining method.
Final code looks like below after adding nullable feature.
- using System;
- using System.Collections.Generic;
- #nullable enable
- namespace TrainingModule
- {
- class Program
- {
- static void Main(string[] args)
- {
- var trainings = TrainingService.GetTrainings();
- foreach (var item in GetTrainingData(trainings))
- {
- Console.WriteLine(item);
- }
- Console.ReadKey();
- }
- static IEnumerable<string> GetTrainingData(IEnumerable<Training> trainings)
- {
- foreach (var item in trainings)
- {
- yield return item.Courses != null ? $" Traning {item.Name} has {item.Cost} with first course {item.Courses[0]}"
- : $" Traning {item.Name} has {item.Cost}";
- }
- }
- }
- public class Training
- {
- public Training(string name, string cost)
- {
- Name = name;
- Cost = cost;
- }
- public string Name { get; set; }
- public string? Courses { get; set; }
- public string Cost { get; set; }
- }
- public static class TrainingService
- {
- public static IEnumerable<Training> GetTrainings()
- {
- var trainings = new List<Training>();
- trainings.Add(new Training("c# 8.0", "$20"));
- trainings.Add(new Training("JS", "$40") { Courses = "Basic, Data type, loops etc" });
- return trainings;
- }
- }
- }
Conclusion
Here, we learned how to use nullable feature of C# 8.0 in your code and avoid null reference exception during development time.
Author
Ashutosh Gupta
381
3.9k
1.1m