Python Basics
Python
Python is an interpreted, high-level, general-purpose programming language. Created by Guido van Rossum and first released in 1991. he started python as a hobby project to keep him occupied in the week around Christmas. Got its name from the name of British comedy troupe Monty Python. It is used in :
- Software Development
- Web Development
- System Scripting
- Mathematics
Features of Python
- Easy to use
due to simple syntax, it is very easy to implement anything and everything
- Interpreted Language
In this, every code is executed line by line.
- Cross-platform Language
It can run on Windows, Linux, Macintosh, Raspberry Pi, etc.
- Expressive Language
It supports a wide range of library
- Free & Open Source
It can be downloaded free of cost and source code can be modified for improvement
Shortcomings of Python
- Lesser Libraries
As compared to other programming languages like c++, java, .Net, etc. it has a lesser number of libraries, but libraries of other libraries can easily be implemented and imported
- Slow Language
Since being an interpreted language, the code is executed slowly
- Weak on Type-Binding
A variable that is initially declared int can be changed to string at any point in the program without any typecasting.
Installing Python
- Python latest version can be download from https://www.python.org/downloads/.
- At the time of writing this, the latest version was 3.9
- Download the installer file according to your operating system and execute the installer commands according to your Operating System.
Python 2 vs Python 3
Python 2 | Python 3 |
1. The output of the division operator is an integer | 1. The output of the division operator is Float |
2. print "hello" is the implementation of the print function here | 2. print("hello") is the implementation of the print function here |
3. Here Bytes and String is the same | 3. Here Bytes and String is different |
4. Implicit String type here is ASCII | 4. Implicit String type here is Unicode |
5. raise IOError, “your error message” is used to raise an exception | 5. raise IOError(“your error message”) is used for raising an exception |
6. The syntax here is comparatively difficult to understand. | 6. The syntax is simpler and easily understandable. |
7. The xRange() function used to perform iterations. | 7. The new Range() function introduced to perform iterations. |
8. The value of the global variable will change while using it inside for-loop. | 8. The value of variables never changes. |
9. Python 2 code can be converted to Python3 code | 9. It is not backward compatible |
10. It uses raw_input() for taking inputs from the command line | 10. It uses input() for taking inputs from the command line |
Flavors of Python
1. CPython
Written in C, the most common implementation of Python
2. JPython
Written in Java, compiles to bytecode
3. IronPython
Implemented in C#, an extensibility layer to frameworks written in .NET
4. Brython
Browser Python runs in the browser
5. RubyPython
A bridge between Python and Ruby interpreters
6. PyPy
Implementation in Python
7. MicroPython
Runs on a Microcontroller
Python Character Set
The following are then character set recognized by python. It uses the traditional ASCII character set.
- Letters
A-Z, a-z
- Digits
0-9
- Special Symbols
Special Symbols available over Keyboard (some of the symbols like rupee symbol may not be available)
- White Spaces
blank space, tab, carriage return, newline, form feed
- Other Characters
Unicode
Python Tokens
The smallest individual unit in a program is known as a token.
- Keywords
There are in total of 31 reserved keywords. Ex: and, finally, not, etc.
- Identifiers
a python identifier is a name used to identify a variable, function, class, module, or other objects. Ex: MyBook, myBook, mybook, etc.
- Literals
Literals in Python can be defined as numbers, text, or other data that represent values to be stored in variables. Ex: age = 22, escape sequence, etc.
- Operators
It can be defined as symbols that are used to perform operations on operands. Ex: arithmetic operators, etc.
- Punctuators
Used to implement the grammatical and structure of a Syntax. Ex: &=, >>=, <<=, etc.
Python Keywords
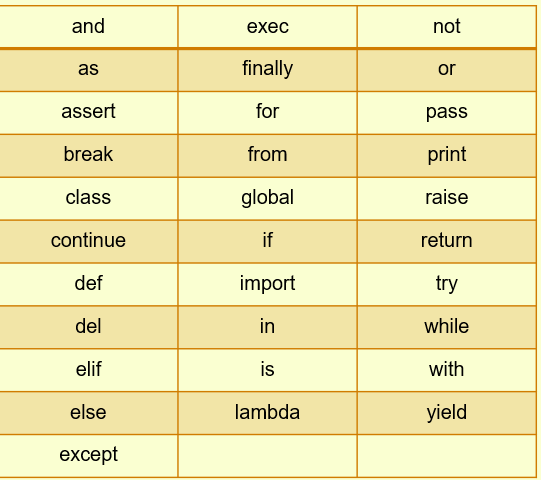
Rules to define Python Identifiers
- An identifier starts with a letter A to Z or a to z or an underscore (_) followed by zero or more letters, underscores, and digits (0 to 9).
- Python does not allow special characters
- An identifier must not be a keyword of Python.
- Python is a case sensitive programming language.
- Class names start with an uppercase letter. All other identifiers start with a lowercase letter.
- Starting an identifier with a single leading underscore indicates that the identifier is private.
- Starting an identifier with two leading underscores indicates a strongly private identifier.
- If the identifier also ends with two trailing underscores, the identifier is a language-defined special name.
Python Punctuators
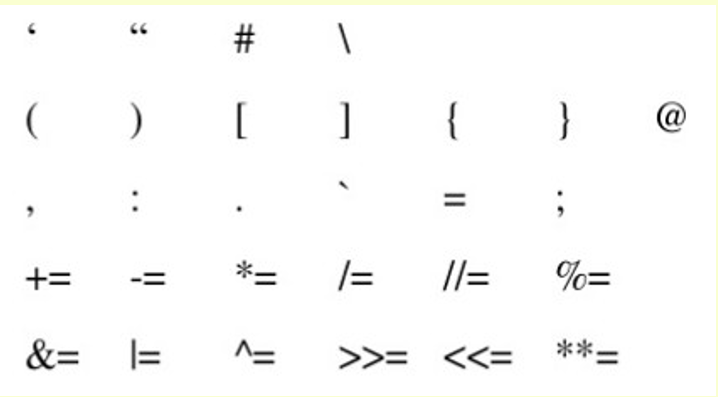
Barebone of a Python Program
- Expression
- Statements
- Comments
- Function
- Block & Indentation
Python Variables
A variable can be considered as a container that holds value. Python is a type-infer language i.e. one which can detect data types automatically or in other words it means you dont need to specify the data type of variable.
Note: although python supports unlimited variable length, following PEP-8, we keep the maximum length to 79 characters
For example:
- name = 'python' # String Data Type
- sum =None # a variable without a value
- a = 23 # integer
- b = 6.2 # Float
- sum = a+b
- print(sum)
Multiple Assignments
- a =b =c =1 #single value to multiple variables
- a,b = 1, 2 #multiple value to multiple variable
- a,b = b,a #value of a and b is swapped
Variable Scope and Lifetime
- Local Variable
- def fun():
- x=8
- print(x)
-
- fun()
- print(x) #error will be shown
- Global Variable
- x = 8
- def fun():
- print(x) #Calling variable 'x' inside fun()
-
- fun()
- print(x) #Calling variable 'x' outside fun()
Python Data Types
- Number
It is used to store numeric values. Python has 3 numeric types:Ex: a = 10Ex: a = 10.0Ex: a = complex(10,5) # 10+5j
- String
A string is a sequence of characters. In python, we can create a string using a single ( ' ' ) or double quotes (" "). Both are same in python
- str = 'computer science'
- print('str- ', str) # print string
- print('str[0]-', str[0]) # print 1st char
- print('str[1:3]-', str[1:3]) # print string from position 1 to 3
- print('str[3:]-', str[3:]) # print string starting from 3rd char
- print('str*2-' , str*2) # print string two times
- print('str + yes-', str+'yes') # concatenated string
- Boolean
It is used to store two possible values either true or false
- str = "comp sc"
- b = str.isupper()
- print(b)
- List
List are collections of items and each item has its index value. Denoted by []
- list = [6,9]
- list[0] = 55
- print(list[0])
- print(list[1])
- Tuple
A tuple is an immutable Python Object. Immutable python objects mean you cannot modify the contents of a tuple once it is assigned. Denoted by ()
- tup = (66,99)
- Tup[0] = 3 #error will be displayed
- print(tup[0])
- print(tup[1])
- Set
It is an unordered collection of unique and immutable items. Denoted by {}
- set1 = {11, 22, 33, 22}
- print(set1)
- Dictionary
It is an unordered collection of items and each item consists of a key and a value.
- dict = {'subject' : 'comp sc", 'class' : '11'}
- print(dict)
- print( "Subject :", dict["subject"])
- print("class :", dict.get('class'))
Python Operators
The Precedence of Operators in Python can be found from the rule PEMDAS ( Parenthesis, Exponential, Multiply, Division, Addition, Subtraction). Operators with the same precedence are evaluated from left to right.
- Python Arithmetic Operator
- x = 5
- y = 4
- print('x + y =',x+y) #Addition
- print('x - y =',x-y) #Substraction
- print('x * y =',x*y) #Multiplication
- print('x / y =',x/y) #Division
- print('x // y =',x//y) #Floor Division
- print('x ** y =',x**y) #Exponent
- Python Comparison Operators
- x = 101
- y = 121
- print('x > y is',x>y) #Greater than
- print('x < y is',x<y) #Less than
- print('x == y is',x==y) #Equal to
- print('x != y is',x!=y) #Not Equal to
- print('x >= y is',x>=y) #Greater than equal to
- print('x <= y is',x<=y) #Less than equal to
- Python Logical Operators
- x = True
- y = False
- print('x and y is',x and y) #if both are true
- print('x or y is',x or y) #if either one is true
- print('not x is',not x) #returns the complement
- Python Bitwise Operators
- a = 6
- b = 3
- print ('a=',a,':',bin(a),'b=',b,':',bin(b)) c = 0
- c = a & b
- print ("result of AND is ", c,':',bin(c))
- c = a | b
- print ("result of OR is ", c,':',bin(c))
- c = a ^ b
- print ("result of EXOR is ", c,':',bin(c))
- c = ~a
- print ("result of COMPLEMENT is ", c,':',bin(c))
- c = a << 2
- print ("result of LEFT SHIFT is ", c,':',bin(c))
- c = a>>2
- print ("result of RIGHT SHIFT is ", c,':',bin(c))
- Python Membership Operators
- a = 5
- b = 10
- list = [1, 2, 3, 4, 5 ]
- if ( a in list ):
- print ("Line 1 - a is available in the given list")
- else:
- print ("Line 1 - a is not available in the given list")
- if ( b not in list ):
- print ("Line 2 - b is not available in the given list")
- else:
- print ("Line 2 - b is available in the given list")
- Python Identity Operators
- a = 10
- b = 10
- print ('Line 1','a=',a,':',id(a), 'b=',b,':',id(b))
- if ( a is b ):
- print ("Line 2 - a and b have same identity")
- else:
- print ("Line 2 - a and b do not have same identity")
Python Operator Overloading
Unary Operator | Method |
- | __neg__(self) |
+ | __pos__(self) |
Python Control Statements
Control statements are used to control the flow of execution depending upon the specified condition/logic.
- If- Statement
- noofbooks = 2
- if (noofbooks == 2) :
- print(" You have")
- print("two books")
- print("outside of if statement")
- if-else statement
- a=10
- if a<100:
- print('less than 100')
- else:
- print('more than equal 100')
- Nested If-Else Statement
- num = float(input("enter a number:"))
- if num >=0:
- if num==0:
- print("zero")
- else:
- print("Negative number")
Python Control Loops
- While Loop
- x=1
- while x<=4 :
- print(x)
- x = x+1
- For Loop
- for i in range (3,5):
- print(i)
In the above code, we are printing numbers from 3 to 5.
- Nested For Loop
- for i in range (1,3):
- for j in range(1,11):
- k=i*j
- print(k, end = ' ')
- print()
In the above code, we are running our loop firstly from 1 to 3 and then for each iteration running for another 10 times.
Python Jump Statements
They are used to transfer the program's control from one location to another. This means these are used to alter the flow of a loop like-to skip a part of a loop or terminate a loop.
- Break
- for val in "string":
- if val == "i":
- break
- print(val)
In the above code, we break the loop as soon as "i" is encountered, hence printing "str"
- continue
- for val in "init":
- if val == "i":
- continue
- print(val)
- print(" The End")
In the above code, we will be printing "nt", because for "i" it will skip.
-
pass
- for i in "initial":
- if(i == "i"):
- pass
- else:
- print(i)
Continue forces the loop to start the next iteration while pass means "there is no code to execute here" and will continue through the remainder of the loop body.
The output will be "ntal"
Python Errors & Exception
Errors
A Syntax error is an error in the syntax of a sequence of characters or tokens that are intended to be written in a particular programming language.
For Example:
>>> while True print 'Hello world'
In the above code, it is an Invalid Syntax error. It should be as :
>>> while True:
print 'Hello world'
Standard Errors available in Python are:
IndentationError, SystemExit, ValueError, TypeError, RuntimeError
Exceptions
The other kind of error in Python are exceptions. Even if a statement or expression is syntactically correct, it may cause an error when an attempt is made to execute it. Errors detected during execution are called exceptions
For Example:
>> 10 * (1/10)
The above code is syntactically okay, but when we execute it, it will result in ZeroDivisionError: integer division or modulo by zero.
Standard Exceptions available in Python are:
SystenExit, OverflowError, FloatingPointError, ZeroDivisonError, EOFError, KeyboardInterrupt, IndexError, IOError
Handling an Exception
If we see some suspicious code that may raise an exception, we can defend our program by placing the code in the try block
Syntax
try:
you do your operation here
except Exception1:
if there is Exception1, then execute this block
except Exception2:
if there is Exception2, then execute this block
.........
else:
if there is no exception, then execute this block.
- try:
- fh = open("testfile", "r")
- fh.write("This is my test file for exception handling!!")
- except IOError:
- print ("Error: can\'t find file or read data")
- else:
- print ("Written content in the file successfully")
Python List
Function | Description |
list.append() | Add an item at end of a list |
list.extend() | Add multiple items at the end of a list |
list.insert() | insert an item at a defined index |
list.remove() | remove an item from a lsit |
del list[index] | delete an item from a list |
list.clear() | empty all the list |
list.pop() | remove an item at a defined index |
list.index() | return index of the first matched item |
list.sort() | sort the items of a list in ascending or descending order |
list.reverse() | reverse the items of a list |
len(list) | return total length of the list |
max(list) | return item with maximum value in the list |
min(list) | return item with the minimum value in the list |
list(seq) | converts a tuple, string, set, dictionary into a list |
Python Dictionary
Function | Description |
dict.clear() | removes all elements of dictionary dict |
dict.copy() | returns a shallow copy of dictionary dict |
dict.items() | retuns a list of dict's (key, value) tuple pairs |
dict.setdeafult(key, default=Nonre) | similiar to get(), but will set dict[key] = default |
dict.update(dict2) | adds dictionary dict2's key-values pairs to dict |
dict.keys() | returns list of dictionary dict's keys |
dict.values() | returns list of dictionary dict's values |
Python Tuples
Function | Description |
tuple(seq) | converts a list into a tuple |
min(tuple) | returns item from the tuple with a minimum value |
max(tuple) | returns item from the tuple with maximum value |
len(tuple) | gives the total length of the tuple |
cmp(tuple1,tuple2) | compares elements of both tuples |
Python Class
A class is a code template for creating objects. Objects have member variables and have behavior associated with them. In python, a class is created by the keyword
class
.
An object is created using the constructor of the class. This object will then be called the instance
of a class. In Python, we create instances in the following manner.Declaring a Class:
class name:
statements
Using a Class
- class Point:
- x = 0
- y = 0
- # main
- p1 = Point()
- p1.x = 2
- p1.y = -5
- from Point import *
- # main
- p1 = Point()
- p1.x = 7
- p1.y = -3
- # Python objects are dynamic (can add fields any time!)
- p1.name = "Tyler Durden"
Objects Methods
def name (self, parameter, ....., parameter):
statements
In the above code, self is very important, as, without the presence of self, python will not allow us to use any of the class member functions. It is similar to "this" used in Java. With only one difference that in java using "this" is not compulsory
- from math import *
- class Point:
- x = 0
- y = 0
- def set_location(self, x, y):
- self.x = x
- self.y = y
- def distance_from_origin(self):
- return sqrt(self.x * self.x + self.y * self.y)
- def distance(self, other):
- dx = self.x - other.x
- dy = self.y - other.y
- return sqrt(dx * dx + dy * dy)
In the above code, we have created 3 methods namely set_location(), distance_from_origin() and distance().
Calling Methods
A client can call the methods of an object in two ways:
- object.method( parameter)
- Class.method( object, parameters)
- p = Point(3, -4)
- p.translate (1, 5)
- Point.translate (p, 1, 5)
Python Class Constructors
a constructor is a special method with the name
__init__
def __init__ (self, parameter, ....., parameter):
statements
- class Point:
- def __init__( self, x, y):
- self.x = y
- self.y = y
toString and __str__
It is equivalent to Java's
toString
which converts objects to a string. It is automatically involved when str
or print
is called.def __str__( self):
return string
- from math import *
- class Point:
- def __init__(self, x, y):
- self.x = x
- self.y = y
- def distance_from_origin(self):
- return sqrt(self.x * self.x + self.y * self.y)
- def distance(self, other):
- dx = self.x - other.x
- dy = self.y - other.y
- return sqrt(dx * dx + dy * dy)
- def translate(self, dx, dy):
- self.x += dx
- self.y += dy
- def __str__(self):
- return "(" + str(self.x) + ", " + str(self.y) + ")"
Python Operator Overloading in Python Classes
Operator | Class Methods |
- | __sub__(self, other) |
+ | __pos__(self, other) |
* | __mul__(self, other) |
/ | __truediv__(self, other) |
== | __eq__(self, other) |
!= | __ne__(self, other) |
< | __lt__(self, other) |
> | __gt__(self, other) |
<= | __le__(self, other) |
>= | __ge__(self, other) |
Summary
In the next chapter, we will learn some basics about Python Django.
Author
Rohit Gupta
45
31.8k
3.1m