Operations on Database models
Introduction
In this chapter, we will learn various operations that can be performed on database models.
Store data in Database:
- Now we will see some extra features of models in Django that will help for customization of the database.
- So, I have already made a demo of a Django project that has the main Django app.
- Open models.py file in the Django app folder.
- Now we want to first create a database using migration.
- Open Command Prompt with the project root directory.
- Enter Commands
- python manage.py makemigrations <Enter Django app name>
- python manage.py sqlmigrate <Enter django app name>
- <prefix of the file which generates from the above command like 0001>
- python manage.py migrate
- So you have to create a database table successfully then we want to save data through command prompt in the database.
- Now Enter command in CMD: python mange.py shell. The above command is used for opening a shell of a demo project that gives direct access to project class and models that are shown as below image.
- Now I have a class named UserData that is shown in code that also defines the models or Table name.
- Now we store the data in the database using some code as below.
- from main.models import UserData
- user= UserData(emailid="[email protected]",password="12345678")
- user.save()
Here main.models denote the file in the main folder that has UserData models. So we access those models using the above code. The second code stored data in is user object. save() is a predefined function that saves object value into the database.
the user object is given many features to see data of objects which were saved using it.
- user.emailid
- user.password
- user.pk
- user. _get_pk_val()
The output of the above code is as shown in the below image:
Access data from Database
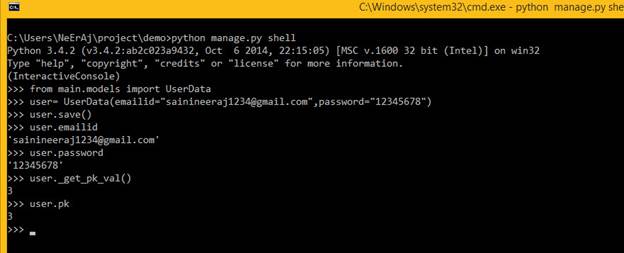
- You have saved data into the database. You can check it some code as below:
- Now you have access to information about UserData Tables.
- So you can access database value with many constraints using predefined methods of Django.
- UserData.objects.filter(pk=2).values_list()
- UserData.objects.filter(emailid='[email protected]').values_list()
- UserData.objects.filter(pk=2).values_list().values()
- UserData.objects.filter(pk=2).values_list().exists()
- UserData.objects.filter(pk=2).values_list()
- It is very useful for that person who doesn’t know SQL queries for doing operations on Database.
- user = UserData.objects.get(pk=2)
- user.password = 'abcdefgh'
- user.save()
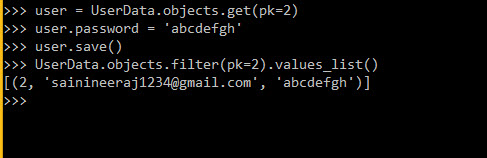
Delete data in Database
Now we will do the operation of deleting data from the database.
- Firstly delete data using filter techniques that remove specific data from the database.
- UserData.objects.values_list()
- UserData.objects.filter(pk=3).delete()
- UserData.objects.values_list()
- UserData.objects.values_list()
- Delete all values from the database table.
- UserData.objects.all().delete()
- UserData.objects.all().delete()
Django has many methods for using databases directly without knowledge of using SQL queries. I hope you understood this part of the python Django tutorial.
Summary
In the next chapter, we will discuss view and URL handling.
Author
Neeraj Kumar
0
8.1k
2.4m