Introduction
This article will explain you about how to work with command line argument .i.e. passing Parameters to Main method of the Console Application in C# and do processing with it.
Technologies:
.net 2.0/3.5
Prerequisites:
Basic knowledge of accessing array in c#.
Implementation
Basically main method can have two Signatures.
static void Main(string[] args)
or
static int Main(string[] args)
In which string[] args will store whatever we have passed through command line to our application. 0th element in args array will hold Application Name it Self.
Suppose our application's name is printname.exe that prints the names on screen when passed by command line like below command in cmd.exe
printname.exe kirtan suresh chirag
it will print
printname
kirtan
suresh
chirag
Note: Here First Name printed is application name as I told you before that String[] Args's 0th element will hold Application Name into it Automatically.
So, now
My purpose of writing this article is to teach you how to make such an application that take this type of input as i shown above.
So main thing here is how to get those arguments that are passed through command line?
Well simply You can Process all the Elements Passed into command Line by Simply Process Array string[] args.
Like below code using foreach loop if your desire code is into Main method it self.
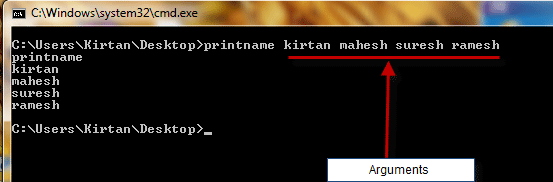
static int Main(string[] args)
{
foreach (string arg in args)
{
Console.WriteLine(arg);
}
Console.ReadKey();
return 0;
}
Above was simple one now main question is:
How to get command line arguments in other Functions than Main() ?
You can simply pass it to other function but it will be cumbersome as you have to work like pipeline work to pass it on to other functions but here is solution to it
You can access Command Line Arguments globally Using Environment Class's GetCommandLineArguments() method which returns string[] array of all CommandLine Arguments.
so you can use command line arguments passed to Main function any were in Program without passing it any were. See the sample code for it.
class Program
{
static int Main(string[] args)
{
Print();
Console.ReadKey();
return 0;
}
static void Print()
{
string[] args = Environment.GetCommandLineArgs();
foreach (string arg in args)
{
Console.WriteLine(arg);
}
}
}
Here print function using command line arguments .
That's it .
You have successfully learned how to use command line arguments.
Conclusion
This article explains about how to work with Command line Arguments in C# Console Application.