Introduction
When developing applications that have menus, toolbars, and other controls, the task of enabling the correct controls as the application changes state can be difficult. In this article, I will present a design pattern that will simplify coding and make it possible to verify the correctness of the enabling logic by inspecting the code.
The pattern I'm presenting works by putting all the enabling logic in one place and coding it in a way that makes the intent clear. This means you shouldn't have to test and fix until it appears to work.
An example
The example is meant to be small, but have complex enough enabling conditions to make it interesting. It's a simple list editor that saves the list contents in an XML file.
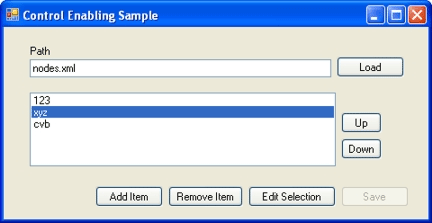
The enabling rules
Here are the enabling rules for the example:
- The Load button is only enabled when there is some text in the path text box. Also, we don't want to load a new file without saving changes to the old one, so the Load button isn't enabled when there are changes that have not been saved.
- For demonstration, no more than five items can be in the list, so the Add button is enabled only when there are less than five items in the list.
- The Remove Item button is only enabled when an item is selected.
- The Edit Selection button is only enabled when an item is selected.
- The Save button is only enabled if a change has been made since the last save and there is some text in the path text box.
- The Up button is enabled when an item in the list box is selected that's not the top item.
- The Down button is enabled when an item in the list box is selected that's not the bottom item.
Steps
- Create an EnableControls method.
- Define and set Boolean variables that will become the primitives in our control enabling language.
- For each menu item, toolbar item, or other control, combine the Boolean "primitives", using ands (&&) and ors (||) to define the conditions under which each control is enabled.
- Call the EnableControls method from the event handler for each event that has the potential to change the application state.
private void EnableControls()
{
// define primitives
bool havePath = textBoxPath.Text != "";
bool isSelection = listBoxItems.SelectedIndex != -1;
bool canAdd = listBoxItems.Items.Count < _maxItemCount;
bool canMoveUp = listBoxItems.SelectedIndex > 0;
bool canMoveDown =
listBoxItems.SelectedIndex < listBoxItems.Items.Count - 1;
// use primitives to enable controls
buttonAddItem.Enabled = canAdd;
buttonRemoveItem.Enabled = isSelection;
buttonLoad.Enabled = havePath && !_dirty;
buttonEditSelection.Enabled = isSelection;
buttonSave.Enabled = _documentIsLoaded && _dirty && havePath;
buttonUp.Enabled = isSelection && canMoveUp;
buttonDown.Enabled = isSelection && canMoveDown;
}
// call EnableControls from event handlers
private void listBoxNodes_SelectedIndexChanged(object sender,EventArgs)
{
EnableControls();
}
As you read this code, the conditions that allow each control to be enabled are clear. If you're like me, you will still make mistakes. But the mistakes we be much easier to find and repair, and less likely to cause other errors.
Conclusion
Imagine what the code would look like if we had put enabling logic into each event, as is commonly done. And this is a simple example. Real-life applications can be much more complex, and the need to manage complexity through good programming patterns is even greater.