Basically this article gives an idea about how to pass the parameters and getting output parameters from the workflow.
Firstly let me discuss about the work flow basics. There are two important classes to understand for executing any workflow.
Workflow runtime: This class represents workflow runtime execution environment. We need to create an instance of work flow runtime engine to execute workflows. To execute any workflow first , we need to instantialte workflow runtime as below.
private WorkflowRuntime wfRunTime = new WorkflowRuntime();
Workflow instance: Represents a workflow instance by using the specified workflow
WorkflowInstance wi;
Once we have runtime environment for executing the workflow, we need to create new workflow instance as below. There are five overloaded methods to create workflow instance from workflow runtime class .one of the method, it takes Dictionary collection which defines the passing parameters to the work flow and getting output parameters values from the workflow.
In this example, 'params' is declared as dictionary collection and passed to the create workflow instance method.
var parms = new Dictionary<string, object>();
wi = wfRunTime.CreateWorkflow(typeof(WorkflowEx), parms);
To retrieve the output result from workflow, workflowcompleted event of workflow runtime will be handled.
wfRunTime.WorkflowCompleted += new EventHandler<WorkflowCompletedEventArgs>(wfRunTime_WorkflowCompleted);
The WorkflowCompletedEventArgs property of output parameters. We can retrieve the output parameters from the completed workflow.
Dictionary<string, object> OutputParameters { get; }
Workflow is added to solution.
In this example, sequential workflow class is added to run this example and named as workflowEx.
If else activity is added to the workflow and based on the user choice, the code will executed.
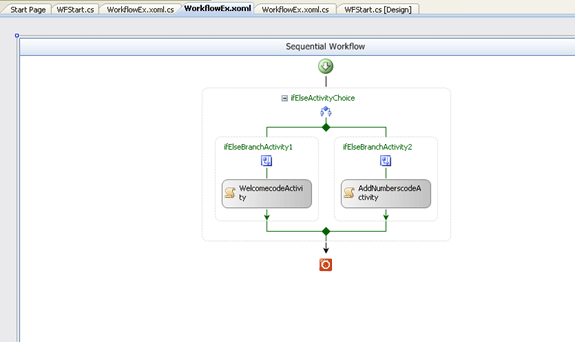
There are two code activities
- output welcome message from the workflow
- Giving sum and difference of the two numbers
All the properties i.e input parameters and output parameters will be declared in the workflow class
public partial class WorkflowEx : SequentialWorkflowActivity
{
private int _num1;
private int _num2;
private int _sum;
private int _diff;
private string _yourName;
private string _outputMessage;
private bool _choice =false;
public bool Choice
{
set { _choice =value; }
get {
return _choice;
}
}
public string OutputMessage
{
get { return _outputMessage; }
set
{
_outputMessage = value;
}
}
public string YourName
{
set { _yourName = value; }
get
{
return _yourName;
}
}
public int Num1
{
set { _num1 = value; }
}
public int Num2
{
set { _num2 = value; }
}
public int Sum
{
get { return _sum; }
}
public int Diff
{
get { return _diff; }
}
private void AddNumberscodeActivity_ExecuteCode(object sender, EventArgs e)
{
_sum = _num1 + _num2;
_diff = _num1 - _num2;
}
private void EvaluateCondition(object sender, ConditionalEventArgs e)
{
if (this.Choice)
{
e.Result = true;
}
else
{
e.Result = false;
}
}
private void WelcomecodeActivity_ExecuteCode(object sender, EventArgs e)
{
OutputMessage = "hey " + YourName + " i am from work flow ";
}
}
In this example, based on user choice, the work flow will be executed and returns the output. Once we create workflow, we need the instance of workflow instance to start the execution of the workflow instance and start the work flows instance to run.
As it was explained, create workflow takes the dictionary type of collection as parameter. Here, we are getting the values from windows form and passing the values to workflow.
Two choices are present on the windows form. Based on user choice, the workflow should return the welcome message or number addition.
choice = rdoString.Checked;
var parms = new Dictionary<string, object>();
parms.Add("Choice", choice);
wi = wfRunTime.CreateWorkflow(typeof(WorkflowEx), parms);
wi.Start();
If the user selects as a choice of welcome message , then pass the name to the workflow as below.
parms.Add("YourName", txtName.Text);
If the user selects as a choice of Number addition , then pass the input numbers to the workflow as below.
parms.Add("Num1", Int32.Parse(txtFstNum.Text));
parms.Add("Num2", Int32.Parse(txtScdNum.Text));
To capture the outparamters from the workflow, workflow completed event will be handled
wfRunTime.WorkflowCompleted += new EventHandler<WorkflowCompletedEventArgs>(wfRunTime_WorkflowCompleted);
Workflowcompleted event args is having propert of out paramters which contains the values of output from workflow.
Getting the welcome message from the workflow:
string opMsge = (string)e.OutputParameters["OutputMessage"];
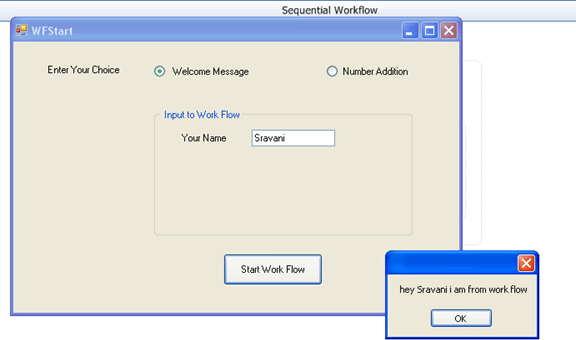
Getting the sum and differnce result from the workflow:
sum = Convert.ToInt32(e.OutputParameters["Sum"]);
diff = Convert.ToInt32(e.OutputParameters["Diff"]);
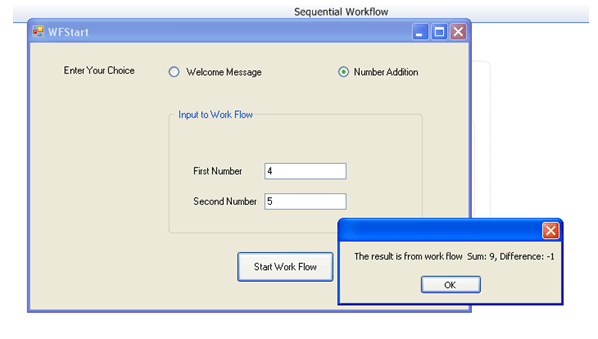