As a first contact with Silverlight applications, the problem is posed, how does an XAML entity interact with code behind I mean C# code, and then many people in forums ask this question, so I decided to write a tutorial about the issue. In this article, I will expose the problematic and some definitions, then in the subsequent parts I will demonstrate some techniques and real use cases through examples and I will illustrate how does this is leveraged.
Problems and questions those need answers:
The first question:
What is mechanism that provides the interconnection and the interchange between a given built in XAML entity or interface and the code behind, I mean C# code?
It is basic and legitimate question, and the answer for that question is the Data Binding. In fact, Data Binding enables XAML entities to interact with the code behind via what could be called as Binding engine. In fact it ties the dependency property of a given XAML entity to the source object property. Thus, every binding has to specify a source and a target. The Binding engine plays the role of the translator in this case because it holds the ability to convert values and provides the inter transfer between the two sides and then make ensure the inter connection between the XAML entity and the source object. The data could be transferred from/to XAML entity from/to source object according to four principal modes:
-
One way:
The data are transmitted in a unique direction from source object to the XAML entity
-
One time:
The data are transmitted in a unique direction from source object to the XAML entity but for only and only once
-
One way to source:
It is like the first case but the data is transmitted in the inverse direction
-
Two ways:
In this case, the data is transmitted in the two directions, from the XAML entity to the source object and from the source object to the XAML entity
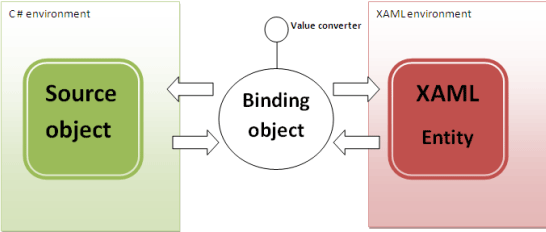
Figure1
The figure 2 gives an idea about the different binding directions
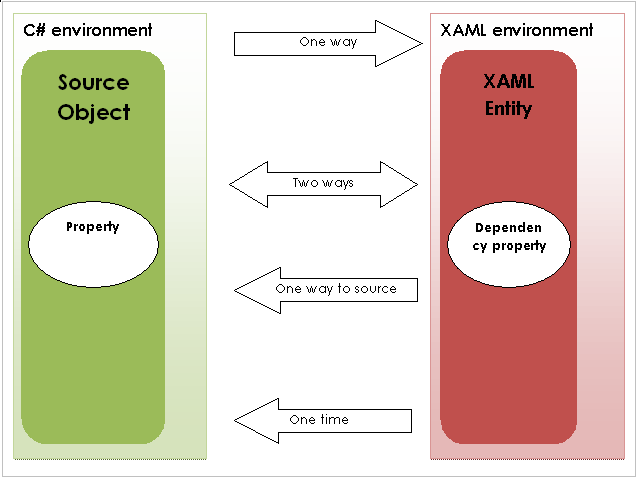
Figure 2
The second question:
What if a property is changed during the application process, how either the UI XAML control or the object source does know that changes are happen and then updating tasks are done? In fact, the source object has to implement an interface that called
INotifyPropertyChanged.
public interface INotifyPropertyChanged
{
event PropertyChangedEventHandler PropertyChanged;
}
This interface has an event as a member, once it is implemented within a given object source, it will be raised exactly when a given property is changed and then updates are applied to a targeted control exactly at the targeted property level.
The third question:
You tell me ok, perfect but what if I have a set of listed objects within a given collection or list and I want that this given collection be notified for each time an element is added or removed, how does one this? In fact an as a response to this question there are two alternatives.
The first one is to make use of the generic ObservableCollection<T> class that implements the INotifyCollectionChanged interface and this is the easy way for instance because it saves us from dealing with the INotifyCollectionChanged interface directly. The second alternative consists on creating our customized collection notified object. This last one has to implement the INotifyCollectionChanged interface. It is recommended to follow the first way because it is standard and very easy to implement, the second way is needed only when special requests or details are needed at the time of adding and removing objects to/from the collection. And this is a representation of the INotifyCollectionChanged.
public interface INotifyCollectionChanged
{
event PropertyChangedEventHandler CollectionChanged;
}
In fact, the CollectionChanged event will be raised each time a modification happens at the object list levels. Recall that INotifyPropertyChanged and INotifyCollectionChanged could be complementary one each other since the first one notifies about changes within a given object, meanwhile the second notifies about changes within a list type of that given object.
The fourth question:
As we said before, the interaction between the two sides could be in the both directions. In the other hand, data could be represented in different formats, date, time, string, integers, Uri and even custom objects that we leverage as a part of a given project. Then the problem appears. Say that you expose a form to the final user through a HTML or an ASP page. And then this final user enters invalid data, like first name that contains numbers, invalid email formats or worse an evil thing such as an SQL injection to get back information from your data base system. Who to integrate validation logic within the interaction process between the source object and the XAML side?
In fact, two properties should be set to true in this case and they are, namely the NotifyOnValidationError property and ValidatesOnExceptions property. The first property, once is set to true, it tells the binding engine to create a validation error when an exception occurs, meanwhile, the second property will tells the binding engine to raise the BindingValidationError event. This even occurs exactly when a data validation error is reported from the source object
The fifth question:
One question remains, what if the developer needs to use a different type of data other than the original one. I explain, say that you have a class within a property type of DateTime, then how to present it within a calendar control at the XAML side. A second case, what if you have a given Uri that you want to represent within a given XAML, then it will be represented as string and then the conversion problem appears since URI and string are different. In other words and as we all know, the displayed data must be type of string, but the original object member is type of URI which differs to string. In that case, what we should do? As a response to this problem an interface could be used to avoid the problem, in fact, it is the IValueConverter interface.
public interface IValueConverter
{
object Convert(object value,
Type targetType,
object parameter,
CultureInfo culture);
object ConvertBack(object value,
Type targetType,
object parameter,
CultureInfo culture);
}
This interface once implemented it provides properties conversion and retro conversion from type to another type with the most flexible way. In the subsequent articles parts, I will answer each of those questions.
Those five questions will be answered in more details within the subsequent parts of this article about the Data Binding logic. So you shouldn't miss them
Good .Netting!!!