Hi All
My query is to get Value from user in textbox, then process it and send HEX Values via Serial Port.
for examle value in textbox is 12345A6789BC01234 and i want to send hex of each value through serial port.
for this i made a dummy program, In this program, i use fixed data instead of getting it from textbox.
fix data is in byte[] dataToSend variable and this program is working fine, sending hex format through serial port.
following is the program and output of program
- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Data;
- using System.Drawing;
- using System.Linq;
- using System.Text;
- using System.Threading.Tasks;
- using System.Windows.Forms;
- using System.IO.Ports;
- using System.IO;
-
- namespace commport_2
- {
- public partial class Form1 : Form
- {
- string dataOUTBuffer;
- string[] dataPrepareToSend;
- int textLength = 0;
- byte[] dataToSend;
-
- byte[] bytetosend = { 0x01, 0x10, 0x80, 0x00, 0x00, 0x0A, 0x14, 0x31, 0x32, 0x33, 0x34, 0x35, 0x41, 0x36, 0x37, 0x38, 0x39, 0x42, 0x43, 0x30, 0x31, 0x32, 0x33, 0x34, 0x00, 0x00, 0x00, 0xC9, 0x21 };
-
- public Form1()
- {
- InitializeComponent();
- }
-
- private void Form1_Load(object sender, EventArgs e)
- {
- string[] ports = SerialPort.GetPortNames();
- cBoxComPort.Items.AddRange(ports);
- }
-
- private void btnOpen_Click(object sender, EventArgs e)
- {
- try
- {
- serialPort1.PortName = cBoxComPort.Text;
- serialPort1.BaudRate = Convert.ToInt32(cBoxBaudRate.Text);
- serialPort1.DataBits = Convert.ToInt32(cBoxDataBits.Text);
- serialPort1.StopBits = (StopBits)Enum.Parse(typeof(StopBits), cBoxStopBits.Text);
- serialPort1.Parity = (Parity)Enum.Parse(typeof(Parity), cBoxParityBits.Text);
-
- serialPort1.Open();
- progressBar1.Value = 100;
-
- }
- catch (Exception err)
- {
- MessageBox.Show(err.Message, "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
- }
-
- }
-
- private void btnClose_Click(object sender, EventArgs e)
- {
- if(serialPort1.IsOpen)
- {
- serialPort1.Close();
- progressBar1.Value = 0;
- }
- }
-
- private void btnSendData_Click(object sender, EventArgs e)
- {
- if(serialPort1.IsOpen)
- {
- serialPort1.Write(bytetosend, 0, bytetosend.Length);
- }
- }
-
- private void button1_Click(object sender, EventArgs e)
- {
- tBoxDataOUT.Text = "";
- }
- }
- }
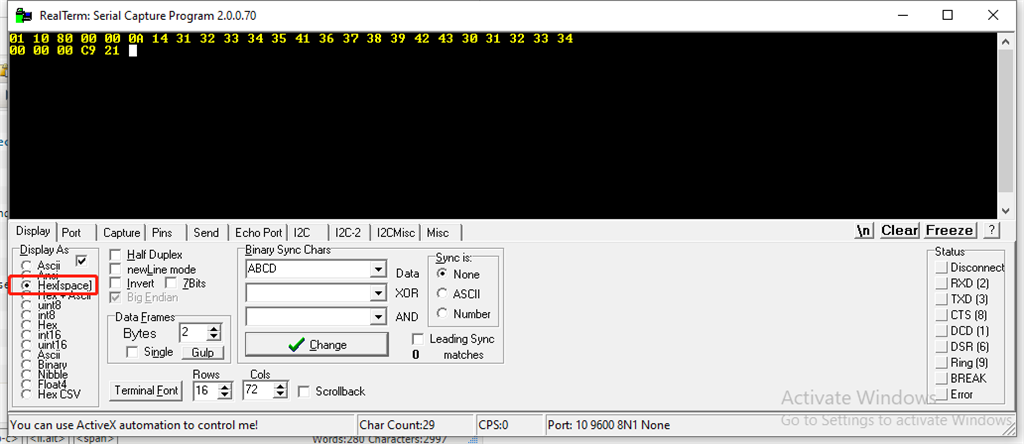
Now i want to use user input by means of textbox.
The value i will get from textbox will be a string value
like string dataFromTBox = tboxDataOut;
I want to know how to do further process in order to seperate each number or alphabet then convert it into hex and send it using serial port. Value send to serial port should be in byte form not in string form.