Hi everyone. I am new to programming. I need your help regarding the creation of Custom MyStrings class in C Sharp which mimics the behaviour of String class. I have tried the following code but it is not giving proper output as expected. Please help me through this . Many thanks
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.InteropServices;
using System.Text;
using System.Threading.Tasks;
namespace CP
{
internal class MyStrings
{
#region(Fields)
#endregion
#region(Properties)
#endregion
#region(Constructors)
#endregion
#region(Index operator)
#endregion
#region(Implicit convertors)
#endregion
#region(Methods)
#endregion
#region(Operators)
#endregion
public char[] chars;
// Constructor that takes no argument
public MyStrings()
{
chars = new char[0];
}
// Index operator
public char this[int index]
{
get { return chars[index]; }
set { chars[index] = value; }
}
// Constructor that takes a single character
public MyStrings(char c)
{
chars = new char[] { c };
}
// Constructor that takes an array of characters
public MyStrings(char[] input)
{
chars = input;
}
// Write() method
public void Write()
{
Console.WriteLine(chars);
}
public int Length
{
get { return chars.Length; }
}
// operator that concatenates two MyStringss
public static MyStrings operator +(MyStrings s1, MyStrings s2)
{
char[] newChars = new char[s1.Length + s2.Length];
Array.Copy(s1.chars, newChars, s1.Length);
Array.Copy(s2.chars, 0, newChars, s1.Length, s2.Length);
return new MyStrings(newChars);
}
// ToCharArray() method
public char[] ToCharArray()
{
char[] newChars = new char[chars.Length];
Array.Copy(chars, newChars, chars.Length);
return newChars;
}
// Implicit conversion from MyString to string
public static implicit operator string(MyStrings s)
{
return new string(s.chars);
}
// Implicit conversion from string to MyStrings
public static implicit operator MyStrings(string s)
{
return new MyStrings(s.ToCharArray());
}
// Implicit conversion from char[] to MyStrings
public static implicit operator MyStrings(char[] chars)
{
return new MyStrings(chars);
}
// ToLower() method
public MyStrings ToLower()
{
char[] result = new char[Length];
for (int i = 0; i < Length; i++)
{
char c = chars[i];
if (c >= 'A' && c <= 'Z')
{
// Convert uppercase letter to lowercase letter
c = (char)(c - ('A' - 'a'));
}
result[i] = c;
}
return new MyStrings(result);
}
// ToUpper() method
public MyStrings ToUpper()
{
char[] result = new char[Length];
for (int i = 0; i < Length; i++)
{
char c = chars[i];
if (c >= 'a' && c <= 'z')
{
// Convert lowercase letter to uppercase letter
c = (char)(c - ('a' - 'A'));
}
result[i] = c;
}
return new MyStrings(result);
}
// Equals() method override
public override bool Equals(object obj)
{
if (obj == null || GetType() != obj.GetType())
{
return false;
}
MyStrings other = (MyStrings)obj;
if (Length != other.Length)
{
return false;
}
for (int i = 0; i < Length; i++)
{
if (chars[i] != other[i])
{
return false;
}
}
return true;
}
// Reverse() method
public MyStrings Reverse()
{
char[] result = new char[Length];
for (int i = Length - 1, j = 0; i >= 0; i--, j++)
{
result[j] = chars[i];
}
return new MyStrings(result);
}
public static void Main(string[] args)
{
MyStrings s = new MyStrings();
Console.WriteLine("Should print nothing: {0}", s);
// Create a constructor that takes array of characters
char[] a = { 'M', 'y', ' ', 'n', 'a', 'm', 'e' };
MyStrings s2 = new MyStrings(a);
Console.WriteLine("Should print 'My name': {0}", s2);
// Write an operator '+' that concatenates two MyStrings
MyStrings s3 = s + s2;
Console.WriteLine("Should print 'My name': {0}", s3);
s3 = s2 + " " + s3;
Console.WriteLine("Should print 'My name My name': {0}", s3);
string s4 = "Hey, " + s3; // S3 is MyStrings. Should use implicit convertor.
Console.WriteLine("Should print 'Hey, My name My name': {0}", s4);
Console.WriteLine("Printing s3: {0}", s3);
Console.WriteLine("Should print 'M': {0}", s3[8]);
char[] a2 = s3.ToCharArray();
Console.WriteLine("Should print 'M': {0}", a2[8]);
MyStrings s5 = new MyStrings("ABC".ToCharArray());
Console.WriteLine("Should print 'ABC': {0}", s5);
s5.ToLower(); //note s5 doesn't change
MyStrings s6 = s5.ToLower();
Console.WriteLine("Should print 'ABC': {0}", s5);
Console.WriteLine("Should print 'abc': {0}", s6);
Console.WriteLine("Should print 'ABC': {0}", s6.ToUpper());
s5 += s6; //note we get this operator for free
Console.WriteLine("Should print 'ABCabc': {0}", s5);
Console.WriteLine("Should print 'True': {0}", s5.Equals(s5));
Console.WriteLine("Should print 'False': {0}", s5.Equals(s6));
MyStrings s7 = new MyStrings("ZYX".ToCharArray());
MyStrings s8 = new MyStrings("ZYX".ToCharArray());
Console.WriteLine("Should print 'True': {0}", s7.Equals(s8));
MyStrings s9 = s5.Reverse();
Console.WriteLine("Should print 'cbaCBA': {0}", s9);
Console.WriteLine("Should print 'ABCabc': {0}", s9.Reverse());
}
}
}
But I am not getting the expected output as commented in the main method .
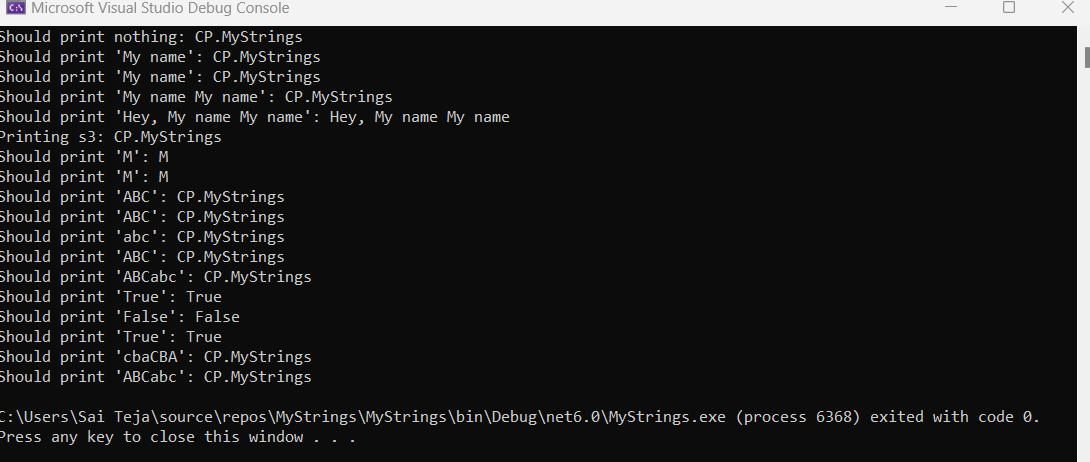
Please help me where is the issue with the code snippet. Thanks in advance.