
I am trying to create a C# program that does the following...
Every time the program is run it must
1. Ask the user to enter a number.
2. Read the previous number that was written to disk by the program the previous time it ran.
3. Add the entered number to the previous number. This will give you a total number.
4. If the total number is greater than 100 then subtract 100 from the total number.
5. Display the total number to the user.
6. Save the total number to disk.
7. Exit.
I have got a plan for this as below
1)Have the exisring data in a txt file with column headers for 1)Previous value 2) New User input 3)Calculations 4) New value
Add a Class Library to have all logic for file IO.
Add a new model say NumberInfo.txt with 4 properties as column headers
Add a class for textfileData Access to handle FileIO operations like ReadAllRecords, WriteAllRecords
2) Add a console app that will be used as a UI.
It will have file info in appsettings.json
Program.cs will be the entry point & will have methods for data access like creating numbers, removing numbers fromNumberInfotextfile, UpdateAnyColumnsinNumberInfo, GetAllNumbersFromNumberInfo , CreateNumbers, InitiaiseConfig etc.. 
I would like to start in Program.cs to load the file if it exists.
If file does not exist, it should be created.
I am a bit unsure about the following & would appreciate some guidance for this.
1)If I am on the right track for this?
2)Where I should add the do while loop to check the validation for valid input for an int i.e 22 not Twenty two?
3)If the previous number is null, how & where this should be handled?
4) What should be the delimitor for calculations column instead of space.
5)Where to add the column header in the console output if I need to display multiple lines?
Thanks in advance
public class NumberInfoTextFileDataAccess
{
public List<NumberInfoModel> ReadAllRecords(string textFile)
{
//check if file exists
if (File.Exists(textFile) == false)
{
//return an empty list..it will help to avoid throwign exception
//as it is an empty list, it returns zero lines
return new List<NumberInfoModel>();
}
var lines = File.ReadAllLines(textFile);
List<NumberInfoModel> numberInfoOutput = new List<NumberInfoModel>();
foreach (var line in lines)
{
NumberInfoModel numberInfo = new NumberInfoModel();
var vals = line.Split('|');
if (vals.Length < 4)
{
throw new Exception($"Invalid row of data: { line }");
}
numberInfo.PreviousNumber = vals[0];
numberInfo.UserInput = vals[1];
numberInfo.Calculations = vals[2].Split("").ToList();
numberInfo.NewValue = vals[3];
numberInfoOutput.Add(numberInfo);
}
return numberInfoOutput;
//bool isValidNum;
//int num;
//if (File.Exists(textFile) == false)
//{
// return new List<ContactModel>();
//}
//var lines = File.ReadAllLines(textFile);
//List<ContactModel> output = new List<ContactModel>();
//foreach (var line in lines)
//{
// ContactModel c = new ContactModel();
// var vals = line.Split(',');
// if (vals.Length < 4)
// {
// throw new Exception($"Invalid row of data: { line }");
// }
// //added this
// if (vals[1] == null)
// {
// do
// {
// Console.WriteLine("Please enter a value");
// vals[1] = Console.ReadLine();
// isValidNum = int.TryParse(vals[1], out num);
// if (isValidNum == false)
// {
// Console.WriteLine("That was an invalid number.");
// }
// } while (isValidNum == false);
// }
// //added upto here
// c.FirstName = vals[0];
// c.LastName = vals[1];
// c.EmailAddresses = vals[2].Split(';').ToList();
// c.PhoneNumbers = vals[3].Split(';').ToList();
// output.Add(c);
}
public void WriteAllRecords(List<NumberInfoModel> numbers, string textFile)
{
//|5 |55 |+55 |60 |
/*
| Previous value | User input | Calculations | New value |
|------------------------|------------------|--------------|-------------------|
| | 5 | +5 | 5 |
|5 | 9 | +9 | 14 |
|14 | 130 | +130 -100 | 144 |
|144 | 20 | +20, -100 | 12 |
|------------------------|------------------|--------------|-------------------|
*/
List<string> lines = new List<string>();
Console.WriteLine(" | Previous value in file | User input value | Calculations | New value in file | ");
foreach (var num in numbers)
{
//lines.Add($"|{ num.PreviousNumber },|{ num.UserInput },{ String.Join(';', c.EmailAddresses) },{ String.Join(';', c.PhoneNumbers) }");
//lines.Add($"|{ num.PreviousNumber },|{ num.UserInput },");
lines.Add($"|{ num.PreviousNumber },|{ num.UserInput },|{ String.Join(' ', num.Calculations) },|{ num.NewValue }");
}
File.WriteAllLines(textFile, lines);
}
}
public class NumberInfoModel
{
public string PreviousNumber { get; set; }
public string UserInput { get; set; }
public List<string> Calculations { get; set; } = new List<string>();
public string NewValue { get; set; }
}
class Program
{
private static IConfiguration _config;
private static string textFile;
private static NumberInfoTextFileDataAccess db = new NumberInfoTextFileDataAccess();
static void Main(string[] args)
{
InitializeConfiguration();
textFile = _config.GetValue<string>("TextFile");
//Creating NumberInfo
Console.WriteLine("Enter number");
NumberInfoModel numberInfo1 = new NumberInfoModel();
numberInfo1.PreviousNumber = "20";
numberInfo1.UserInput = Console.ReadLine();
numberInfo1.Calculations.Add("+" + numberInfo1.UserInput);
numberInfo1.Calculations.Add("+100");
numberInfo1.NewValue = ("120");
List<NumberInfoModel> numbers = new List<NumberInfoModel>
{
numberInfo1
};
//Start Processing
//Create Number Info
CreateNumberInfo(numberInfo1);
//Get All Numbers
GetAllNumbers();
Console.ReadLine();
}
public static void RemoveNumberInfo()
{
var numbers = db.ReadAllRecords(textFile);
numbers.RemoveAt(0);
db.WriteAllRecords(numbers, textFile);
}
public static void RemoveCalculationsFromNumberInfo(string calculation)
{
var numbers = db.ReadAllRecords(textFile);
numbers[0].Calculations.Remove(calculation);
db.WriteAllRecords(numbers, textFile);
}
private static void UpdateNumberInfoPreviousNumber(string previousNumber)
{
var numbers = db.ReadAllRecords(textFile);
numbers[0].PreviousNumber = previousNumber;
db.WriteAllRecords(numbers, textFile);
}
private static void GetAllNumbers()
{
var numbers = db.ReadAllRecords(textFile);
//Console.WriteLine("| Previous value in file | User input value | Calculations | New value in file |");
//Console.WriteLine("|------------------------|------------------|--------------|-------------------|");
foreach (var number in numbers)
{
//Console.WriteLine("Please enter a value");
//if (Int32.Parse(number.UserInput) > 100)
// if (Try.Parse(number.UserInput) > 100)
Console.WriteLine($"|{ number.PreviousNumber } | { number.UserInput } | + {number.UserInput} -100 | {number.NewValue}");
//else
// Console.WriteLine($"|{ number.PreviousNumber} |{ number.UserInput} |+ {number.UserInput} |{number.NewValue}");
}
}
private static void CreateNumberInfo(NumberInfoModel numberInfoModel)
{
var numbers = db.ReadAllRecords(textFile);
numbers.Add(numberInfoModel);
db.WriteAllRecords(numbers, textFile);
}
private static void InitializeConfiguration()
{
var builder = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json");
_config = builder.Build();
}
}
}
{
"TextFile": "C:\\Temp\\NumberInfo.txt"
}
SolutionDetails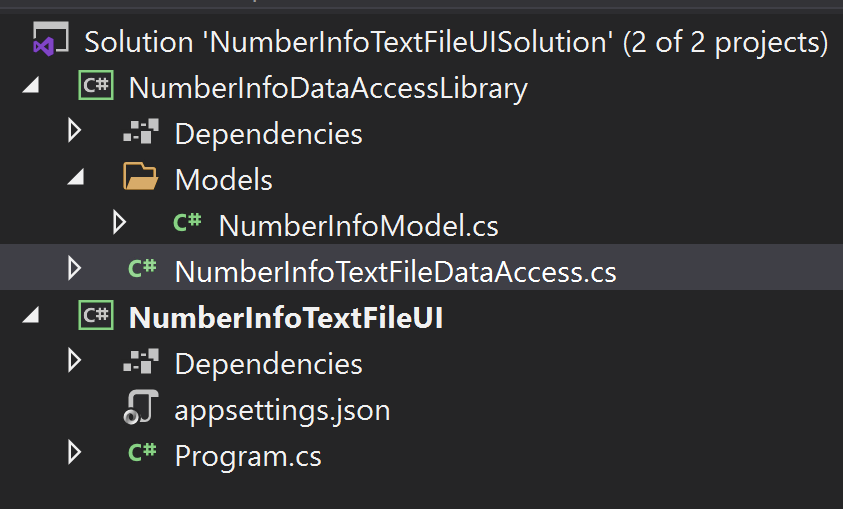