Good afternoon, I need to show master detail data, I already have the data in the database. how do you see next
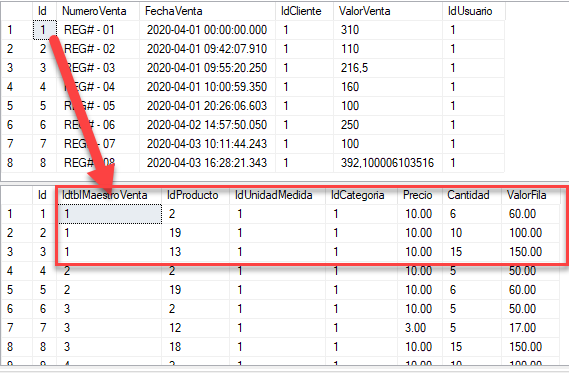
I also have the classes in the model
- namespace CapaDatos
- {
- using System;
- using System.Collections.Generic;
-
- public partial class tblMaestroVenta
- {
- [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")]
- public tblMaestroVenta()
- {
- this.tblDetalleVentas = new HashSet();
- }
-
- public int Id { get; set; }
- public string NumeroVenta { get; set; }
- public System.DateTime FechaVenta { get; set; }
- public Nullable<int> IdCliente { get; set; }
- public double ValorVenta { get; set; }
- public int IdUsuario { get; set; }
-
- public virtual tblCliente tblCliente { get; set; }
- [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly")]
- public virtual ICollection tblDetalleVentas { get; set; }
- }
- }
a MasterSale class that inherits from tblMaestroventa
- namespace CapaLogica
- {
- public class MaestroVenta : tblMaestroVenta
- {
- public string NombreCliente { get; set; }
- public string NombreUsuario { get; set; }
-
- public static int GuardarMaestroVenta(MaestroVenta maestroVenta)
- {
- using (GourmetEntities db = new GourmetEntities())
- {
- try
- {
- var returnCode = new SqlParameter("@ReturnValueId", SqlDbType.Int);
- returnCode.Direction = ParameterDirection.Output;
- db.Database.ExecuteSqlCommand("Sp_InsertarMaestroVenta @FechaVenta,@IdCliente,@ValorVenta,@IdUsuario, @ReturnValueId OUTPUT",
- new SqlParameter("@FechaVenta", maestroVenta.FechaVenta),
- new SqlParameter("@IdCliente", maestroVenta.IdCliente),
- new SqlParameter("@ValorVenta", maestroVenta.ValorVenta),
- new SqlParameter("@IdUsuario", maestroVenta.IdUsuario),
- returnCode);
- db.SaveChanges();
- return (int)returnCode.Value;
- }
- catch (Exception exp)
- {
- var me = exp.Message;
- throw;
- }
-
-
- }
- }
- public static tblMaestroVenta MaestroDetalle(int Id)
- {
- using (GourmetEntities db = new GourmetEntities())
- {
- return db.tblMaestroVentas.Include(x => x.tblDetalleVentas)
- .FirstOrDefault(x => x.Id == Id);
- }
- }
-
- }
- }
I also have the detail class
- namespace CapaDatos
- {
- using System;
- using System.Collections.Generic;
-
- public partial class tblDetalleVenta
- {
- public int Id { get; set; }
- public int IdtblMaestroVenta { get; set; }
- public int IdProducto { get; set; }
- public int IdUnidadMedida { get; set; }
- public int IdCategoria { get; set; }
- public decimal Precio { get; set; }
- public int Cantidad { get; set; }
- public decimal ValorFila { get; set; }
-
- public virtual tblCategoria tblCategoria { get; set; }
- public virtual tblMaestroVenta tblMaestroVenta { get; set; }
- public virtual tblProducto tblProducto { get; set; }
- public virtual tblUnidad tblUnidad { get; set; }
- }
- }
A SalesDetail class inherited from tblDetalleVenta
- namespace CapaLogica
- {
- public class DetalleVenta : tblDetalleVenta
- {
-
- public string Codigo { get; set; }
- public string Descripcion { get; set; }
-
- public string Unidad { get; set; }
- public decimal TotalFila { get { return Precio * (decimal)Cantidad; } }
- public static void InsertarDetalleVenta(tblDetalleVenta detalleVenta)
- {
- using (GourmetEntities db = new GourmetEntities())
- {
- db.Sp_InsertarDetalleVenta(detalleVenta.IdtblMaestroVenta, detalleVenta.IdProducto, detalleVenta.IdUnidadMedida, detalleVenta.IdCategoria, detalleVenta.Precio, detalleVenta.Cantidad, detalleVenta.ValorFila);
- }
- }
-
-
- }
- }
I have a method that returns the query
- public static tblMaestroVenta MaestroDetalle(int Id)
- {
- using (GourmetEntities db = new GourmetEntities())
- {
- return db.tblMaestroVentas.Include(x => x.tblDetalleVentas)
- .FirstOrDefault(x => x.Id == Id);
- }
- }
I use all this with entity Framework
now I have my RDLC Report
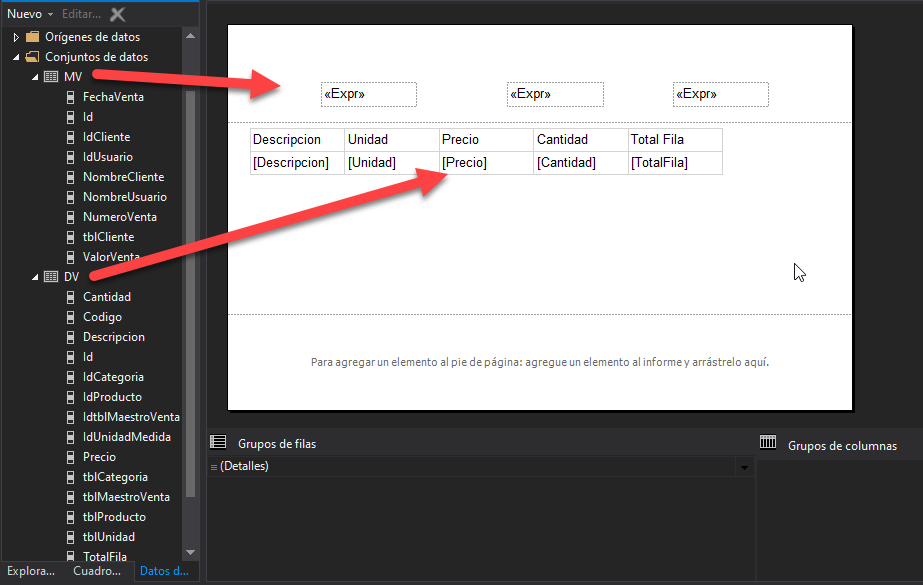
to load in the form I made this code
- private void FrmRptVenta_Load(object sender, EventArgs e)
- {
- MaestroVentaBindingSource.DataSource = MaestroVenta.MaestroDetalle(1);
- DetalleVentaBindingSource.DataSource = MaestroVenta.MaestroDetalle(1);
- this.reportViewer1.RefreshReport();
- }
but only load part of master as you can see in the following image
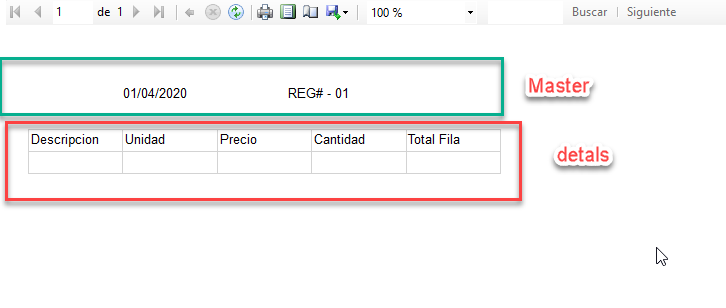
Please can someone help me to solve this problem, or can you give me an example of how to solve this problem please. I appreciate the collaboration
Robert