Factory Pattern:
According to GoF, the definition of factory pattern is "Creates an instance of several derived classes".
or
"Define an interface for creating an object, but let subclasses decide which class to instantiate. Factory Method lets a class defer instantiation to subclasses".
The Factory Pattern is a Creational Pattern that simplifies object creation. You need not worry about the object creation; you just need to supply an appropriate parameter and factory to give you a product as needed.
Suppose you (the client) are in a restaurant and you need some food according to your choice, for that you must know the name of the food (parameters) or you can demand a menu from waiter to see all available food options. Now suppose you need "Curd", "Rice" some "Bread" and "Mix Vegetables", you place the order to the waiter (Creator/Subclass), and you get all the desired foods in your plate. So here you need not worry about food preparation (Object Creation).
Here I have created an example with library and books; here two libraries (specialized books library and General Books Library) are available to you as a product, now you have to supply appropriate parameters to select, whether you need general books library or a specialized books library.
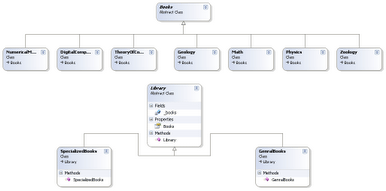
Code Example:
Books options available:
abstract class Books
{
public virtual string BookName()
{
return "Abstract Book";
}
}
class Geology : Books
{
public override string BookName()
{
return "Books Name : Geology";
}
}
class Zoology : Books
{
public override string BookName()
{
return"Books Name : Zoology";
}
}
class Physics : Books
{
public override string BookName()
{
return "Books Name : Physics";
}
}
class Math : Books
{
public override string BookName()
{
return "Books Name : Math";
}
}
class DigitalComputer : Books
{
public override string BookName()
{
return "Books Name : DigitalComputer";
}
}
class NumericalMethods : Books
{
public override string BookName()
{
return "Books Name : NumericalMethods";
}
}
class TheoryOfComputation : Books
{
public override string BookName()
{
return "Books Name : TheoryOfComputation";
}
}
Different libraries available to categorize the books:
abstract class Library
{
List<Books> _books = new List<Books>();
public Library()
{
}
public List<Books> Books
{
get { return _books; }
}
}
class GenralBooks : Library
{
public GenralBooks()
{
Books.Add(new Geology());
Books.Add(new Zoology());
Books.Add(new Physics());
Books.Add(new Math());
}
}
class SpecializedBooks : Library
{
public SpecializedBooks()
{
Books.Add(new DigitalComputer());
Books.Add(new NumericalMethods());
Books.Add(new TheoryOfComputation());
}
}
Main Program to access the methods available with different books:
class Program
{
static void Main(string[] args)
{
Library[] objLib = new Library[2];
objLib[0] = new GenralBooks();
objLib[1] = new SpecializedBooks();
//objLib[0].CreateBooksCategories();
//objLib[1].CreateBooksCategories();
foreach (Library lib in objLib)
{
Console.WriteLine("\n" + lib.GetType().Name + "--");
foreach (Books objBook in lib.Books)
{
Console.WriteLine(objBook.BookName());
}
}
Console.ReadLine();
}
}