Async Await In C#
C# Async Await
Nowadays, Asynchronous programming is very popular with the help of the async and await keywords in C#.
When we are dealing with UI and on button click, we use a long running method like reading a large file or something else which will take a long time, in that case, the entire application must wait to complete the whole task.
In other words, if any process is blocked in a synchronous application, the entire application gets blocked and our application stops responding until the whole task completes.
Asynchronous programming is very helpful in this condition. By using Asynchronous programming, the Application can continue with the other work that does not depend on the completion of the whole task.
We will get all the benefits of traditional Asynchronous programming with much less effort by the help of async and await keywords.
Suppose, we are using two methods as Method1 and Method2 respectively and both the methods are not dependent on each other and Method1 is taking a long time to complete its task. In Synchronous programming, it will execute the first Method1 and it will wait for completion of this method and then it will execute Method2. Thus, it will be a time intensive process even though both the methods are not depending on each other.
We can run all the methods parallelly by using the simple thread programming but it will block UI and wait to complete all the tasks. To come out of this problem, we have to write too many codes in traditional programming but if we will simply use the async and await keywords, then we will get the solutions in much less code.
Also, we are going to see more examples, if any third Method as Method3 has a dependency of method1, then it will wait for the completion of Method1 with the help of await keyword.
Async and await are the code markers, which marks code positions from where the control should resume after a task completes.
Let’s start with practical examples for understanding the programming concept.
Code examples of C# async await
We are going to take a console application for our demonstration.
Example 1
In this example, we are going to take two methods, which are not dependent on each other.
Code sample
- class Program
- {
- static void Main(string[] args)
- {
- Method1();
- Method2();
- Console.ReadKey();
- }
- public static async Task Method1()
- {
- await Task.Run(() =>
- {
- for (int i = 0; i < 100; i++)
- {
- Console.WriteLine(" Method 1");
- }
- });
- }
- public static void Method2()
- {
- for (int i = 0; i < 25; i++)
- {
- Console.WriteLine(" Method 2");
- }
- }
- }
Here, we can clearly see Method1 and Method2 are not waiting for each other.
Output
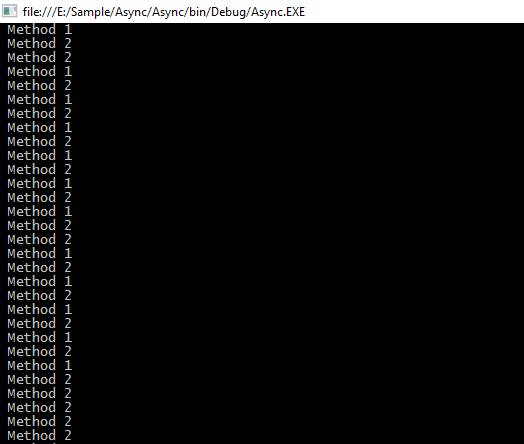
Now, coming to the second example, suppose we have Method3, which is dependent on Method1
Example 2
In this example, Method1 is returning total length as an integer value and we are passing a parameter as a length in a Method3, which is coming from Method1.
Here, we have to use await keyword before passing a parameter in Method3 and for it, we have to use the async keyword from the calling method.
We can not use await keyword without async and we cannot use async keyword in the Main method for the console Application because it will give the error given below.

We are going to create a new method as callMethod and in this method, we are going to call our all Methods as Method1, Method2, and Method3 respectively.
Code sample
- class Program
- {
- static void Main(string[] args)
- {
- callMethod();
- Console.ReadKey();
- }
- public static async void callMethod()
- {
- Task<int> task = Method1();
- Method2();
- int count = await task;
- Method3(count);
- }
- public static async Task<int> Method1()
- {
- int count = 0;
- await Task.Run(() =>
- {
- for (int i = 0; i < 100; i++)
- {
- Console.WriteLine(" Method 1");
- count += 1;
- }
- });
- return count;
- }
- public static void Method2()
- {
- for (int i = 0; i < 25; i++)
- {
- Console.WriteLine(" Method 2");
- }
- }
- public static void Method3(int count)
- {
- Console.WriteLine("Total count is " + count);
- }
- }
Output
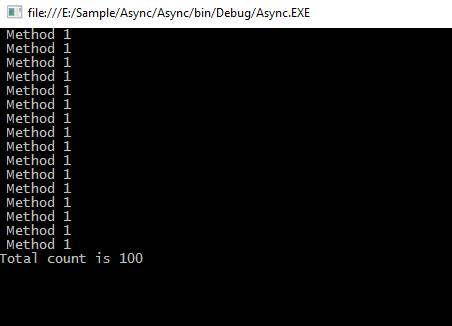
Real time example
There are some supporting API's from the .NET Framework 4.5 and the Windows runtime contains methods that support async programming.
We can use all of these in the real time project with the help of async and await keyword for the faster execution of the task.
Some APIs that contain async methods are HttpClient, SyndicationClient, StorageFile, StreamWriter, StreamReader, XmlReader, MediaCapture, BitmapEncoder, BitmapDecoder etc.
In this example, we are going to read all the characters from a large text file asynchronously and get the total length of all the characters.
Sample code
- class Program
- {
- static void Main()
- {
- Task task = new Task(CallMethod);
- task.Start();
- task.Wait();
- Console.ReadLine();
- }
- static async void CallMethod()
- {
- string filePath = "E:\\sampleFile.txt";
- Task<int> task = ReadFile(filePath);
- Console.WriteLine(" Other Work 1");
- Console.WriteLine(" Other Work 2");
- Console.WriteLine(" Other Work 3");
- int length = await task;
- Console.WriteLine(" Total length: " + length);
- Console.WriteLine(" After work 1");
- Console.WriteLine(" After work 2");
- }
- static async Task<int> ReadFile(string file)
- {
- int length = 0;
- Console.WriteLine(" File reading is stating");
- using (StreamReader reader = new StreamReader(file))
- {
- // Reads all characters from the current position to the end of the stream asynchronously
- // and returns them as one string.
- string s = await reader.ReadToEndAsync();
- length = s.Length;
- }
- Console.WriteLine(" File reading is completed");
- return length;
- }
- }
In our sampleText.txt, file contains too many characters, so It will take a long time to read all the characters.
Here, we are using async programming to read all the contents from the file, so it will not wait to get a return value from this method and execute the other lines of code but it has to wait for the line of code given below because we are using await keyword and we are going to use the return value for the line of code given below..
- int length = await task;
- Console.WriteLine(" Total length: " + length);
Subsequently, other lines of code will be executed sequentially.
- Console.WriteLine(" After work 1");
- Console.WriteLine(" After work 2");
Output
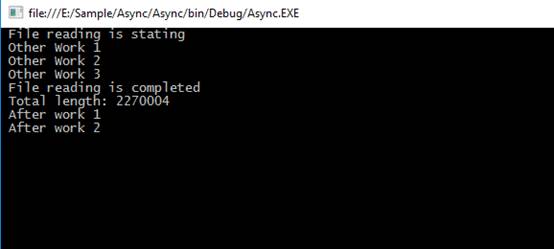
Here, we have to understand very important points that if we are not using await keyword, then the method works as a synchronous method. The compiler will show the warning to us but it will not show any error.
In this easy way, we can use async and await keywords in C# to implement async programming.
If you're new to Async programming, here is a detailed tutorial - Tutorial on Asynchronous programming in C#.
Author
Vivek Kumar
159
11.8k
5.7m