Asynchronous Programming in C# 5.0 - Access Data Sing Asynchronous Function
Welcome to the Asynchronous Programming in C# 5.0 article series. In previous articles we discussed many more things in asynchronous programming. You can read them here.
- Asynchronous programming in C# 5.0: Part-1: Understand async and await
- Asynchronous Programming in C# 5.0 Part 2: Return Type of Asynchronous Method
- Asynchronous Programming in C# 5.0 Part 3: Understand Task in Asynchronous programming.
- Asynchronous Programming in C# 5.0 Part 4:Exception Handling in Asynchronous Programming
In this article we will try to implement a few real-time applications of asynchronous programming. Here we will see in which scenario we can implement asynchronous threads.
Let's try to understand the situation.
To call a Web service
If we want to call a web service then we can implement an asynchronous function. Because we know that, to call a web service it takes time (for other reasons, like low internet speed, server down and so on).
And if we want our application's performance to not decrease due to low response of the web service then we can implement asynchronous functions or we can call this web service asynchronously.
To pull huge amounts of data from a database
Let's think of one situation where in one form (Windows or Web Form for example) a huge amount of data needs to be pulled from the database. Now if we access the data synchronously then the entire UI will block and the user is unable to do anything until all database operations finish.
But if we do it asynchronously (or call the data fetching function asynchronously) then the user is free to do her work while the data is loading in the background.
To read file
Sometimes it's necessary to read a large file in an application. Reading the file using the StreamReader object takes time. We can read this file asynchronously then it will not affect the performance of the application.
Hope those three examples are enough to understand the real time implementation of asynchronous functions. Now let's implement asynchronous functions in a few scenarios.
Fetch data asynchronously
We will create one sample program to fetch data asynchronously. We have created two functions to load data in the grid asynchronously. Let's create a simple user interface containing two grids and one button. When we press a button the grid will load asynchronously. Have a look at the following code.
- using System;
- using System.ComponentModel;
- using System.Threading.Tasks;
- using System.Windows.Forms;
- using System.Data;
- namespace WindowsFormsApplication1
- {
- public partial class Form1 : Form
- {
- DataTable dt = new DataTable();
- public Form1()
- {
- InitializeComponent();
- dt.Columns.Add("Id", typeof(int));
- dt.Columns.Add("Name", typeof(String));
- dt.Columns.Add("Surname", typeof(String));
- dt.Rows.Add(1, "sourav", "kayal");
- dt.Rows.Add(2, "Ram", "Kumar");
- dt.Rows.Add(3, "Shyam", "Kymar");
- }
- private void Form1_Load(object sender, EventArgs e)
- {
- }
- public Task<DataTable> LoadData1()
- {
- return Task.Run(() => {
- System.Threading.Thread.Sleep(10000);
- return dt;
- });
- }
- public Task<DataTable> LoadData2()
- {
- return Task.Run(() =>
- {
- System.Threading.Thread.Sleep(10000);
- return dt;
- });
- }
- private async void button1_Click(object sender, EventArgs e)
- {
- //Load Data Asynchronously
- this.dataGridView1.DataSource = await LoadData1();
- this.dataGridView2.DataSource = await LoadData2();
- }
- }
- }
Here is sample output.
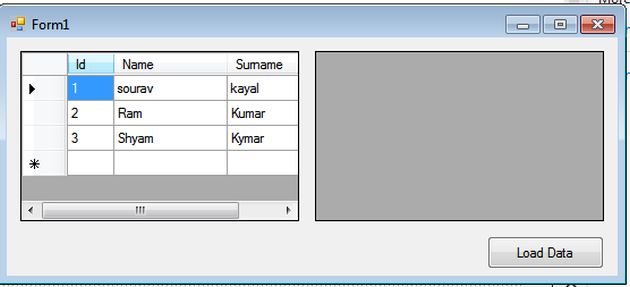
We are seeing that one grid has loaded and still data is not available in the second grid and the most noticeable fact is that, the user interface is not collapsed in the data fetch operation. We can drag this window anywhere and even resize it.
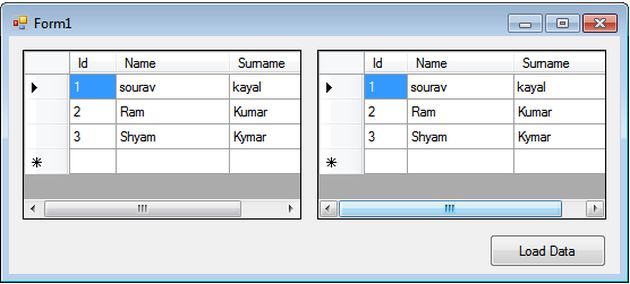
Now data has been populated in both grids.
So, this is an actual example of an asynchronous function. Here we have loaded static data using a data table but in practical ujse you may implement data fetching operations from a database.
Conclusion
In this article we learned how to access data asynchronously in C#. Hope you have understood the concept. In future articles we will see a few more scenarios where we can implement the asynchronous mechanism.
Author
Sourav Kayal
0
27.7k
24.6m