Functions in JavaScript
Introduction
In this chapter, we learn about Functions in JavaScript. Function refers to a set of Statements, to perform some specific task. When a JavaScript code is executed, the instructions are carried out inside the main function. There are many ways we can create a function in JavaScript.
- Function Declaration
- Function Parameters
- Function Return Statement
Function
A function is a block of code designed to perform a specific task. It enhances code reuse in functions, and the same function can be used multiple times within the program. Any function created using different methods of JavaScript functions will be one of the functions below:
- A function without parameters and without returning value
- A function without parameters and with returning value
- A function with parameters and with returning value
- A function with parameters and without returning value
Uses of Function
- Code reusability
- You can eliminate the need to write the same code.
- The function can be called anywhere in the program.
Variables using function
- Local variable- using inside the function.
- Global variable- using outside the function.
Function Declaration
Using the ‘function’ keyword, followed by a set of ‘function name’ and ‘parentheses ()’. The block of code to be executed inside the Curly braces {}.
Syntax
- function name () // inside the brazes pass the parameters/arguments
- {
- //code to be executed;
- }
A function name can contain a letter, digit, underscore, and dollar symbol only. Don’t use any other special characters.
Example 1
Try it yourself:
- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="utf-8">
- <title>Functions</title>
- </head>
- <body>
- <h2>Functions in JavaScript</h2>
- <script type="text/javascript">
- function myfunction() //define a function
- {
- document.write("Hello World");
- }
- </script>
- </body>
- </html>
Output
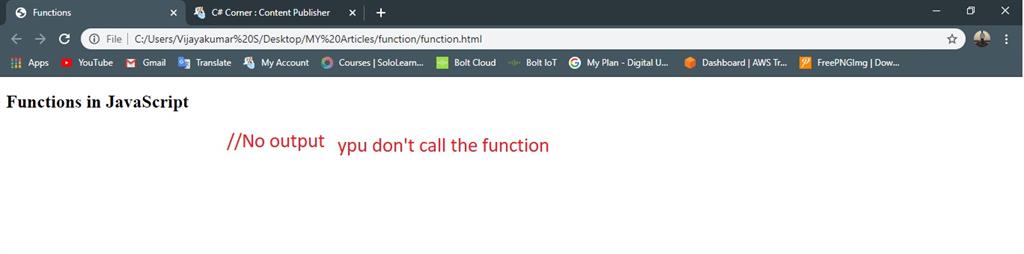
Calling a Function
In Example 1, we have a function, but there is no output. Why? To execute the function, you need to call the function. To call a function, start with the name of the function, then follow it with the function arguments in parentheses.
Example 2
Try it yourself:
- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="utf-8">
- <title>Functions</title>
- </head>
- <body>
- <h2>Functions in JavaScript</h2>
- <script type="text/javascript">
- function myfunction() // declare a function
- {
- document.write("Hello World");
- }
- myfunction(); // calling a function
- </script>
- </body>
- </html>
Output
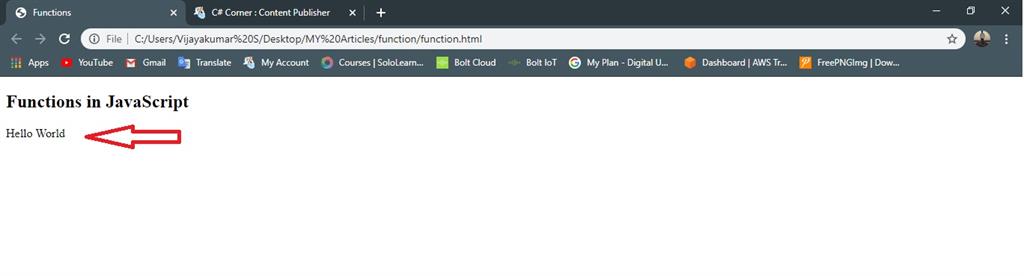
Example 2.1
Try it yourself:
- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="utf-8">
- <title>Functions</title>
- <script type="text/javascript">
- function myfunction()
- {
- document.write("Hello World");
- }
- myfunction();
- </script>
- </head>
- <body>
- <h2>Functions in JavaScript</h2>
- <p>Click on the button Show the message</p>
- <button onclick="myfunction()"> Click_Here</button> // calling a function
- </body>
- </html>
Output
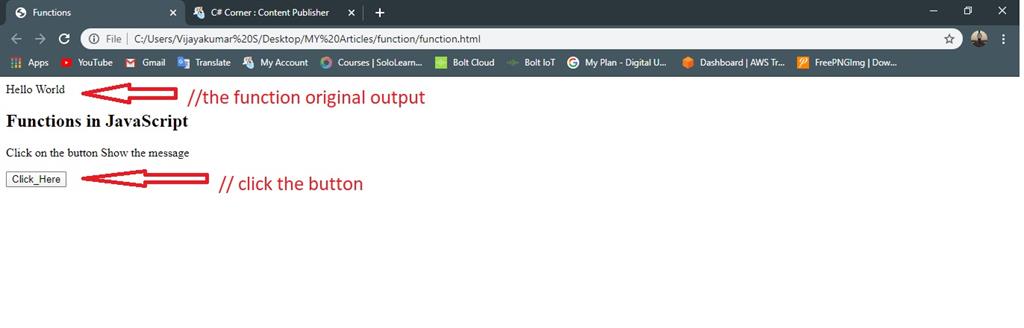
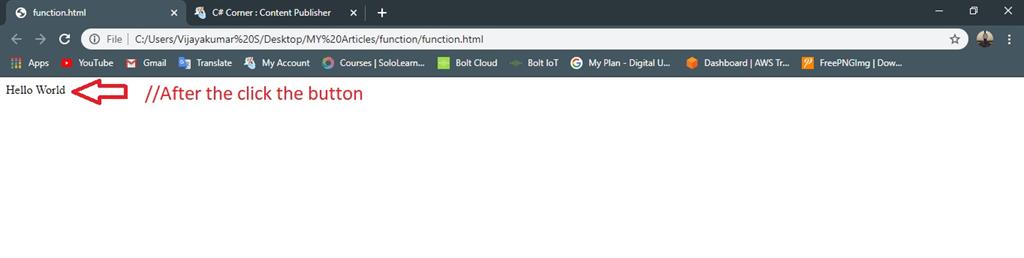
Function Parameters
A function can take a parameter, which is just values passing to the function. Parameters are specified into the parentheses. It is provided in the argument field of the function.
Syntax
- function name (pram 1, param 2, param 3,) {
- // block of code
- }
Example 3
Try it yourself:
- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="utf-8">
- <title>Functions in Parameters</title>
- </head>
- <body>
- <h1>Passing the Parameters in function</h1>
- <script type="text/javascript">
- function sayHello(name, age) // parameters
- {
- alert( name + " is " + age + " years old.");
- }
- sayHello("NaveenKumar", 23) // calling a function
- sayHello("VijayKumar", 21)
- sayHello("Surya", 21)
- </script>
- </body>
- </html>
Output
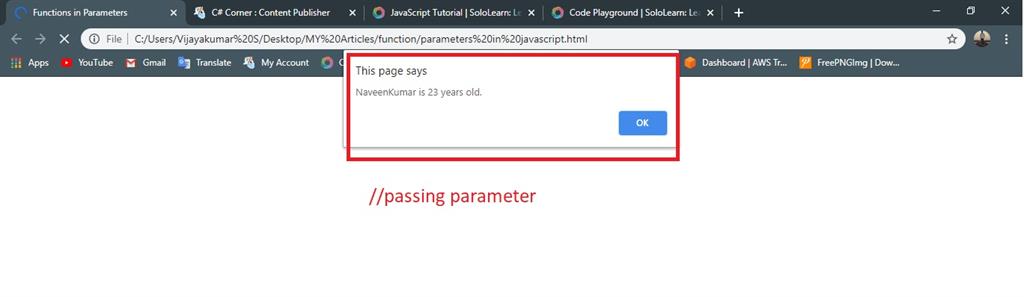
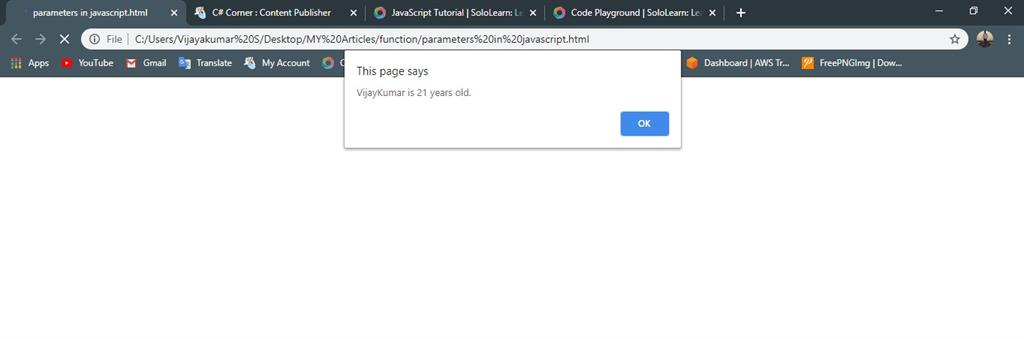
Default Parameters
The default parameter is nothing but to set a default value for the function parameter. If the value is not passed, it is undefined.
Syntax
- function name (param1 = value1, param2 = value2, param3 = value3)
- {
- //Statements;
- }
Example 3.1
Try it yourself:
- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="utf-8">
- <title>Functions in Parameters</title>
- </head>
- <body>
- <h1>Default Parameters in function</h1>
- <script type="text/javascript">
- function sayHello(name, age =20) // default parameters
- {
- document.write( name + " is " + age + " years old.");
- }
- sayHello("vijay"); // not assign value for default parameter
- </script>
- </body>
- </html>
Output
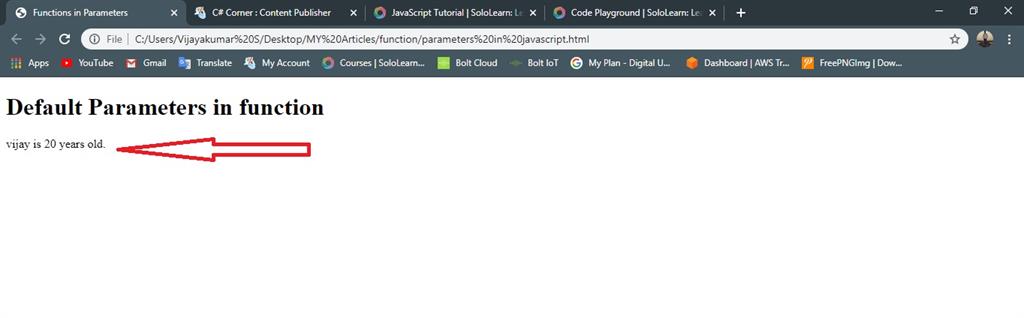
The Return Statement
The return statement is used to return a value from the function. You need to make a calculation and receive the result.
Example 4
Try it yourself:
- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="utf-8">
- <title>Functions in Parameters</title>
- </head>
- <body>
- <h1>Return Statememnt in function</h1>
- <script type="text/javascript">
- function myfunction(a,b) {
- return a*b; //return statement
- }
- document.write("Product of Two Numbers"+myfunction(10,30));
- </script>
- </body>
- </html>
Output
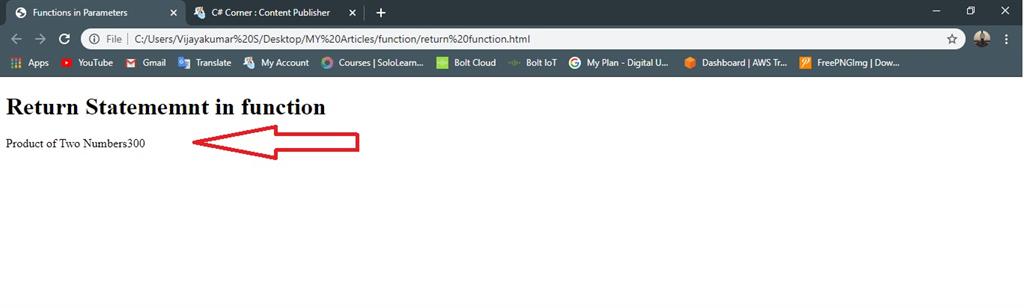
Summary
In this chapter, we learned about functions in JavaScript, declaration of the function, and how to use these functions in JavaScript example programs.
Author
Vijayakumar S
0
3.9k
2m