Project 2: Tic-Tac-Toe Game
Introduction
In this chapter, we will learn to implement a Tic-Tac-Toe game in Python.
Tic-Tac-Toe
The Tic-Tac-Toe board looks like the following:
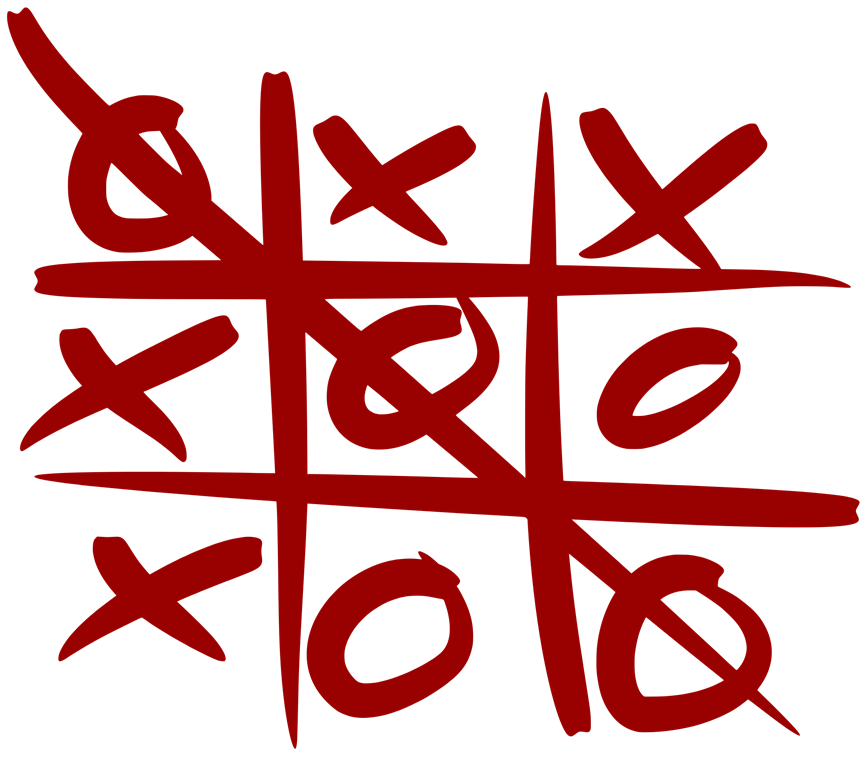
Game Rules
- Traditionally the first player plays with "X". So you can decide who wants to go with "X" and who wants to go with "O".
- Only one player can play at a time.
- If any of the players have filled a square then the other player and the same player cannot override that square.
- There are only two conditions that may match will be a draw or may win.
- The player that succeeds in placing three respective marks (X or O) in a horizontal, vertical, or diagonal row wins the game.
Winning condition
The code for the game is as follows,
- import os
- import time
- board = [' ',' ',' ',' ',' ',' ',' ',' ',' ',' ']
- player = 1
- ########win Flags##########
- Win = 1
- Draw = -1
- Running = 0
- Stop = 1
- ###########################
- Game = Running
- Mark = 'X'
- #This Function Draws Game Board
- def DrawBoard():
- print(" %c | %c | %c " % (board[1],board[2],board[3]))
- print("___|___|___")
- print(" %c | %c | %c " % (board[4],board[5],board[6]))
- print("___|___|___")
- print(" %c | %c | %c " % (board[7],board[8],board[9]))
- print(" | | ")
- #This Function Checks position is empty or not
- def CheckPosition(x):
- if(board[x] == ' '):
- return True
- else:
- return False
- #This Function Checks player has won or not
- def CheckWin():
- global Game
- #Horizontal winning condition
- if(board[1] == board[2] and board[2] == board[3] and board[1] != ' '):
- Game = Win
- elif(board[4] == board[5] and board[5] == board[6] and board[4] != ' '):
- Game = Win
- elif(board[7] == board[8] and board[8] == board[9] and board[7] != ' '):
- Game = Win
- #Vertical Winning Condition
- elif(board[1] == board[4] and board[4] == board[7] and board[1] != ' '):
- Game = Win
- elif(board[2] == board[5] and board[5] == board[8] and board[2] != ' '):
- Game = Win
- elif(board[3] == board[6] and board[6] == board[9] and board[3] != ' '):
- Game=Win
- #Diagonal Winning Condition
- elif(board[1] == board[5] and board[5] == board[9] and board[5] != ' '):
- Game = Win
- elif(board[3] == board[5] and board[5] == board[7] and board[5] != ' '):
- Game=Win
- #Match Tie or Draw Condition
- elif(board[1]!=' ' and board[2]!=' ' and board[3]!=' ' and board[4]!=' ' and board[5]!=' ' and board[6]!=' ' and board[7]!=' ' and board[8]!=' ' and board[9]!=' '):
- Game=Draw
- else:
- Game=Running
- print("Tic-Tac-Toe Game Designed By Sourabh Somani")
- print("Player 1 [X] --- Player 2 [O]\n")
- print()
- print()
- print("Please Wait...")
- time.sleep(3)
- while(Game == Running):
- os.system('cls')
- DrawBoard()
- if(player % 2 != 0):
- print("Player 1's chance")
- Mark = 'X'
- else:
- print("Player 2's chance")
- Mark = 'O'
- choice = int(input("Enter the position between [1-9] where you want to mark : "))
- if(CheckPosition(choice)):
- board[choice] = Mark
- player+=1
- CheckWin()
- os.system('cls')
- DrawBoard()
- if(Game==Draw):
- print("Game Draw")
- elif(Game==Win):
- player-=1
- if(player%2!=0):
- print("Player 1 Won")
- else:
- print("Player 2 Won")
Output
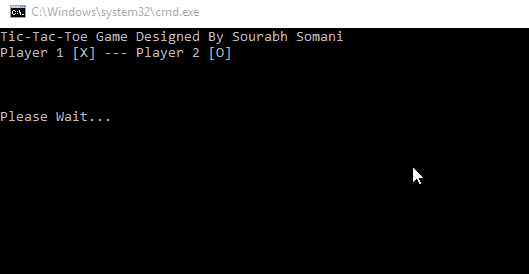
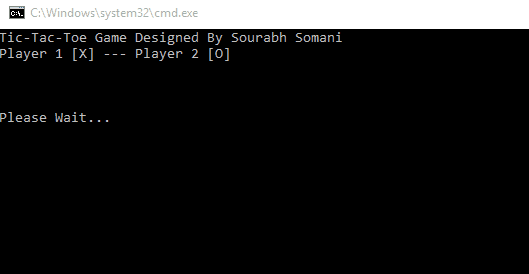
Conclusion
In the next chapter, we will learn how to make a music instrument using Python.
Author
Sourabh Somani
Tech Writter
47.6k
10.9m