Attributes of DataContract and DataMember
Introduction
We are explaining various concepts of WCF applications in this series. We have learned how to create a simple service, host it in a hosting environment, consume it, and much more about WCF applications. Here are the complete URL set for your reference:
In this chapter, we will see how to add attributes to a Data Contract and Data Member. In our previous chapter, we saw that a Data Contract is nothing but a simple C# class and the Data Member is the member of the class, it might be any data type.
Properties of Data Contract
According to MSDN, there are the following 4 properties of a Data Contract:
- IsReference: Gets or sets a value that indicates to preserve object reference data
- Name: Gets or sets the name of the data contract for the type
- Namespace: Gets or sets the namespace for the data contract for the type
- TypeId: When implemented in a derived class, gets a unique identifier for this Attribute.
Properties of Data Member
- EmitDefaultValue: If you want to add default values in the serialization then set the EmitDefaultValue to true, else set EmitDefaultValue to false. By default it is true.
- IsRequired: It controls the minOccurs attribute for the schema element. The default value is false.
- Name: It creates the schema element name generated for the member.
- Order: It maintains the order of each element in the schema. By default, it appears alphabetically.
But the most used property for Data Contract is the "name" property. We will see one example of it in a WCF application.
Create one WCF application and modify code for the Data Contract as in the following.
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Runtime.Serialization;
- using System.ServiceModel;
- using System.ServiceModel.Web;
- using System.Text;
- namespace DataContract
- {
- [ServiceContract]
- public interface IService1
- {
- [OperationContract(Name="AddTowPerson")]
- string AddName(Person obj);
- }
- //Create a data contract and set the property into it.
- [DataContract(Name="PersonClass")]
- public class Person
- {
- [DataMember(Name="FirstName",IsRequired=true,Order=0)]
- public string name;
- [DataMember(Name="LastName",IsRequired=true,Order=1)]
- public string surname;
- }
- }
We will now implement the interface in the concrete class. Try to understand the following code.
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Runtime.Serialization;
- using System.ServiceModel;
- using System.ServiceModel.Web;
- using System.Text;
- namespace DataContract
- {
- public class Service1 : IService1
- {
- public string AddName(Person obj)
- {
- return obj.name + obj.surname;
- }
- }
- }
We will use a console application to develop our client code. Here is sample code.
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using System.Diagnostics;
- using System.Collections;
- using Client;
- using System.ServiceModel;
- using System.ServiceModel.Description;
- using Client.ServiceReference1;
- namespace Client
- {
- class Program
- {
- static void Main(string[] args)
- {
- PersonClass p = new PersonClass();
- p.FirstName = "Sourav";
- p.LastName = "Kayal";
- Client.ServiceReference1.Service1Client obj = new Service1Client();
- String fullName = obj.AddTowPerson(p);
- Console.WriteLine(fullName);
- Console.ReadLine();
- }
- }
- }
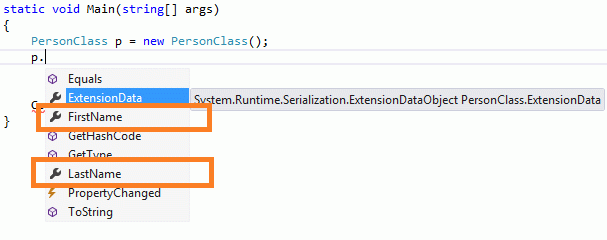
Again, Visual Studio IntelliSense is showing that there are two data members of the "PersonClass" class; they are "FirstName" and "LastName", but actually, we have given the identifier name like "name" and "surname", so here the name attribute is effective and suppressing the actual identifier name.
The following is sample output of the application:
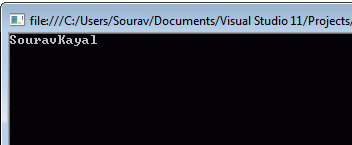
Conclusion
In this chapter, we saw the name property of the DataContract and DataMember attributes. Basically, this property suppresses the actual identifier name from the outside world. Hope you have enjoyed the chapter.
And this is the end of the learn series. Hope you learned all the necessary concepts to start working with WCF.