Components In React
Introduction
In the previous article, we went through the basics of creating React application. Now, moving towards what Components are, we will learn where and how components can be used in React.
Component
In React, a component is referred to as an isolated piece of code which can be reused in one or the other module. The React application contains a root component in which other subcomponents are included; for example - in a single page, the application is subdivided into 3 parts - Header, Main Content, and Footer. So, there is a single App Component having 3 subcomponents - Header Component, MainContent Component, and Footer Component.
There are 2 types of components in React.js
- Stateless Functional Component
- Stateful Class Component
Stateless Functional Component
This type of component includes simple JavaScript functions. These components include immutable properties, i.e., the value for properties cannot be changed. We need to use Hooks (will be discussed in the next article) to achieve functionality for making changes in properties using JS. A functional component is used mainly for UI.
Example
- function Demo(props) {
- return <h1> Hello, {props.Name} </h1>;
- }
Demo
Open App.js and remove additional HTML code except for the div. Now, add a new folder named Components and add a file named addition.js.
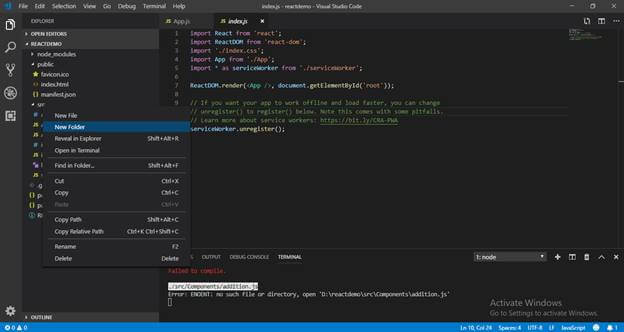
Create a new file named addition.js.
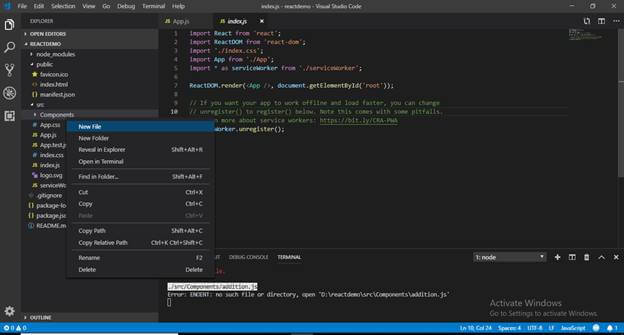
Add Stateless functional component code.
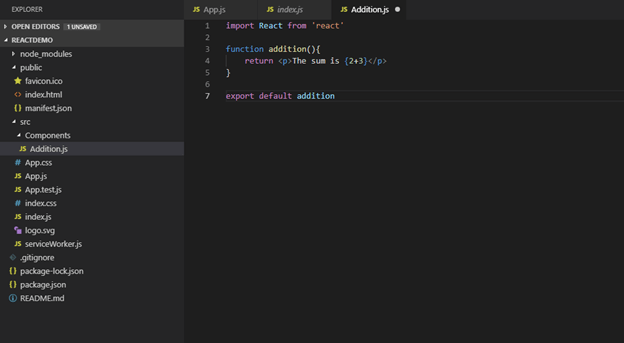
Please note in Addition.js, the last line exports default addition that is a must. Only through this line, it can be used in the parent component.
Now, again in App.js, import Addition from ‘./Components/Addition’. After importing, we can use the imported component as tag <Addition></Addition>.
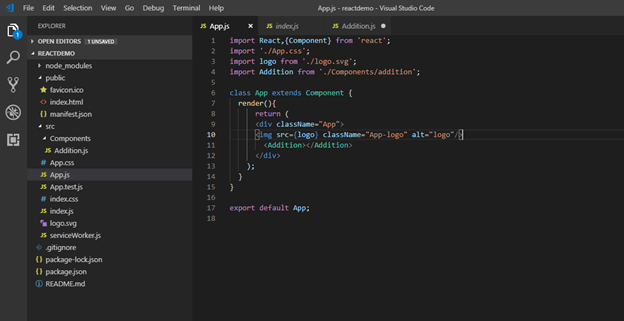
Now in terminal, run the command npm start.
This will run the React application and in the browser, the result will be displayed as below.
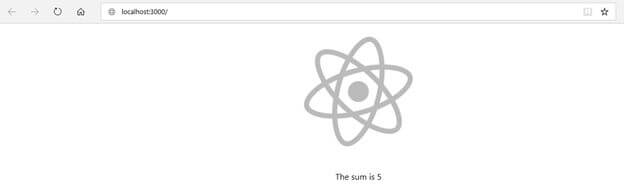
So, this way, we can call the functional component.
In another way, we can write addition function as arrow function like in the snap below.
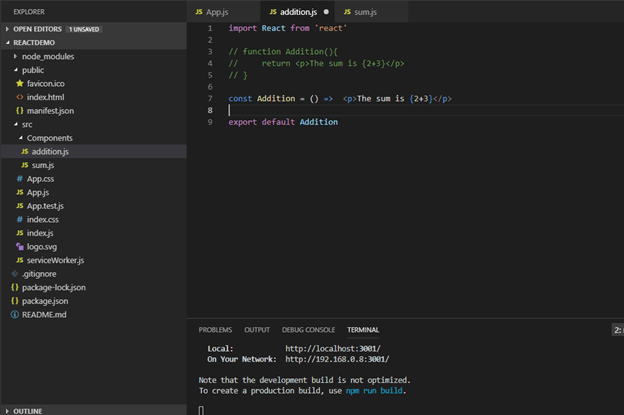
The output will remain the same,
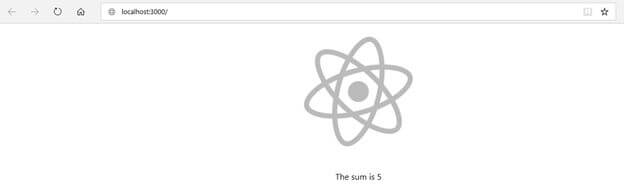
We can add as many tags for the additional component. It will be displayed in the browser.
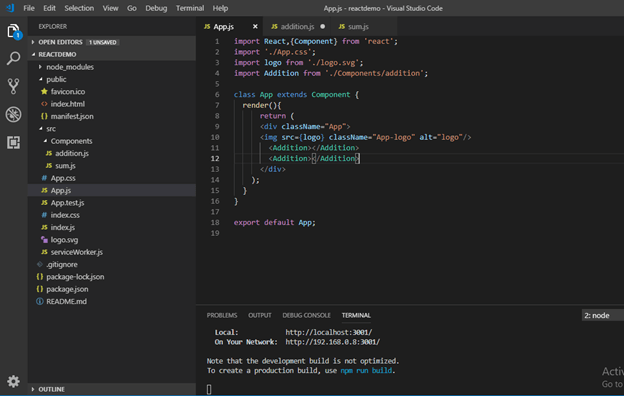
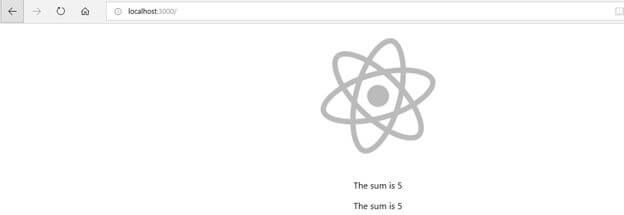
Stateful Class Component
The Class component is ES6 classes which extend the Component class from React library. The class component must include the render method which returns HTML.
- class Demo extends React.Component{
- render(){
- return <h1> Hello, {props.Name} </h1>;
- }
- }
Demo
For class component, create another JS file named sum.js in the component folder.
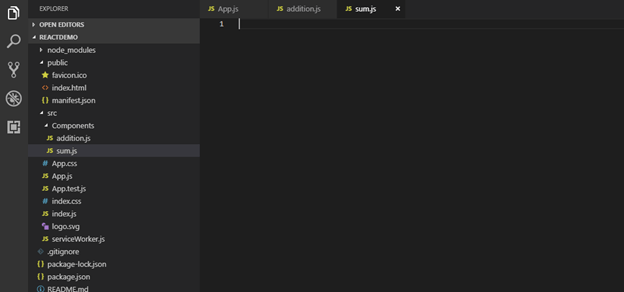
Now, import React and component from the React library. After that, declare the class extending component.
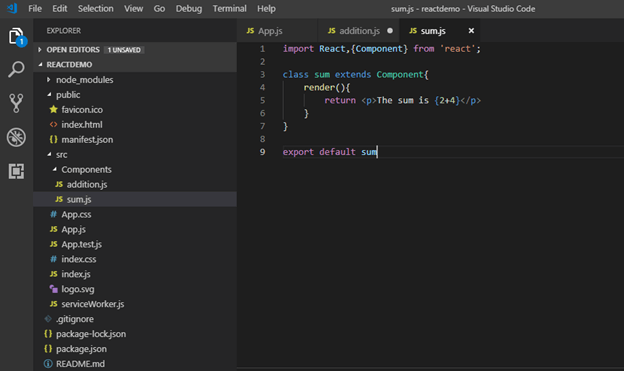
Now, import the exported sum class to App.js.
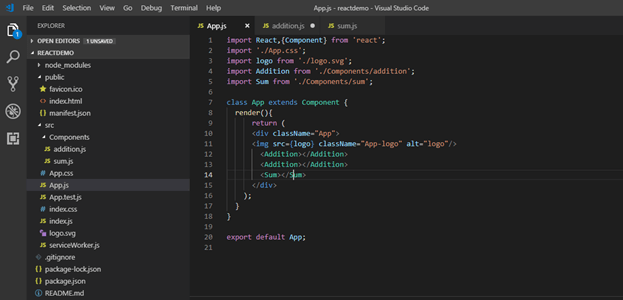
Save file it will automatically compile and result will be updated in the browser,
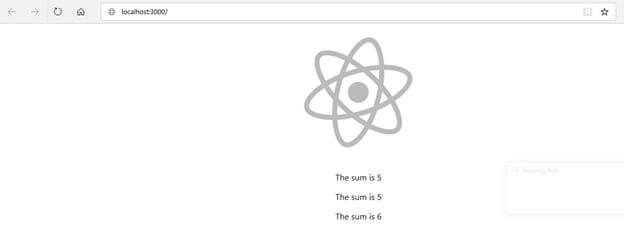
Important Point
- You can use it without exporting/importing class/functional component.
- The imported class/function must be in upper case; else it will give an error.
- Class/Functional component returning JSX i.e) Javascript XML.
Difference between Stateless Functional and Stateful Class Component
Stateless Functional Component | Stateful Class Component |
These are a simple javascript function | These classes extend the Component class from React library |
Stateless function uses Hooks to change properties value in View | Stateful class uses State and setState to change properties value |
Stateless doesn't use ‘this’ keyword | Stateful component provide complex UI data |
Summary
In this article, we have reviewed about components and their importance in React applications. Components are building a block of React js and can be reused and nested within other components. They play a very important role in React applications. React provides 2 types of components, Stateless Functional, and Stateless Class Component.
Next in this series >> Reactjs JSX, props, and Hooks
Author
Priyanka Jain
0
9.6k
907.4k