HTTP And React
Introduction
In the previous article, we learned about the concept of Render Props and Context in React. In this article, we will dive into some advanced concepts of React, like HTTP and usage of the GET and POST methods for data retrieval.
Basics of HTTP and React
So far, we have learned about the basics of React and how props and state are used. Now, we will learn how React makes API calls fetch data and display in the browser. As React is just a library for the user interface, it only knows about Props and State so here, HTTP comes into the picture.
To fetch data in React, there are many HTTP libraries available like - Apollo, Axios, Relay Modern, Request, and Superagent. In this article, we are going to use Axios library.
First of all, we will create a new React app using the following command.
- npx create-react-app http-react
The app is created now. Now, go to your newly created folder and install Axios to use it further in our application.
- npm install axios
After the command is executed successfully, we will see in the package.json file that the dependencies are updated.
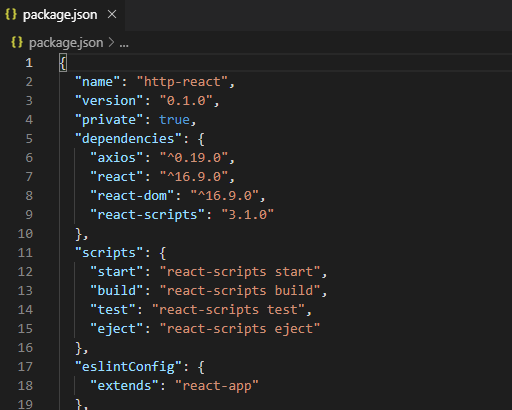
Now, we will see how we can fetch data and display in the browser.
HTTP GET
Here, we are using an online API to fetch data from it instead of creating our own API. We are using https://reqres.in/ to test our application.
Create a component named DisplayList.js.
- import React, { Component } from 'react'
- export class DisplayList extends Component {
- render() {
- return (
- <div>
- List of Users
- </div>
- )
- }
- }
- export default DisplayList
And include this component in App.js.
- import React from 'react';
- import logo from './logo.svg';
- import './App.css';
- import DisplayList from './components/DisplayList'
- function App() {
- return (
- <div className="App">
- <DisplayList></DisplayList>
- </div>
- );
- }
- export default App;
It will display the output as below.

Now, we will fetch data from library using Axios. For that, first, we need to import the axios library.
- import axios from 'axios'
Now, to store the data that we get from API, we will create an empty array in state.
- this.state = {
- Users:[]
- }
Now, we will call the API and store the data in our array in componentDidMount() lifecycle method. This is executed when the component mounts for the first time and it is called only once.
So, the component will go like below.
- import React, { Component } from 'react'
- import axios from 'axios'
- const error ={
- color: 'red',
- fontWeight:'bold',
- fontSize:'14'
- }
- export class DisplayList extends Component {
- constructor(props) {
- super(props)
- this.state = {
- users:[],
- errorMessage:""
- }
- }
- componentDidMount(){
- axios.get('https://reqres.in/api/users/')
- .then(response => {
- console.log(response.data.data)
- this.setState({
- users:response.data.data
- })
- })
- .catch(error =>{
- this.setState({
- errorMessage:"Error fetching data"
- })
- })
- }
- render() {
- const {users,errorMessage} = this.state
- console.log(users.length)
- return (
- <div>
- List of Users
- {
- users.length?
- users.map(user=> <div key={user.id}><img src={user.avatar} alt={user.first_name}/>{user.first_name + " "+ user.last_name}</div>):
- null
- }
- {errorMessage ? <div style={error}>{errorMessage}</div> : null}
- </div>
- )
- }
- }
- export default DisplayList
The output will be as below.
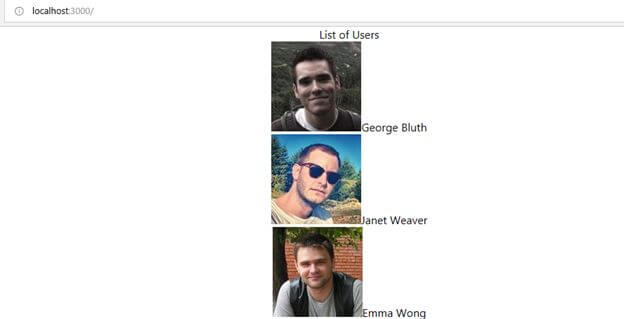
In the above, componentDidMount() will be called once the component is mounted, so it will display a list of users from provided API. If the API is incorrect or does not return output, then the error message will be displayed.

Next, we will be learning about HTTP Post.
HTTP POST
Like HTTP Get, we use Axios Post method to send the data to the API we use.
Let’s see a demo.
- import React, { Component } from 'react'
- import axios from 'axios'
- class UserForm extends Component {
- constructor(props) {
- super(props)
- this.state = {
- first_name: "",
- last_name: "",
- email: ""
- }
- }
- onChangeHandler = (e) => {
- this.setState({ [e.target.name]: e.target.value })
- }
- onSubmitHandler = (e) => {
- e.preventDefault();
- console.log(this.state)
- axios.post('https://reqres.in/api/users/',this.state)
- .then(response=>{
- console.log(response)
- })
- .catch(error => {
- console.log(error)
- })
- }
- render() {
- const { first_name, last_name, email } = this.state
- return (
- <div>
- <form onSubmit={this.onSubmitHandler}>
- <div>
- Email : <input type="text" name="email" value={email} onChange={this.onChangeHandler} />
- </div>
- <div>
- First Name : <input type="text" name="first_name" value={first_name} onChange={this.onChangeHandler} />
- </div>
- <div>
- Last Name : <input type="text" name="last_name" value={last_name} onChange={this.onChangeHandler} />
- </div>
- <button type="submit">Submit</button>
- </form>
- </div>
- )
- }
- }
- export default UserForm
Let us include UserForm component in App.js.
- import React from 'react';
- import './App.css';
- import UserForm from './components/UserForm'
- function App() {
- return (
- <div className="App">
- <UserForm></UserForm>
- </div>
- );
- }
- export default App;
The output will be displayed as below.
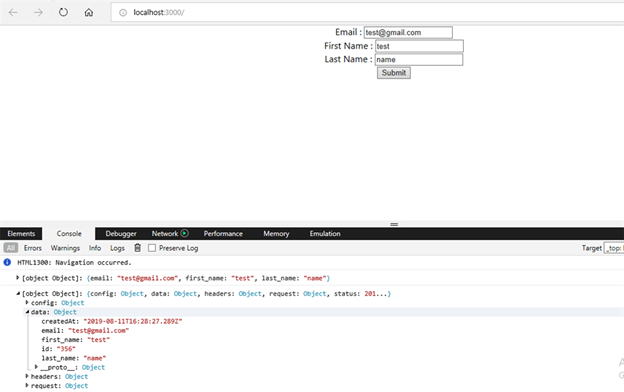
We can also use PUT, DELETE function in the same way.
FAQs
How can AJAX call be made in React?
There are many AJAX libraries available you can use with React. Some popular libraries are Axios, jQuery Ajax, and Window.fetch which is browser built-in.
Where AJAX calls should be made in Component lifecycle?
The calls should be made in componentDidMount() method as this method allows setState() to update your component after retrieval of data.
Summary
In this article, we have learned how React and Web server connect to each other to pass data and how HTTP Get and HTTP Post work.
You can download the source code attached. With this article, I've finished the basics of React. Now, I will move on to Advanced React which will have React Hooks and how hooks work in React. If you have any confusion or suggestions, please do comment.
Author
Priyanka Jain
0
9.6k
907.7k