Conditional Rendering And List Rendering In React
Introduction
In the previous article, we reviewed about binding event handler in React and using a method as property in React. In this article, we will learn about conditional rendering and how we can define conditions in React. Also, we will see how a list can be rendered in React.
Conditional Rendering
There are 5 ways we can define conditional rendering.
- If/Else
- Element Variables
- Ternary Operator
- Short-circuit operator
- Immediately - Invoked Function Expressions (IIFE).
If/Else Condition
The If/Else condition is the easiest way to specify conditional statements. The syntax is the same as in JavaScript despite the fact that for each if/else statement, a return statement needs to be defined which makes the code repetitive and not easy to read and modify.
For example, look at this code.
- import React from 'react';
- class ConditionalRendering extends React.Component{
- constructor(props){
- super(props);
- this.state ={
- IsLoggedIn : false
- }
- }
- render(){
- if(this.state.IsLoggedIn){
- return <div>Welcome User </div>
- }
- else{
- return <div>You need to login</div>
- }
- };
- }
- export default ConditionalRendering;
The output will be displayed as below,
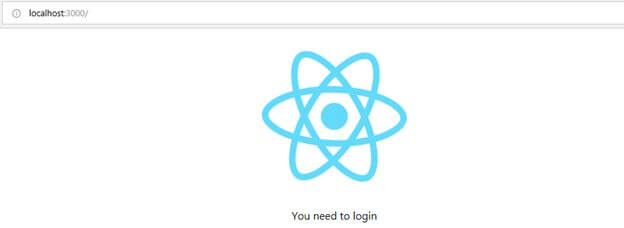
After changing the IsLogged : true, the output will be slightly different.
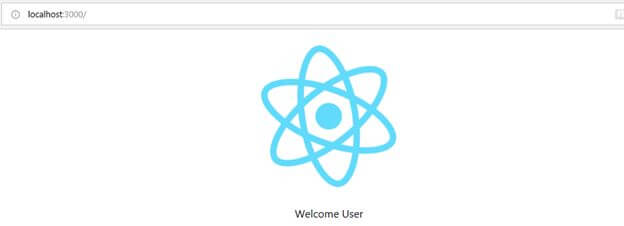
As seen in the above example, the return statement is defined every time for each if and else. This is just a demo example so it just includes 2 statements. However, the most common mistake is having multiple if/else statements and various return statements; which makes React to refresh the entire DOM when any condition is updated.
In short, React applications should not have multiple return statements in the render() method. So, to resolve this issue, either use embedded JSX expressions or variable assignment for achieving performance optimization.
As in the previous article, we have defined that React performs a search from top to bottom and after signifying changes in the component tree, updates only that part, so it is appropriate to use a proper conditional statement that helps React to evaluate properly. Now, look at the second approach.
Element Variables
The solution of repeating return statement in render() method is Element Variable. This approach uses a JSX variable that stores values when the condition returns true. And that variable is then used while displaying data.
For Example,
- import React from 'react';
- class ConditionalRendering extends React.Component{
- constructor(props){
- super(props);
- this.state ={
- IsLoggedIn : true
- }
- }
- render(){
- let message;
- if(this.state.IsLoggedIn){
- message = <div>Welcome User </div>;
- }
- else{
- message = <div>You need to login</div>;
- }
- return message;
- };
- }
- export default ConditionalRendering;
The output for the above code is as below.
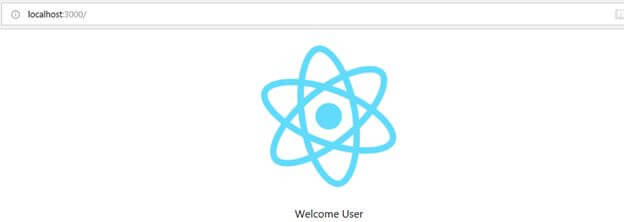
The main render() method has only 1 return statement but it is not necessary to use if-else blocks. We have another approach to simplifying this.
Ternary operator
Despite using if/else multiple block statements, we can use Ternary conditional operator. That includes:
- Condition? Expr_if_true : expr_if_false;
The operator is wrapped in curly braces and the expression can contain JSX, optionally wrapped in parentheses to improve readability and can be applied in different parts of the component.
For example,
- import React from 'react';
- class ConditionalRendering extends React.Component{
- constructor(props){
- super(props);
- this.state ={
- IsLoggedIn : true
- }
- }
- render(){
- return this.state.IsLoggedIn? <div> Welcome User</div> : "";
- };
- }
- export default ConditionalRendering;
The output for example will be displayed the same as above.
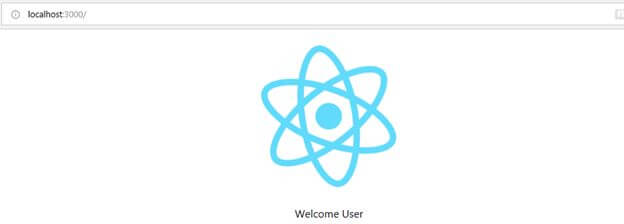
Short-circuit operator
This operator is the extended version of the short circuit operator. It is a special case and simplified version of the Ternary operator which uses && operator.
It is not like the & operator which evaluates the right side expression first. The && operator evaluates the left side expression and evaluates the result.
So, if the statement includes false && expr, it will not evaluate expr as the statement always returns false.
For example,
- import React from 'react';
- class ConditionalRendering extends React.Component{
- constructor(props){
- super(props);
- this.state ={
- IsLoggedIn : true
- }
- }
- render(){
- return this.state.IsLoggedIn && <div> Welcome User</div>;
- };
- }
- export default ConditionalRendering;
The output will be displayed as below.
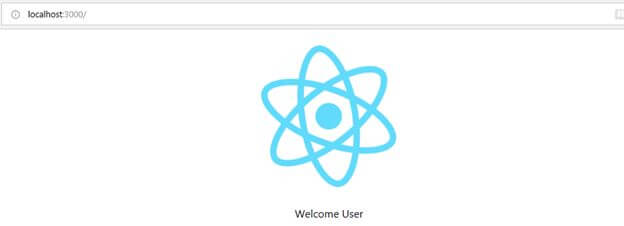
By changing the value of IsLoggedIn to false, the output will not display anything.
- this.state ={
- IsLoggedIn : false
- }
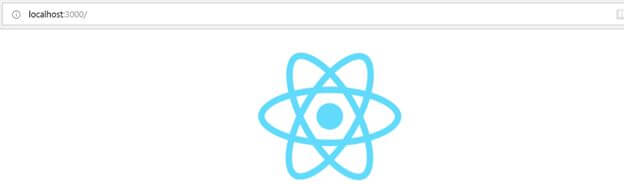
These techniques are used when we are not having multiple nested statements, for such purpose React provides another approach named Immediately-Invoked function expressions(IIFE).
Immediately-Invoked function expressions (IIFE)
As the name itself suggests, IIFE is the functions that are executed itself as soon as it is defined.
As in the below syntax it is defined and used.
- function TestFunc(){
- }
- TestFunc();
But if we need to execute function as soon as it's defined:
- (function TestFunc(/*args*/){
- }(/*args*/));
Or
- (function TestFunc(/*args*/){
- })(/*args*/);
Or we can use the arrow function:
- ((/*args */) => {
- })(/* args */)
In React, curly braces are used to use any of the above conditional statements and return based on user output.
List Rendering
In React, List array can have many ways to display in the browser, we will go for each type step by step. Let’s start with the first approach.
Using array index
List items can be displayed in the browser using the array index, but this approach becomes quite tedious as it includes code repetition and if the number of array is large then this is not a good approach.
For example:
- import React from 'react';
- function EmployeeList(){
- const employee = ['zbc','xyz'];
- return(
- <div>
- <h2>{employee[0]}</h2>
- <h2>{employee[1]}</h2>
- </div>
- )
- }
- export default EmployeeList;
The output will be displayed as below
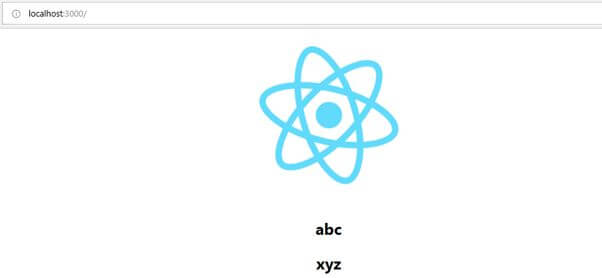
This approach uses code repetition so there is another approach named Array.map()
Array.map()
This function is used to display data from a list in an easy manner. This way provides easy readability and understandability.
Syntax - Array.map(name => {name})
For example,
- import React from 'react';
- function EmployeeList(){
- const employee = ['abc','xyz'];
- return(
- <div> {employee.map(emp => <h2>{emp}</h2>)} </div>
- )
- }
- export default EmployeeList;
The output will be same for the above example
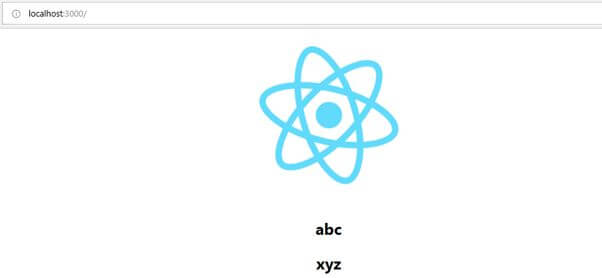
Now this can also be normalized by storing array list in the variable,
- import React from 'react';
- function EmployeeList(){
- const employee = ['abc','xyz'];
- const employeeList = employee.map(emp => <h2>{emp}</h2>);
- return(
- <div>{employeeList}</div>
- )
- }
- export default EmployeeList;
The output will be the same as above but the code provides better readability.
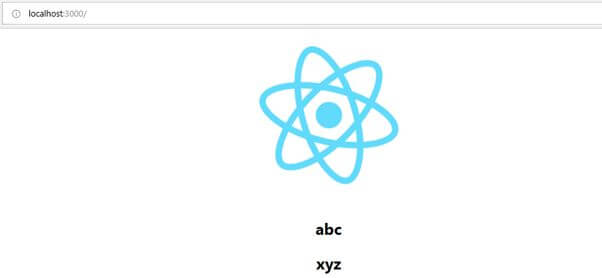
The above codes are simple concepts, having only 1 list item. Now we will see complex examples of having an array with key-value pair in each array having multiple values.
For example:
- import React from 'react';
- function EmployeeList(){
- const employee = [{
- name:'abc',
- salary:'50$',
- position:'Jr. Developer'
- },{
- name:'xyz',
- salary:'100$',
- position:'Sr. Developer'
- },{
- name:'mno',
- salary:'150$',
- position:'Project Manager'
- }
- ];
- const employeeList = employee.map(emp => <h2>My name is {emp.name} working as {emp.position} and having salary {emp.salary} </h2>);
- return(
- <div>
- {employeeList}
- </div>
- )
- }
- export default EmployeeList;
The output will be displayed as,
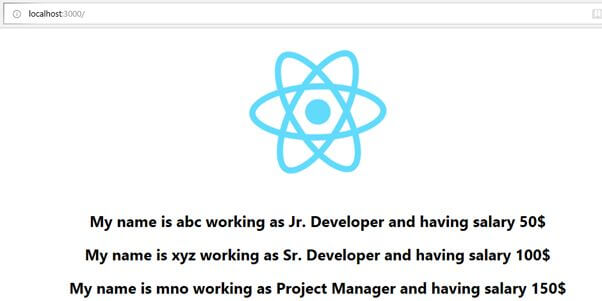
Now let's talk about moving the array concept to subcomponent and calling that component in main component.
Create a subcomponent having function that will return html:
- import React from 'react';
- function Employees({emp}){
- return(
- <div>
- <h2>My name is {emp.name} working as {emp.position} and having salary {emp.salary} </h2>
- </div>
- )
- }
- export default Employees;
Now import this component in parent component
- import React from 'react';
- import Employees from './Employees';
- function EmployeeList(){
- const employee = [{
- name:'abc',
- salary:'50$',
- position:'Jr. Developer'
- },{
- name:'xyz',
- salary:'100$',
- position:'Sr. Developer'
- },{
- name:'mno',
- salary:'150$',
- position:'Project Manager'
- }
- ];
- const employeeList = employee.map(emp =>
- <Employees emp={emp}></Employees>
- );
- return <div>{employeeList}</div>;
- }
- export default EmployeeList;
The output for the above will be displayed as below
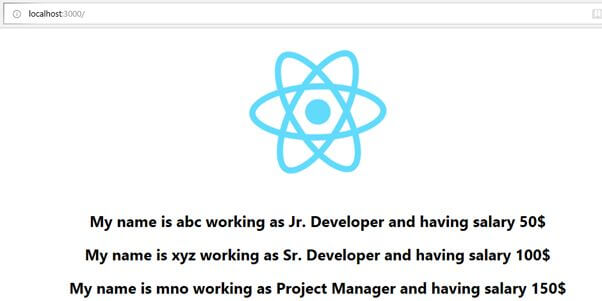
Now look at the console in browser where there are errors related to keys:
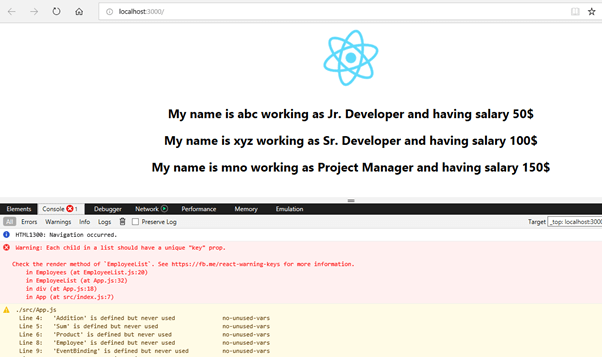
It will show aa warning related to keys as each child in a list should have a unique key prop. This error can be resolved by defining the key to each list item generated using JSX. The key defined should not be the same for any list item.
Now as in the previous example add key property to EmployeeList component,
- import React from 'react';
- import Employees from './Employees';
- function EmployeeList(){
- const employee = [{
- id:1,
- name:'abc',
- salary:'50$',
- position:'Jr. Developer'
- },{
- id:2,
- name:'xyz',
- salary:'100$',
- position:'Sr. Developer'
- },{
- id:3,
- name:'mno',
- salary:'150$',
- position:'Project Manager'
- }
- ];
- const employeeList = employee.map(emp => <Employees key= {emp.id} emp={emp}></Employees>);
- return <div>{employeeList}</div>;
- }
- export default EmployeeList;
Now, check the result in the browser.
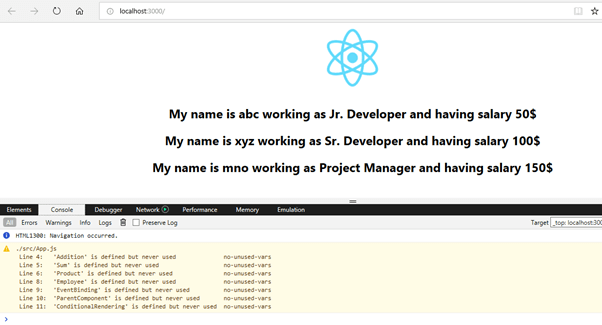
Now we can see there is no warning in the console. The key prop can contain anything from the list that we know is unique.
The important point that needs to be kept in mind when using key prop is that key prop cannot be used in child component, for example, if we access key prop from EmployeeList component in Employee Component,
- import React from 'react';
- function Employees({emp,key}){
- return(
- <div>
- <h2>{key} My name is {emp.name} working as {emp.position} and having salary {emp.salary} </h2>
- </div>
- )
- }
- export default Employees;
The output will be displayed as below,
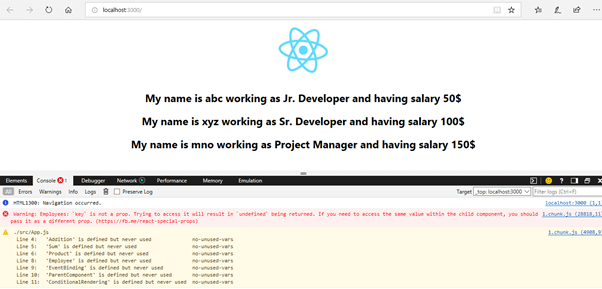
The warning in console itself suggests that key is undefined when passed from parent component to child component.
Now here the important point is why do we need key props. The key props are used to identify item lists on that key and to append, remove or insert list item from the lists.
Importance of key prop,
-
Key prop helps to easily identify which item is added, updated or removed.
-
Key prop helps in updating user interface efficiently.
-
Keys provide stable identity to elements..
Now let’s move further and see what if we have a simple array which doesn’t have any attribute name, id for example ,
- const cities = ['surat','vadodara','mumbai'];
- const cityList = cities.map(city => <h2 key={city}>{city}</h2>);
- return <div>{cityList}</div>;
The output will be displayed as below
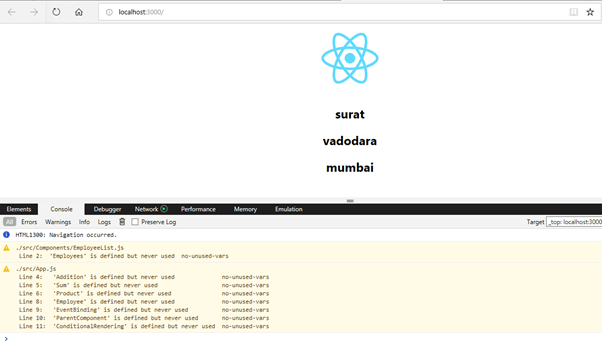
Now, the key is defined based on the values of the list. Now what if the values in the array have repeating values, then it will throw a warning for keys to be unique.
Updated list:
- const cities = ['surat','vadodara','mumbai','surat'];
The output will be shown as below
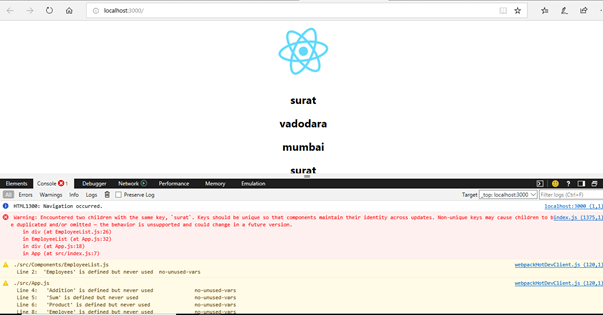
So in that case we should use index as key for array values. The map function accept 2 parameters , map(value,index).
For example,
- const cities = ['surat','vadodara','mumbai','surat'];
- const cityList = cities.map((city,index) => <h2 key={index}>{city}</h2>);
- return <div>{cityList}</div>;
Now the output will be displayed as below without warning,
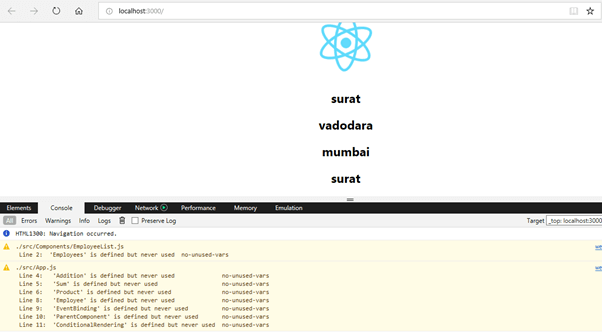
In React, index starts from 0 as shown below,
- const cities = ['surat','vadodara','mumbai','surat'];
- const cityList = cities.map((city,index) => <h2 key={index}>{index} {city}</h2>);
- return <div>{cityList}</div>;
After defining index as key the ouput will be displayed as below,
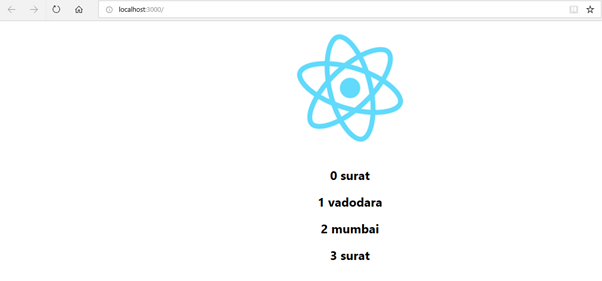
When should index be used as a key?
It is not valid to always use index as a key as it may result in UI issues. So following are the points where index should be used as key:
-
The items in the list don’t have a unique id.
-
The list is static and that is not going to change.
-
The list is not going to filter or sort.
When all the above 3 conditions are fulfilled by the list then the index can be used as a key in the list.
Conclusion
In this article, we learned Conditional Rendering and List rendering in React applications, and in how many ways we can use Array.Map() to display list data. In the next article, we will learn about Styling and CSS basics usage in React and get an introduction to the Form concept.
Next in this series >> Styling And CSS in React
Author
Priyanka Jain
0
9.6k
906.6k