Styling And CSS In React
Introduction
In the previous article, we learned about condition rendering and list rendering in React and saw in how many ways, we can use conditional rendering. We made the use of Array.Map() in list rendering. Now, in this article, we will learn about Styles and CSS basics in React and how styled components work in React.
Styling in React
There are 4 ways styling can be done in React.
-
CSS Stylesheets
-
Inline style
-
CSS Modules.
-
CSS in JS libraries.
To specify the CSS class, we can use the className attribute. This will apply to all DOM and SVG elements.
CSS Stylesheets
This styling involves importing external CSS file in our component. This is used in the same way as we use in our HTML form. Using this approach, we can create individual file for each component.
For example,
I have created a file named custom.css having the below code
- .error{
- color:red
- }
- .info{
- color: blue
- }
Now, import the css file in your component file
- import React from 'react';
- import '../css/custom.css';
- const Messages = () =>(
- <div>
- <p className="error">This is Error</p>
- <p className="info">This is information</p>
- </div>
- )
- export default Messages;
This will display an output.
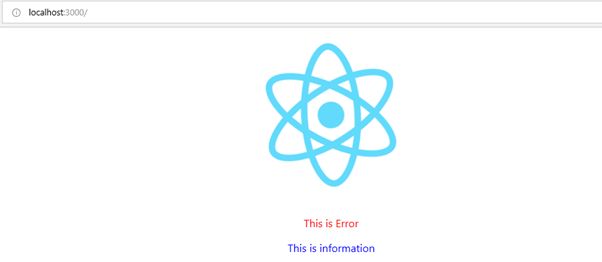
Inline style
This styling is not a string. It contains a key-value pair in camel case format having style name and its value. The value is usually a string.
In this, we can use style={nameofvariable} as to pass variable name created or directly style is passed style={{color:’red’}}
For example,
In our component, we will define an object containing style key-value pair
- import React from 'react';
- const error ={
- color: 'red',
- fontWeight:'bold',
- fontSize:'14'
- }
- const info ={
- color: 'blue',
- fontWeight:'italics',
- fontSize:'16'
- }
- const Messages = () =>(
- <div>
- <p style={error}>This is Error</p>
- <p style={info}>This is information</p>
- </div>
- )
- export default Messages;
The output will be displayed as below.
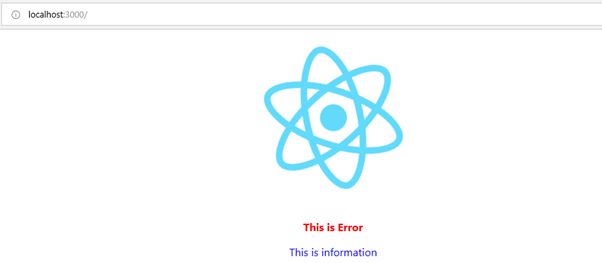
CSS Modules
In a class module, every class name and animation are scoped locally to the component that is importing it. In this way, a naming convention can be used based on requirements without worrying about global conflicts as React uses component and every component is separate until imported.
For example,
custom.css
- .error {
- color:red;
- font-weight: bold;
- font-Size :14
- }
- .info{
- color: blue;
- font-weight:'italics';
- font-size:'16';
- }
And in style component,
- import React from 'react';
- import styles from '../css/custom.css';
- class Messages extends React.Component{
- render(){
- return(
- <div>
- <p className={styles.error}>This is Error</p>
- </div>
- )
- }
- }
- export default Messages;
This will display an output as below.
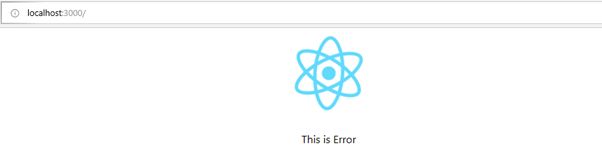
As in the above snapshot, we can see that CSS is not applied, the reason is for using CSS Modules concept in React the naming convention for CSS files should be <ModuleName>.module.css
Now change the file name as Messages.module.css and update its import name in Messages.js file. Now the output will be displayed as below:
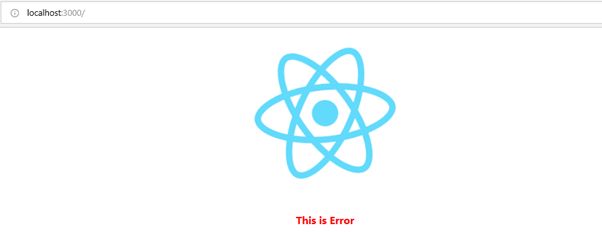
CSS Modules allows class composition as one class can be inherited in other class. It is also possible to extend multiple classes at the same time. This feature allows using existing classes with a new class.
Css-In-Js (styled component)
Styled components are Css-In-Js library that includes the utilization of template literals to style your desired component. This technique is used for writing CSS code to customize your component and it will also not conflict with other elements or components.
Benefits of Styled Component
Automatic critical CSS -The styles component keeps track of components rendered on the page and add their styles and will do nothing else, that results in making it fully automatic.
Conditional Rendering -The styled component has the functionality to implement styles based on values of props which is not provided by any other CSS methods like SASS, LESS, etc.
Easy Maintenance -The styled components provide quick changes as we need not surf all the CSS files in search of CSS or if it may affect other components as it is implemented component-wise.
No Confliction of classes - There is no conflict of the class name in the styled component as each style is bound to its component and generates a unique class name.
For using Styled Component, we need to execute below 2 steps
First, to use styled component first install styled-components through npm manager using the command given below.
- npm i styled-components
Now, the second thing. We need to import our styled component in our component.
- import styled, { css } from 'styled-components';
After completing the above 2 steps, we can continue using style components.
Let’s see a demo for Styled Components
Created one component file named UserForm.js
- import React,{Component} from 'react';
- import styled from 'styled-components';
- const Textarea = styled.textarea`
- padding: 10px 5px;
- background-color:black;
- color:white;
- `;
- const Button = styled.button`
- padding: 5px;
- color : black;
- background-color: gray
- `
- const Label = styled.label`
- color : black;
- background-color: yellow
- `
- class UserForm extends Component{
- render(){
- return(
- <div>
- <Label>Enter Name</Label>
- <Textarea type="text"></Textarea>
- <Button type="submit">Submit</Button>
- </div>
- )
- }
- }
- export default UserForm;
Now import that form in App.js file
- import React from 'react';
- import logo from './logo.svg';
- import './App.css';
- import UserForm from "./components/UserForm";
- function App() {
- return (
- <div className="App">
- <header>
- <img src={logo} className="App-logo" alt="logo" />
- </header>
- <UserForm></UserForm>
- </div>
- );
- }
- export default App;
This will display output as below,
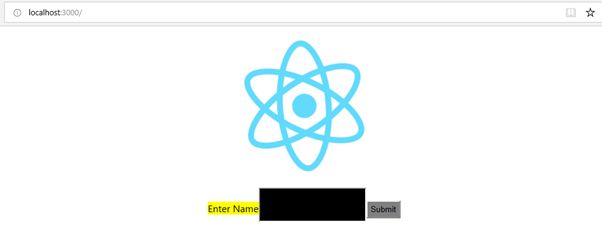
FAQ related to Styling and CSS in React,
How CSS classes can be added in the component in React?
In React, we can pass prop as className to the element to define CSS class in camelCase.
- <p className=”error”>Error</p>
Can inline styles be used in a React component? Which technique is better to use in component?
Yes, Inline styles can be used in React. But using inline styles is not preferable to React. Using CSS class is better in terms of performance rather than inline styles.
Which browsers support styled components?
Styled Components are supported by,
- V2.x (React v0.14+): IE9, Chrome, Edge.
- V3.x (React v0.14+): IE9+,Chrome, Edge.
- V4.x (React v16.3+): IE11, IE9, Chrome, Edge.
Chrome and edge are referred to as Evergreen browsers as they can be updated without depending on the operating system on which it is needed to install.
Why should styled components not be declared in render() method?
While declaring styled component in render() method of the component, React will recompile that part of DOM subtree on each render instead of just observing the changes of the previous node. This will result in performance and behavioral issues. So, it is preferred to use a styled component above your component definition.
Example:
The below code should not be used as each time component is rendered without considering only changes.
- const User = () => {
- const Button = `
- color: black;
- background-color: white;
- `
- return(
- <div>
- <button>Click Me</Button>
- </div>
- )
- }
We can use the below code which will improve performance and will only check for changes that have been done.
- const Button = `
- color: black;
- background-color: white;
- `
- const User = () => {
- return(
- <div>
- <button>Click Me</Button>
- </div>
- )
- }
Basics of Form in React
HTML form elements work a little bit differently than they do in React. This is because the form element has some internal state. This form behavior has the default HTML behavior of submitting to a new page when the user submits the form. This same concept will also work in React but sometimes it will fail so it is preferable to use JavaScript function to access submitted data. This can be achieved using a technique called “Controlled Components”.
Conclusion
In this article, we reviewed some basics Styles and CSS and how it can be used in React and some basic implementation of Styled Components and CSS Modules. In the next article, we are going to learn about the details of Form and how it can be used in React and Component of Lifecycle methods.
Next in this series > Forms in React
Author
Priyanka Jain
0
9.6k
906.6k