State In React
Introduction
In the previous article, we learned about JSX, hooks, and props. In this article, we are going to see what State is, what are its uses, and how State is different from props in React.
State in React
A State in React can be changed as per the user action or any other actions. When the state is changed, React re-renders the component automatically to the browser. We can say that State contains the information that can be changed during the component’s lifecycle. React stores the state of a component in this.state. The value of state can be set in 2 ways depending on the way a component is created.
Below are the two ways by which the value for a state can be set or initialized.
- // Using React.createClass
- var Counter = React.createClass({
- getInitialState: function(){
- return {counter: 0};
- }
- });
or
- // Using ES6 classes
- class Counter extends React.Component{
- constructor(props){
- super(props);
- this.state = {counter: 0};
- }
- }
The component state can be changed calling the following code.
- this.setState(data,callback);
In this.setState() method, it performs a shallow merge of data which then re-renders the component. The data argument under setState() method contains object or function that contains keys to be updated.The optional callback parameter under setState method contain function.
setState() is asynchronous,it do not immediately update values if user tries to access this.state immediately after setState(), there are chances to get old values.This method schedules an update, computation is delayed until necessary.
In State, 3 things need to be taken care of,
- State should not be assigned a value directly other than in constructor, always use setState() method to assign value.
- State updates may be asynchronous, so the user should not rely on state value for calculating next state value.
- State updates are shallow merge, In setState() multiple variables may be present so it merged values even though access or updated separately.
State is also stated as local or encapsulated, as it can only be accessed in a function or class which owns it, irrespective of whether it is in stateless or stateful components.
Reconciliation process
React has a process named Reconciliation, this process includes how React updates the DOM after making changes to a component when there is a change in state. When setState() method is triggered, React creates a new tree containing the React element in the component along with updated State. This tree is used to find out how component’s UI should change in response to state change by comparing its element with the previous tree. As React knows which changes need to be done that’s why react will only update that part of DOM making React performing fast.
Demo
In React, here is a demo that uses State. In this demo, we create a component named StateDemo.js under component folder.
Now, let us add the code as below.
- import React from 'react';
- class States extends React.Component {
- constructor(props) {
- super(props);
- this.state = {count: 0};
- this.onClick = this.onClick.bind(this);
- }
- render() {
- return (
- <div onClick={this.onClick}>{this.state.count}</div>
- );
- }
- onClick() {
- this.setState({count: this.state.count + 1});
- console.log(this.state.count);
- }
- }
- export default States;
Now, in app.js, import your newly created component and define its tag in App class.
- import React,{Component} from 'react';
- import './App.css';
- import logo from './logo.svg';
- import States from './Components/StateDemo';
- class App extends Component {
- render(){
- return (
- <div className="App">
- <img src={logo} className="App-logo" alt="logo"/>
- <States/>
- </div>
- );
- }
- }
- export default App;
Now, run your application. This will display your output in the browser.
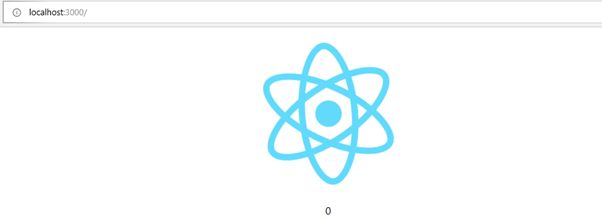
Now, on clicking on div where 0 is displayed, it will increase the counter based on clicks.
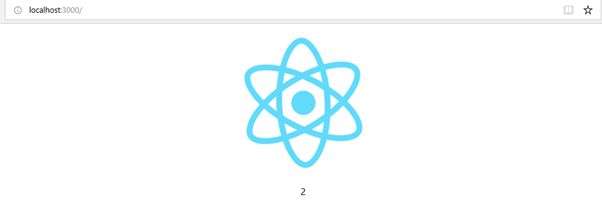
Now, let's introduce a button. On click of this, the counter will get updated.
- import React from 'react';
- class States extends React.Component {
- constructor(props) {
- super(props);
- this.state = {count: 0};
- this.onClick = this.onClick.bind(this);
- }
- render() {
- return (
- <div>
- <h1>{this.state.count}</h1>
- <button onClick={this.onClick}>Counter</button>
- </div>
- );
- }
- onClick() {
- this.setState({count: this.state.count + 1});
- console.log(this.state.count);
- }
- }
- export default States;
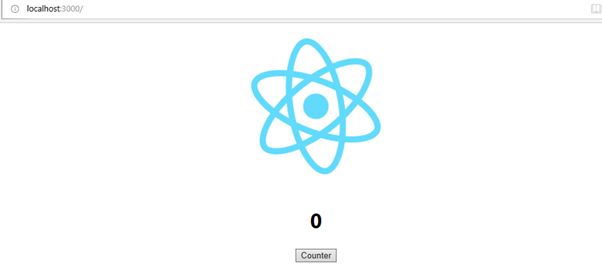
Difference between props and State
props | State |
Props make components reusable by giving components the ability to receive data from a parent component | State referred to the local state of a component which neither be accessed nor modified outside component. It can only be used and accessed within a component. |
Props are passed to the component | State is managed within the component |
Props are used in function parameter | State is used as a variable declared in the function body |
Props are immutable i.e) their values cannot be changed once assigned | State are mutable i.e) their values can be changed within a component |
In functional components, props is used While in class component, this.props is used. | In functional components, useState() is used While in class component, this.state is used. |
Props provide better performance | State gives a performance way worse than that of props |
Props are used to pass data to child components | State cannot be used to access from a child component, rather than it uses props |
Points to remember:
- Always use setState() method to change the value of State, never assign a value directly.
- For code that needs to be executed after state modified, always implement that code in a callback function which is the second parameter in setState() method.
- When required to update state on previous state value, pass the state as a function value instead of a regular object.
Some FAQ's related to State
What is the difference between State and Props?
As already stated previously, props are properties that is passed to component in the same as functional parameter and cannot be changed while state is managed within component and can be changed and updated.
What is the use of setState() ?
setState() method is used to schedule the update in the component. This component re-render when any changes are made in state.
Why setState() returns incorrect value ?
The call to setState() are asynchronous. Any code should not depend on this.State value just after setState() method is called. It it do not update value instantly. So in spite of using object in setState() , updater function should be used if values need to be computed.
How do the values are updated that depend on current value?
In setState() method, function should be used instead of object to ensure that call will always use the current state value.
What does the second argument in setState() used for?
The setState() function’s second argument is a callback function. This callback function is invoked when setState() has finished its execution and the component is re-rendered. This function contains the statements that need to be executed just after state updated.
Conclusion
In this article, we reviewed what is State and its usage, and how it works in React. We also reviewed the difference between props and State. Both have their pros and cons and can be used according to their requirement in our application. In the next article, we are going to learn about destructuring of props and state and also what is event handling in React and how it works.
Next in this series >> Destructing of props and state in React
Author
Priyanka Jain
0
9.6k
905.2k