Destructuring And Event Handler In React
Introduction
In the previous article, we learned about state and its usage, and the difference between props and state. Now, in this article, we will go ahead and review a small concept of destructuring props and states and the basics of event handling in React.js.
Destructuring
Destructuring is a simple concept introduced in React ES6. It is a JavaScript feature that allows the users to extract multiple pieces of data from an array or object and assign them to their own variable. This concept allows us to easily extract data out of an array or object, and makes your code readable and usable.
For example, you have an employee with multiple properties.
- const employee = {
- name : "emp",
- salary:"10k",
- designation:"tester"
- }
Before ES6, you need to access properties as below.
- console.log(employee.name);
- console.log(employee.salary);
- console.log(employee.designation);
Destructuring let us distribute this code.
- const {name,salary,designation} = employee
The above code is same as the code shown below.
- const name = employee.name;
- const salary = employee.salary;
- const designation = employee.designation;
Now, the above properties can be accessed without using the employee. prefix.
- console.log(name);
- console.log(salary);
- console.log(designation);
Destructuring props and states
Now, look at the example below. I want to pass by properties in the below-mentioned way.
- const personalinfo =
- {
- name: "xyz",
- address: "150 seattle",
- zipcode: "111111",
- city: "wc",
- contactnumber: "999999999"
- }
- const proffessionalinfo =
- {
- occupation : "business",
- designation : "CEO",
- salary : "50k"
- }
Now, add the above properties in Employee component in App.js.
- import React,{Component} from "react";
- import "./App.css";
- import logo from "./logo.svg";
- import Employee from "./Components/Employee";
- class App extends Component {
- render(){
- const personalinfo =
- {
- name: "xyz",
- address: "150 seattle",
- zipcode: "111111",
- city: "wc",
- contactnumber: "999999999"
- }
- const proffessionalinfo =
- {
- occupation : "business",
- designation : "CEO",
- salary : "50k"
- }
- return (
- <div className="App">
- <img src={logo} className="App-logo" alt="logo"/>
- <Employee personalinfo={personalinfo} proffessionalinfo={proffessionalinfo} >
- </Employee>
- </div>
- );
- }
- }
- export default App;
Access the above properties in Employee component, as below.
- import React from 'react';
- const Employee = (props) => {
- return(
- <div>
- <legend>Personal Details</legend><br/>
- Name : {props.personalinfo.name}<br/>
- address : {props.personalinfo.address}<br/>
- zipcode : {props.personalinfo.zipcode}<br/>
- city : {props.personalinfo.city}<br/>
- contactnumber : {props.personalinfo.contactnumber}<br/>
- <legend>Proffessional Detail</legend><br/>
- occupation : {props.proffessionalinfo.occupation}<br/>
- designation : {props.proffessionalinfo.designation}<br/>
- salary : {props.proffessionalinfo.salary}<br/>
- </div>
- )
- }
- export default Employee;
The output will be displayed as below.
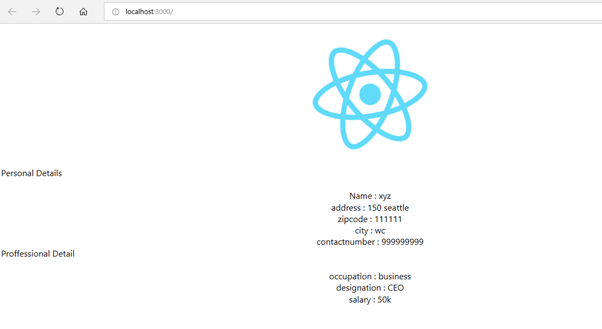
In the above code, we need to specify props.personalinfo or props.proffessionalinfo for every property.
Now, if we destructure props, we made the below changes in Employee component.
- import React from 'react';
- const Employee = ({personalinfo,proffessionalinfo}) => {
- return(
- <div>
- <legend>Personal Details</legend><br/>
- Name : {personalinfo.name}<br/>
- address : {personalinfo.address}<br/>
- zipcode : {personalinfo.zipcode}<br/>
- city : {personalinfo.city}<br/>
- contactnumber : {personalinfo.contactnumber}<br/>
- <legend>Proffessional Detail</legend><br/>
- occupation : {proffessionalinfo.occupation}<br/>
- designation : {proffessionalinfo.designation}<br/>
- salary : {proffessionalinfo.salary}<br/>
- </div>
- )
- }
- export default Employee;
The important changes are done in the first line. Instead of props, we have passed personalinfo and proffessionalinfo object.
Now, we will be reviewing the output.
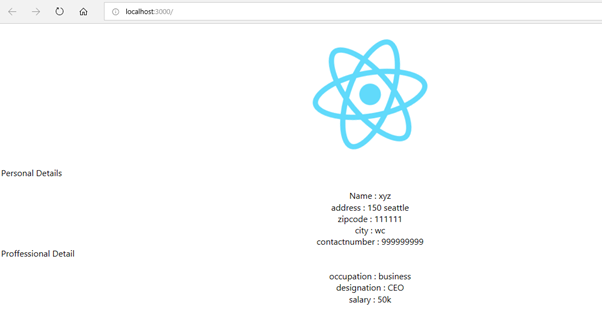
The output remains the same but now the code is more readable and clean.
So, this is a way to destructure props and in the same way, we can destructure the state.
Event Handling
Event handling is the same method we use in JavaSript. It is a handler that determines what actions need to be taken when an event is fired. Here, an event refers to a mouse click, button click, mouse hover, text input, and so on.
In React, all event handlers are defined in camel case, i.e., in JavaScript onclick will be defined as onClick() in React application.
Unlike in HTML, in JSX, we pass a function as an event handler rather than a string.
For example, in HTML, we used to define the function on button click as below.
- <button onclick =”showName()”> Display Name
- </button>
While in React, we define this as:
- <button onclick ={showName}> Display Name
- </button>
The other difference between is the value returned by function.
In HTML normally we write,
- <a href=”#” onclick=”console.log(‘Link Clicked’); return false;”>Click me</a>
While in React, False cannot be returned. We need to compulsorily add it to prevent the default behavior. You need to call preventDefault explicitly. For example -
- function DisplayLink(){
- function linkClicked(e){
- e.preventDefault(e);
- console.log(“Link Clicked”);
- }
- return(
- <a href=”#” onClick={linkClicked}> Click me </a>
- );
- }
Here, e refers to Synthetic event. React implements its own events to provide cross-browser compatibility support. Basically, React defines the browser native event into an instance of React SyntheticEvent and passed it to the React handlers.
Synthetic Events
Synthetic event is the concept introduced by React to provide cross-browser functionality. It has the same interface as the browser’s native event which includes stopPropagation() and preventDefault() event. This concept is pooled which means that SyntheticEvent object will be reused and all properties inside this event became null after event callback due to performance reasons, as this event cannot be used in an asynchronous way.
If required to use SyntheticEvent in an asynchronous way, the event.persist() method is used, that will remove SyntheticEvent() from the pool and allow references to the event to be retained by user code.
Conclusion
In this article, we learn about destructuring of props and state in React and also about some basic concepts of event handling in React and how it is different from basic HTML. In the next article, we will learn in detail about event handling and rendering the props techniques in React.
Next in this series >> Binding Event Handler and Method as Props
Author
Priyanka Jain
0
9.6k
905.2k