Google Firebase Email/Password and Google Login
Introduction
Google Firebase is trending nowadays. It has around 10 sign in methods which include email, Google, Facebook, phone, Twitter, Yahoo etc. We are covering only the email and Google login options in this article. Now, we are going step by step. I have given the git repository link at the bottom as well.
Steps
-
The first and most basic step is to create a new application in Flutter. If you are a beginner in Flutter, then you can check my blog Create a first app in Flutter. I have created an app named “flutter_email_signin”.
-
Now, you need to set up a project in Google Firebase. Follow the below steps for that. Please follow the steps very carefully.
-
Go here and add a new project. I will share a screenshot of how it looks so you will get a better idea.
-
Click on "Add Project" to add a new project in Google Firebase. Then, you will find the below form.
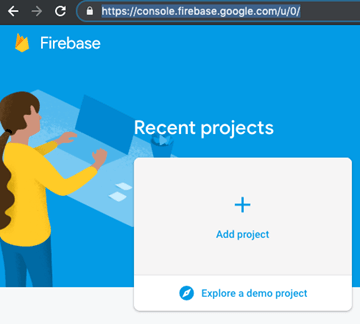
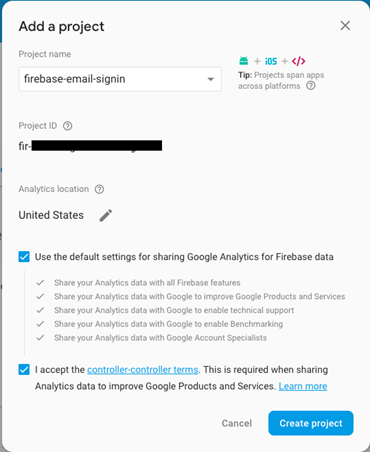
-
Give a project name and accept the terms and conditions and click on Create Project. It will take some time to create a new project and redirect you to the project overview page.
-
Now, you need to add an Android app in this project [Please check the below screenshot]. You can add a new Android project by clicking on the Android icon. You can also add an iOS project if you want to create the iOS application for the same.
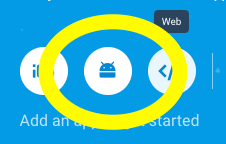
-
In Project Overview, add Android app. For that, click on the Android icon. It will open a new form.
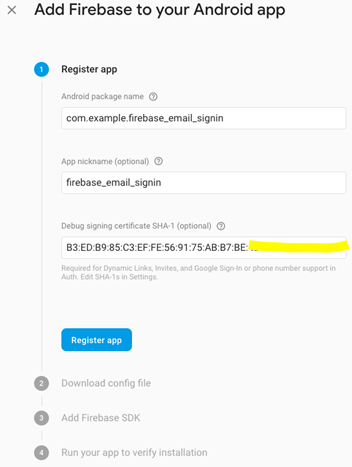
-
You will find the Android package name in the AndroidManifest.xml file in the Android => App => main folder of your project.
-
App nickname is optional
-
For SHA-1 generation goto here.
-
In step 2 download google-service.json and put in Android => App folder of your project
-
In step 3 you can see you need to configure some dependencies
- buildscript {
- dependencies {
- // Add this line
- classpath 'com.google.gms:google-services:4.2.0'
- }
- App - level build.gradle( < project > /<app-module>/build.gradle): means build.gradle file in Android = > App folder
- // Add to the bottom of the file
- apply plugin: 'com.google.gms.google-services’
Don’t need to add implementation 'com.google.firebase:firebase-core:16.0.9' in dependencies
-
In Step 4 It will try to verify your app. For that, you need to run your app once or you can skip this step.
-
Hooray :) Your Android app has been created.
-
Now, you need to enable the Email/Password and/or Google Sign In method in Firebase. For that, you need to go to the Authentication tab and then the "Sign in" method tab. From there, enable Email/Password and Google sign-in method. Please check the screenshot.
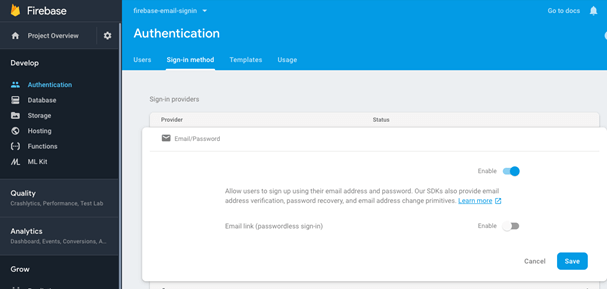
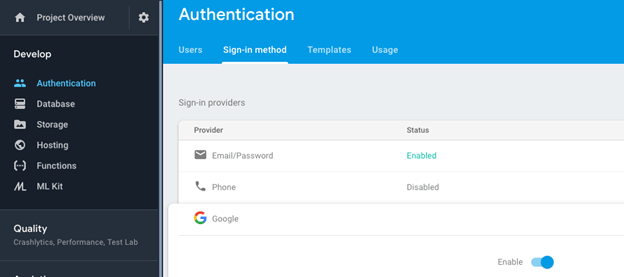
-
You are all done with firebase Setup. Congratulations…. :)
-
Go back to the project and open pubspec.yaml file in the root of the project. Pubspec.yaml is used to define all the dependencies and assets of the project.
-
Add the below dependencies and save the file
firebase_auth:google_sign_in:
-
Please check the below screenshot and you will get a better idea of where to add the dependency.
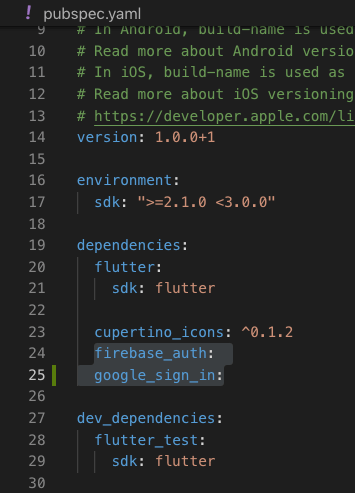
-
Run Flutter packages using get in the terminal OR If you are using Visual Studio Code then after saving file it will automatically run the Flutter package get command.
-
Now, we are done with all dependency setup at project side as well…. :)
-
Now, we need to programmatically handle signup and sign in in Google firebase. For that, we create 2 pages, login_page.dart and registration_page.dart, and signup and sign in in both pages. I have attached a link for the git repo in the bottom of the article, you can take reference from there. Here I will just define sign in and sign up methods for understanding.
import 'package:firebase_auth/firebase_auth.dart';
-
SignUp Using Email/Password in Google Firebase
- Future < FirebaseUser > signUp(email, password) async {
- try {
- FirebaseUser user = await auth.createUserWithEmailAndPassword(email: email, password: password);
- assert(user != null);
- assert(await user.getIdToken() != null);
- return user;
- } catch (e) {
- handleError(e);
- return null;
- }
- }
-
SignIn Using Email/Password in Google Firebase
- Future < FirebaseUser > signIn(String email, String password) async {
- Try {
- FirebaseUser user = await auth.signInWithEmailAndPassword(email: email, password: password);
- assert(user != null);
- assert(await user.getIdToken() != null);
- final FirebaseUser currentUser = await auth.currentUser();
- assert(user.uid == currentUser.uid);
- return user;
- } catch (e) {
- handleError(e);
- return null;
- }
- }
-
Sign In using Google
- Future < FirebaseUser > googleSignin(BuildContext context) async {
- FirebaseUser currentUser;
- try {
- final GoogleSignInAccount googleUser = await googleSignIn.signIn();
- final GoogleSignInAuthentication googleAuth = await googleUser.authentication;
- final AuthCredential credential = GoogleAuthProvider.getCredential(accessToken: googleAuth.accessToken, idToken: googleAuth.idToken, );
- final FirebaseUser user = await auth.signInWithCredential(credential);
- assert(user.email != null);
- assert(user.displayName != null);
- assert(!user.isAnonymous);
- assert(await user.getIdToken() != null);
- currentUser = await auth.currentUser();
- assert(user.uid == currentUser.uid);
- print(currentUser);
- print("User Name : ${currentUser.displayName}");
- } catch (e) {
- handleError(e);
- return currentUser;
- }
- }
-
Signout from Google
- Future < bool > googleSignout() async {
- await auth.signOut();
- await googleSignIn.signOut();
- return true;
- }
- }
-
When you sign up successfully, you can check the Google Firebase store and the user details in server. Please check the screenshot.
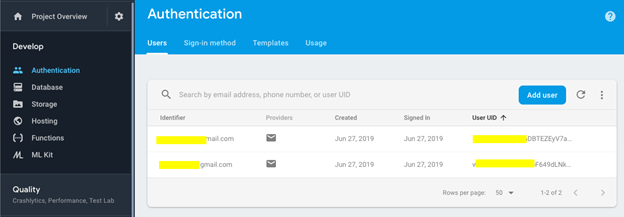
NOTE
PLEASE CHECK OUT ATTACHED ZIP FULL SOURCE CODE. YOU NEED TO ADD YOUR google-services.json FILE IN ANDROID => APP FOLDER.
PLEASE CHECK OUT ATTACHED ZIP FULL SOURCE CODE. YOU NEED TO ADD YOUR google-services.json FILE IN ANDROID => APP FOLDER.
Author
Parth Patel
270
7k
1.7m