Release APK In Flutter
Introduction
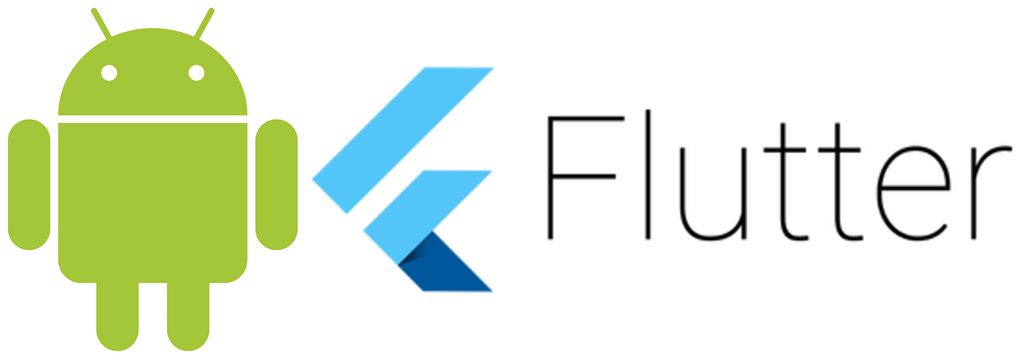
Steps
- If you are a beginner in Flutter then you can check my blog Create a first app in Flutter. I have created app named as “flutter_release_apk”
- You can specify some app related setting in build.gradle under app folder (android/app/build.gradle). I have mentioned important settings below:
- applicationId
This must be unique all around all apps in Google Play so wisely choose the name. Your app will be identified by this name and this can’t be changed. - versionCode
This is the app version code and needs to be updated each time you update the app on Google Play. Updating with the same version of code will not be accepted in Google Play Store.
For example purposes:
applicationId com.example.flutter_release_apk
versionCode 1 - Now, we need to generate signing certificate to sign android apk. Following is the command to generate a signing certificate. You need to execute this command in the terminal and this will generate flutter_release_apk.jks file in the same folder where terminal opened.
keytool -genkey -v -keystore flutter_release_apk.jks -alias alias_name -keyalg RSA -keysize 2048 -validity 10000
Enter keystore password: abcd1234
Re-enter new password: abcd1234What is your first and last name?
[Unknown]: demo demoWhat is the name of your organizational unit?
[Unknown]: demoWhat is the name of your organization?
[Unknown]: demoWhat is the name of your City or Locality?
[Unknown]: demoWhat is the name of your State or Province?
[Unknown]: demoWhat is the two-letter country code for this unit?
[Unknown]: 91Is CN=demo demo, OU=demo, O=demo, L=demo, ST=demo, C=91 correct?
[no]: yesGenerating 2,048 bit RSA key pair and self-signed certificate (SHA256withRSA) with a validity of 10,000 days
for: CN=demo demo, OU=demo, O=demo, L=demo, ST=demo, C=91Enter key password for <alias_name>
(RETURN if same as keystore password): abcd1234Re-enter new password: abcd1234
[Storing flutter_release_apk.jks]So, now I have my flutter_release_apk.jksin directory where my terminal is open.
NOTE
Copy and Save this file safely because it is required every time you need to update your app. If you lose this file then there is no way to update your app in future - Now, we need to create one file under android folder named as “key.properties”. This file stores the credentials for signing the release apk. Put the following code in this file and update as per your jsk file
storePassword=abcd1234
keyPassword=abcd1234
keyAlias=alias_name
storeFile=/Applications/AMPPS/www/Flutter_Demos/flutter_release_apk/flutter_release_apk.jks
Save the file. - Now, go back to build.gradle file under app directory (android/app/build.gradle). And now we need to define some settings for release apk generation. I have made bold some text that needs to be added or updated.
- def localProperties = new Properties()
- def localPropertiesFile = rootProject.file('local.properties')
- if (localPropertiesFile.exists()) {
- localPropertiesFile.withReader('UTF-8') {
- reader - > localProperties.load(reader)
- }
- }
- def flutterRoot = localProperties.getProperty('flutter.sdk')
- if (flutterRoot == null) {
- throw new GradleException("Flutter SDK not found. Define location with flutter.sdk in the local.properties file.")
- }
- def flutterVersionCode = localProperties.getProperty('flutter.versionCode')
- if (flutterVersionCode == null) {
- flutterVersionCode = '1'
- }
- def flutterVersionName = localProperties.getProperty('flutter.versionName')
- if (flutterVersionName == null) {
- flutterVersionName = '1.0'
- }
- apply plugin: 'com.android.application'
- apply from: "$flutterRoot/packages/flutter_tools/gradle/flutter.gradle"
- def keystoreProperiesFile = rootProject.file("key.properties")
- def keystoreProperies = new Properties()
- keystoreProperies.load(new FileInputStream(keystoreProperiesFile))
- android {
- compileSdkVersion 28
- lintOptions {
- disable 'InvalidPackage'
- }
- defaultConfig {
- applicationId "com.example.flutter_release_apk"
- minSdkVersion 16
- targetSdkVersion 28
- versionCode flutterVersionCode.toInteger()
- versionName flutterVersionName
- testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner"
- }
- signingConfigs {
- release {
- keyAlias keystoreProperies["keyAlias"]
- keyPassword keystoreProperies["keyPassword"]
- storeFile file(keystoreProperies["storeFile"])
- storePassword keystoreProperies["storePassword"]
- }
- }
- buildTypes {
- release {
- // signingConfig signingConfigs.debug
- signingConfig signingConfigs.release
- }
- }
- }
- flutter {
- source '../..'
- }
- dependencies {
- testImplementation 'junit:junit:4.12'
- androidTestImplementation 'com.android.support.test:runner:1.0.2'
- androidTestImplementation 'com.android.support.test.espresso:espresso-core:3.0.2'
- }
Save the file.
- Now, Run the command flutter build apk --release in terminal. Following is my terminal log and you can see that my release apk is generated
MyVss-Mac-mini:flutter_release_apk myvs $ flutter build apk --release
Initializing gradle... 41.4s
Resolving dependencies... 46.5s
Calling mockable JAR artifact transform to create file: /Users/myvs/.gradle/caches/transforms-1/files-1.1/android.jar/fe6e28b8b8fb0fe581763b573c73df6a/android.jar with input /Users/myvs/Library/Android/sdk/platforms/android-28/android.jar
Running Gradle task 'assembleRelease'...
Running Gradle task 'assembleRelease'... Done 117.6s
Built build/app/outputs/apk/release/app-release.apk (4.9MB).
-
Now this generated apk can be uploaded on Google Play Store as release APK.
-
Congratulations you can now upload as many apps as you need to upload on Google Play Store.
-
Best of luck, I hope you will make awesome apps in the future :)))