Push Notification Using Firebase
Introduction
In this article we are going to learn how to integrate Firebase Push Notification in Flutter applications. Firebase provides a cloud messaging service, also known as Firebase Cloud Messaging (FCM). Previously this service was known as Google Cloud Messaging (GCM). So don’t get confused with the term, basically FCM is the upgraded version of GCM.
So let’s start integrating FCM in Flutter. The plugin which we require is firebase_messaging. We need to setup a Firebase Project as well.
Prerequisite (Firebase Project Setup)
To set up the Firebase project, please check the previous part to learn about how to setup Firebase for Flutter. Please note that you need to follow only the first 2 steps.
Steps
Step 1
Follow the prerequisites and create a new Flutter project and setup a Firebase project.
Step 2
Add firebase_messaging dependency in the Flutter project. To add dependency, open pubspec.yaml, which is located at the root of the project. Check the below code snippet for more understanding.
dependencies:
flutter:
sdk: flutter
cupertino_icons: ^0.1.2
firebase_messaging: ^5.1.1
Step 3
Import Firebase messaging plugin in main.dart file.
import'package:firebase_messaging/firebase_messaging.dart';
Step 4
If you want to be notified in your app (via onResume and onLaunch, see below) when the user clicks on a notification in the system tray include the following intent-filter within the <activity> tag of your android/app/src/main/AndroidManifest.xml,
- <intent-filter>
- <action android:name="FLUTTER_NOTIFICATION_CLICK" />
- <category android:name="android.intent.category.DEFAULT" />
- </intent-filter>
Step 5
Following is the instantiation and implementation of Firebase push notification in Flutter. We have declared _firebaseMessagin object and implemented its configuration.
- final FirebaseMessaging _firebaseMessaging = FirebaseMessaging();
- final List<Notification> notifications = [];
- @override
- void initState() {
- super.initState();
- _firebaseMessaging.configure(
- onMessage: (Map<String, dynamic> notification) async {
- setState(() {
- notifications.add(
- Notification(
- title: notification["notification"]["title"],
- body: notification["notification"]["body"],
- color: Colors.red,
- ),
- );
- });
- },
- onLaunch: (Map<String, dynamic> notification) async {
- setState(() {
- notifications.add(
- Notification(
- title: notification["notification"]["title"],
- body: notification["notification"]["body"],
- color: Colors.green,
- ),
- );
- });
- },
- onResume: (Map<String, dynamic> notification) async {
- setState(() {
- notifications.add(
- Notification(
- title: notification["notification"]["title"],
- body: notification["notification"]["body"],
- color: Colors.blue,
- ),
- );
- });
- },
- );
Firebase Messaging configuration includes events like onMessage, onResume, onLaunch.
-
onMessage
If the app is open and notification is delivered then this event will be fired.
-
onResume
If app is in the background and notification is delivered and you open the app via notification tap then this event will be fired.
-
onLaunch
If app is closed and notification is delivered and you open the app via notification tap then this event will be fired.
Step 6
Request notification permission.
_firebaseMessaging.requestNotificationPermissions();
Step 7
Send Firebase push notification from Firebase project. Go to Firebase project and click on Cloud Messaging option in left menu under Grow Option. You can create your first Notification from there.
NOTE
You need to pass click_action and FLUTTER_NOTIFICATION_CLICK in additional option custom data.
You need to pass click_action and FLUTTER_NOTIFICATION_CLICK in additional option custom data.
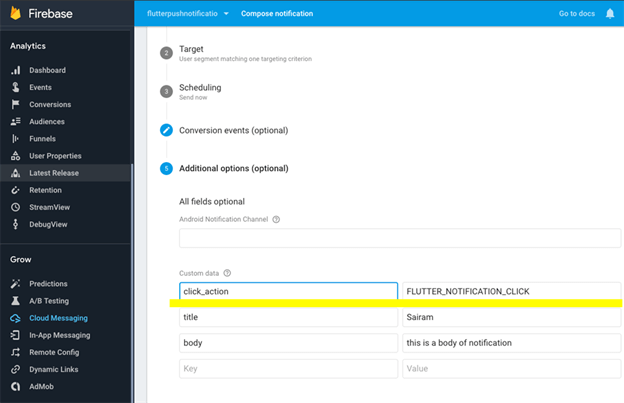
Try sending notification while: 1. Your app is pen; 2. Your app is in the background; and 3. Your app is closed. That’s all, you are finished with the Firebase push notification.
I have attached the full source code and git repository for this article.
Conclusion
In this article we have learned how to integrate firebase push notifications in Flutter apps and broadcast push notifications from Firebase. You can also broadcast push notifications programmatically from the server side.
Author
Parth Patel
267
7k
1.7m